How to Get Cursor Position in JavaScript
-
Use
selectionStart
andselectionEnd
Property to Get Cursor Position -
Use
screenX/Y
Properties to Get Cursor Position -
Use
clientX/Y
to Get Cursor Position -
Use
pageX/Y
Property to Get Cursor Positional Value
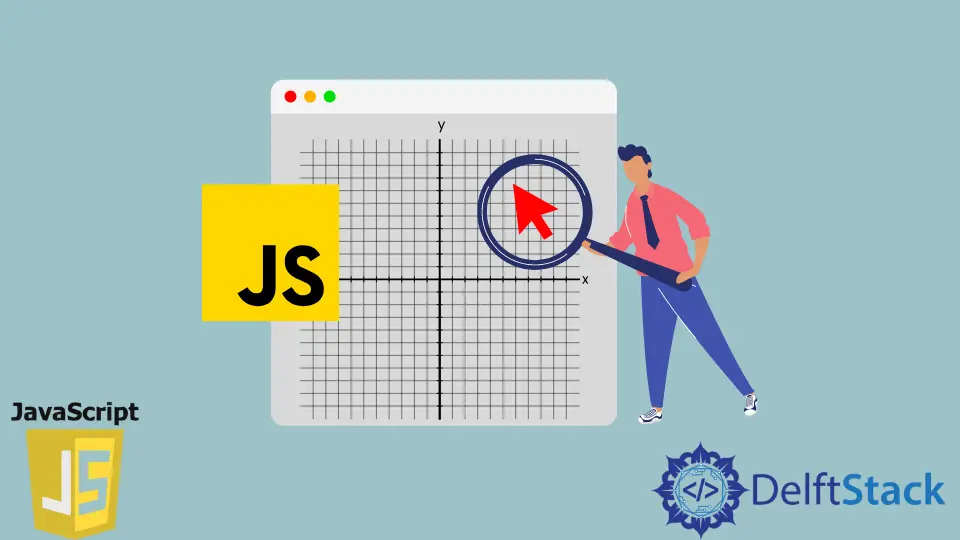
JavaScript was incorporated with the fundamental positional property selectionStart
and selectionEnd
, where we can only retrieve the positional value from a string literal’s 0th
index. Other properties also play a vital role in extracting the positional values based on coordinate marks.
In this case, the reference point can vary and thus results in different values respective to different frames. The following section will demonstrate examples of the selectionStart
and selectionEnd
properties.
We will also consider the working principle for the screenX/Y
, clientX/Y
, and pageX/Y
, where three properties are based on coordinate systems. But, first, let’s jump into the main part!
Use selectionStart
and selectionEnd
Property to Get Cursor Position
The selectionStart
and selectionEnd
properties are read/write properties. You can either define them with a positional value or take the default value, which performs the read operation.
There is also the getSelectionRange
property that explicitly takes the selectionStart
and selectionEnd
values and figures out a specific range of sub-string. Playing with strings is limited as they only work on some particular attributes.
For example, input:text
, input:textarea
, input:password
, and etc. You can have a more detailed aspect in this particular documentation. In the instance demonstrated here, we will only showcase the read-only method for the selectionStart
.
When talking about the read-only convention, the selectionStart
and selectionEnd
will perform similarly. It prints the position of the string character that the cursor has passed.
To be precise, the cursor is the text
type cursor on which these properties work. And so, we only get the position of the horizontal part, and the calculation is dependent on the character counts. So let’s check how it works.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Get Cursor</title>
</head>
<body>
<input id="Javascript_example" name="one" type="text" value="Javascript example" onmousemove="textbox()">
<p id="vals"></p>
<script>
function textbox(){
var ctl = document.getElementById('Javascript_example');
var startPos = ctl.selectionStart;
document.getElementById('vals').innerText = startPos;
}
</script>
</body>
</html>
Output:
The selectionEnd
would perform similarly here, so we didn’t add any preview. You can try and see the same outcome as the selectionStart
property. It is the read-only section of these properties.
Use screenX/Y
Properties to Get Cursor Position
In the case of the screenX/Y
property, we calculate the position referred by a reference point. The coordinate value strictly follows a certain referral point to show the position.
Here, the cursor position is for the default
cursor; thus, we will use the onmousemove
attribute to track the positions. The screenX/Y
is a read-only property, and it follows the reference point as the global or the whole screen coordinate. Let’s check the example below.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<title>Get Cursor</title>
</head>
<body>
<p>Move your mouse to see its position.</p>
<p id="screen-log" style="height:900px;width:600px; text-align:center"></p>
</body>
<script>
let screenLog = document.querySelector('#screen-log');
document.addEventListener('mousemove', logKey);
function logKey(e) {
screenLog.innerText = `
Screen X/Y: ${e.screenX}, ${e.screenY}`;
}
</script>
</html>
Output:
As can be seen, the coordinate values are updated on the cursor’s moving position. Also, in one section, you will notice that scrolling does not affect the cursor’s position.
It means that whenever a page is scrolled up-down or left-right, the whole page gets frozen and calculates the position for that instance of the frame considering the screen size.
Use clientX/Y
to Get Cursor Position
The clientX/Y
is also a read-only property with the reference point as the viewport. In this regard, the scrolled up-down viewport is kept statue, and the coordinate values are measured.
More clearly, if you are in the top section of your page, then the y
value would be 0
. And supposedly, you have scrolled down to 20
pixels, and if you try to retrieve the y
value, it will still show it as 0
.
It means whatever portion is occupying the viewport, only that is taken under consideration.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<title>Get Cursor</title>
</head>
<body>
<p>Move your mouse to see its position.</p>
<p id="screen-log" style="height:900px;width:600px; text-align:center"></p>
</body>
<script>
let screenLog = document.querySelector('#screen-log');
document.addEventListener('mousemove', logKey);
function logKey(e) {
screenLog.innerText = `
Client X/Y: ${e.clientX}, ${e.clientY}`;
}
</script>
</html>
Output:
So, in the portion where we checked the page’s top value(y)
, it resulted in 0
. And when scrolled down a little, the value remained 0
. So, we can do a similar task for the x-axis value.
Use pageX/Y
Property to Get Cursor Positional Value
Similar to screenX/Y
and clientX/Y
, pageX/Y
is also a read-only property. But unlike those properties,pageX/Y
considers the whole page’s dimension while calculating values.
It means if the viewport has a larger length than the viewport that is screened on, the property will count for the whole dimension of the entire page. Thus we can fetch important sections or cursor positions more intuitively through this property.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<title>Get Cursor</title>
</head>
<body>
<p>Move your mouse to see its position.</p>
<p id="screen-log" style="height:900px;width:600px; text-align:center"></p>
</body>
<script>
let screenLog = document.querySelector('#screen-log');
document.addEventListener('mousemove', logKey);
function logKey(e) {
screenLog.innerText = `Page X/Y: ${e.pageX}, ${e.pageY}`;
}
</script>
</html>
Output:
As can be visualized, when we have not scrolled the scrollbar, the top position value of the y-axis was 0
.
And when we scrolled down a bit, the top showed the y
value as the number of pixels that have been scrolled down to, which is 58
. So now, we can go for a comparative discussion on which property is more suitable depending on the work purpose.