Bubble Sort in JavaScript
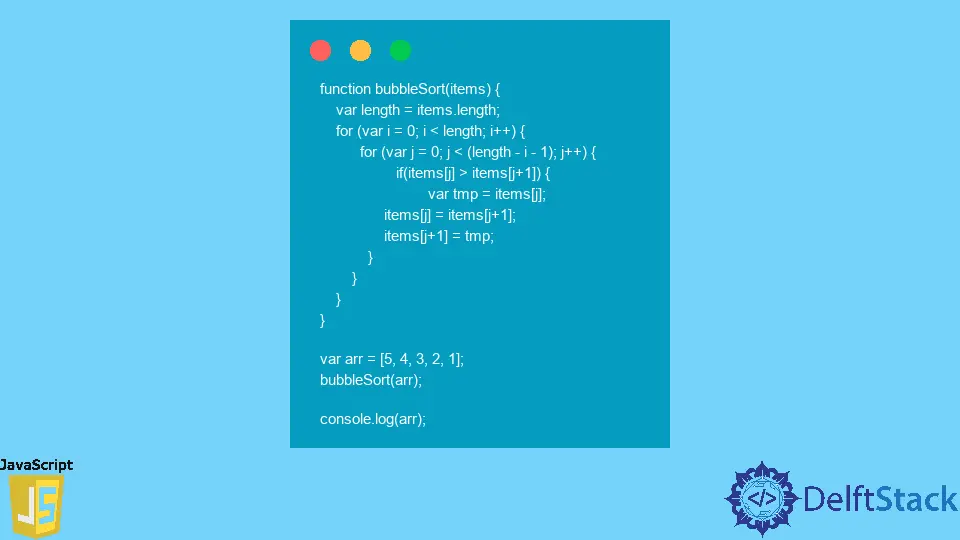
Sorting algorithms are fundamental in programming, and one of the simplest and most intuitive methods is Bubble Sort. If you’re looking to sort arrays using Bubble Sort in JavaScript, you’ve come to the right place.
In this tutorial, we’ll explore what Bubble Sort is, how it works, and provide you with clear, practical examples. Whether you’re a beginner or someone looking to brush up on your skills, this guide will help you understand the mechanics of Bubble Sort and its implementation in JavaScript. Let’s dive in!
What is Bubble Sort?
Bubble Sort is a straightforward sorting algorithm that repeatedly steps through a list, compares adjacent elements, and swaps them if they are in the wrong order. This process is repeated until the list is sorted. While it’s not the most efficient algorithm for large datasets, its simplicity makes it a great choice for educational purposes.
The name “Bubble Sort” comes from the way larger elements “bubble” to the top of the list. The algorithm has a time complexity of O(n^2), making it less suitable for large datasets compared to more advanced algorithms like Quick Sort or Merge Sort. However, its ease of implementation makes Bubble Sort a popular choice for beginners.
Implementing Bubble Sort in JavaScript
Let’s jump into the code! Below is a simple implementation of the Bubble Sort algorithm in JavaScript.
function bubbleSort(arr) {
let n = arr.length;
for (let i = 0; i < n - 1; i++) {
for (let j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
let temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
return arr;
}
const unsortedArray = [64, 34, 25, 12, 22, 11, 90];
const sortedArray = bubbleSort(unsortedArray);
console.log(sortedArray);
Output:
[11, 12, 22, 25, 34, 64, 90]
In this code, we define a function called bubbleSort
that takes an array as an argument. The outer loop runs through the entire array, while the inner loop performs the comparisons and swaps. If the current element is greater than the next element, we swap them. This process continues until the array is sorted. The final sorted array is returned.
Optimizing Bubble Sort
While the basic implementation of Bubble Sort works well, we can optimize it to improve performance. One way to do this is by introducing a flag that tracks whether any swaps were made during a complete pass through the array. If no swaps occurred, the array is already sorted, and we can break out of the loop early.
function optimizedBubbleSort(arr) {
let n = arr.length;
let swapped;
do {
swapped = false;
for (let i = 0; i < n - 1; i++) {
if (arr[i] > arr[i + 1]) {
let temp = arr[i];
arr[i] = arr[i + 1];
arr[i + 1] = temp;
swapped = true;
}
}
n--; // Reduce the range of the next pass
} while (swapped);
return arr;
}
const unsortedArray = [64, 34, 25, 12, 22, 11, 90];
const sortedArray = optimizedBubbleSort(unsortedArray);
console.log(sortedArray);
Output:
[11, 12, 22, 25, 34, 64, 90]
In this optimized version, we use a do...while
loop to allow for at least one pass through the array. The swapped
variable keeps track of whether any swaps were made. If no swaps occur during a pass, we can conclude that the array is sorted and exit the loop early. This can significantly reduce the number of comparisons in nearly sorted arrays.
Conclusion
Bubble Sort in JavaScript is a great starting point for understanding sorting algorithms. While it may not be the most efficient for large datasets, its simplicity and ease of understanding make it an excellent choice for beginners. By implementing both the basic and optimized versions of Bubble Sort, you can see the differences in performance and gain a deeper understanding of how sorting works. Whether you’re coding for fun or preparing for technical interviews, mastering Bubble Sort is a valuable skill.
FAQ
-
What is Bubble Sort?
Bubble Sort is a simple sorting algorithm that repeatedly compares adjacent elements in a list and swaps them if they are in the wrong order, continuing this process until the list is sorted. -
Why is it called Bubble Sort?
The algorithm is named Bubble Sort because larger elements “bubble” to the top of the list with each iteration. -
What is the time complexity of Bubble Sort?
The time complexity of Bubble Sort is O(n^2), making it inefficient for large datasets compared to more advanced sorting algorithms. -
Can Bubble Sort be optimized?
Yes, Bubble Sort can be optimized by introducing a flag to track whether any swaps were made during a complete pass through the array. If no swaps occur, the algorithm can terminate early. -
Is Bubble Sort used in real applications?
Due to its inefficiency, Bubble Sort is rarely used in real applications. However, it is commonly taught in computer science courses to illustrate basic sorting concepts.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn