How to Make Ajax Call Without jQuery
-
How to Make Ajax Call With
XMLHttpRequest
in JavaScript -
How to Make Ajax Call With
Fetch
API in JavaScript
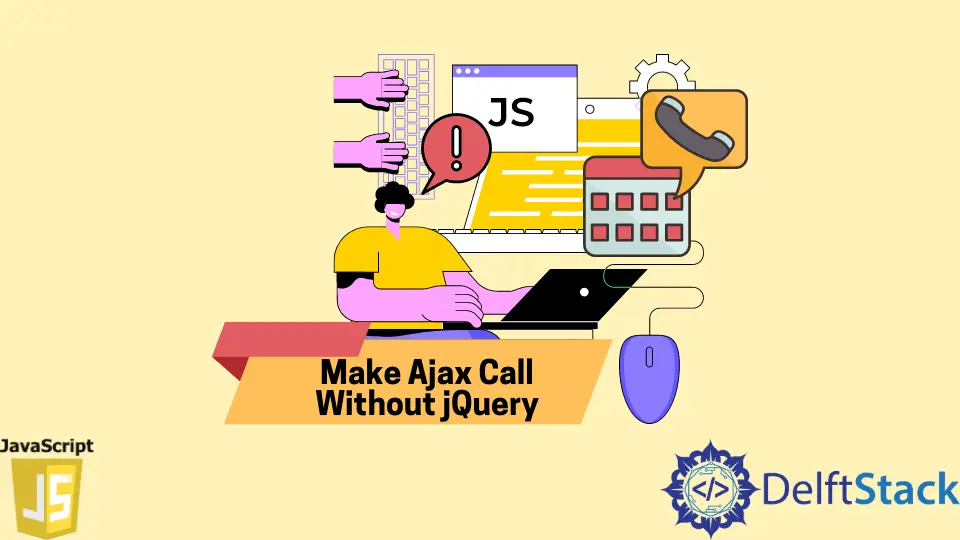
This tutorial will teach you how to make an AJAX call in JavaScript without the need for an external library. Therefore, we’ll teach you the following.
- How to make an AJAX call with
XMLHttpRequest
- How to make an AJAX call with the
Fetch
API
How to Make Ajax Call With XMLHttpRequest
in JavaScript
The XMLHttpRequest
is an object in JavaScript that allows you to make an AJAX call. It makes this possible thanks to its suite of methods.
These methods include:
XMLHTTPRequest.onreadystatechange()
XMLHTTPRequest.readyState
XMLHTTPRequest.status()
XMLHTTPRequest.open()
XMLHTTPRequest.send()
If combined correctly, these methods will allow you to make an AJAX call. Also, the object names give you an idea of what they do.
For example, XMLHTTPRequest.send()
will send the AJAX call when you are ready. The following is a typical algorithm for using XMLHttpRequest
for an AJAX call.
-
Create a new
XMLHTTPRequest
object -
Attach a function to
XMLHTTPRequest.onreadystatechange()
2.1 The function will check if
XMLHTTPRequest.readyState
returnsDONE
2.2 CheckXMLHTTPRequest.status()
and act accordingly -
Open the AJAX connection
3.1 This is the part where you specify if the connection will be a
GET
orPOST
request. -
Send the AJAX call
Meanwhile, depending on what you are trying to achieve, you might have a longer algorithm. However, for our example detailed next, the algorithm serves our purpose.
The user can initiate an AJAX call by clicking on the HTML button in the example. The call will replace the content of the <main>
element.
<head>
<meta charset="utf-8">
<title>Plain AJAX Request</title>
<style>
body {
display: grid;
justify-content: center;
align-items: center;
height: 100vh;
}
button {
padding: 1.2em;
background-color: #1a1a1a;
color: #ffffff;
border: none;
cursor: pointer;
}
</style>
</head>
<body>
<main id="button_container">
<button type="button" onclick="load_ajax_button()">CLICK ME</button>
</main>
<script>
function load_ajax_button() {
const xhttp = new XMLHttpRequest();
xhttp.onload = function() {
let button_container = document.getElementById("button_container")
button_container.innerHTML = this.responseText;
}
xhttp.open("GET", "ajax-button.html", true);
xhttp.send();
}
</script>
</body>
Output:
How to Make Ajax Call With Fetch
API in JavaScript
The Fetch
API is a built-in feature of modern web browsers that allows you to make AJAX calls. What’s more, you can consider the Fetch
API as a modern version of XMLHttpRequest
.
For example, in our code below, every time you press the HTML
button, we fetch a random image from the Picsum
API. Meanwhile, the following is how the process works.
- Click the
HTML
button to initiate theFetch
request to thePicsum
API. - We grab the
url
property from thePicsum
API response. That’s because theurl
contains the address of the random image. - Use the address from the
Picsum
API as the image source. - Therefore, every time you click the
HTML
button, you get a new random image.
<head>
<meta charset="utf-8">
<title>Fetch API</title>
<style>
main {
text-align: center;
margin: 0 auto;
max-width: 800px;
}
img {
max-width: 100%;
display: inline-block;
}
button {
margin-top: 20px;
}
</style>
</head>
<body>
<main>
<h2>A Simple Fetch API Example</h2>
<div class="img-container">
<img src="" id="random_image" width="300" height="300">
</div>
<button>Click Me for a Random Image</button>
</main>
<script>
let button = document.querySelector('button');
button.addEventListener('click', function () {
fetch('https://picsum.photos/200/300')
.then((response) => {
return response;
})
.then((image_source) => {
random_image.src = image_source['url'];
});
}, false);
</script>
</body>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn