Bubble Sort em JavaScript
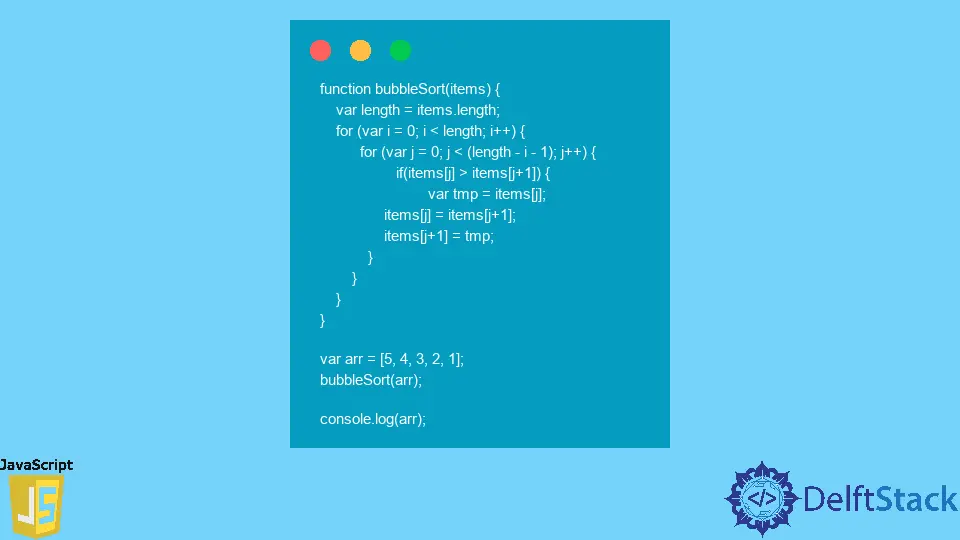
Este tutorial ensina como classificar arrays usando a classificação por bolha em JavaScript.
A classificação por bolha é um algoritmo de classificação simples. Ele funciona por comparação repetida de elementos adjacentes e trocando-os se estiverem na ordem errada. As comparações repetidas borbulham o menor / maior elemento no final do array e, portanto, esse algoritmo é denominado bubble sort. Embora ineficiente, ainda representa a base para algoritmos de classificação.
Implementação de classificação em bolha de JavaScript
function bubbleSort(items) {
var length = items.length;
for (var i = 0; i < length; i++) {
for (var j = 0; j < (length - i - 1); j++) {
if (items[j] > items[j + 1]) {
var tmp = items[j];
items[j] = items[j + 1];
items[j + 1] = tmp;
}
}
}
}
var arr = [5, 4, 3, 2, 1];
bubbleSort(arr);
console.log(arr);
Resultado:
[1, 2, 3, 4, 5]
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn