How to Get Element by Type in JavaScript
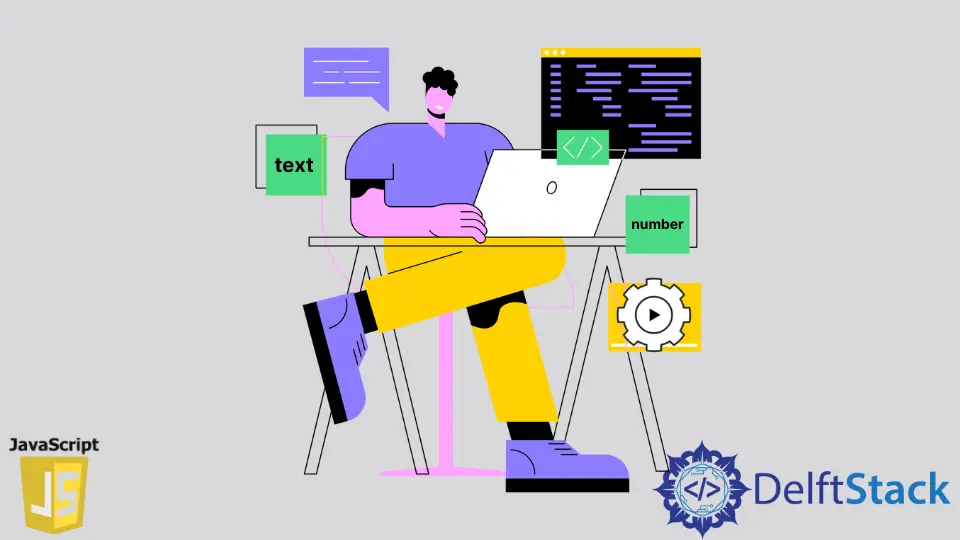
JavaScript provides various ways to get an element like getElementById()
and getElementByClass()
. But what if you want to select/process all the elements of specific type? JavaScript provides querySelectorAll()
method or getElementsByTagName()
method to perform such a task.
In this article, we are going to learn how to get elements by their type in JavaScript.
Get Element by querySelectorAll()
in JavaScript
This is a built-in document
method that is provided by JavaScript and returns the element objects/NodeList whose selectors
match the specified selectors. Multiple selectors can also be passed. There is one difference between querySelectorAll()
and querySelector()
. With the first one, all objects of the matching element are returned, and then with the second one, it’s a single matching element object. If an invalid selector is passed, a SyntaxError
is issued.
Syntax
document.querySelectorAll($selectors);
Paramater
$selectors
: It is a mandatory parameter that specifies theselector
of an HTML attribute that must match. Multiple selectors can be passed by comma separation. For eg,document.querySelectorAll("p")
find all thep
tag elements.
Return Value
Returns the corresponding DOM element objects if the corresponding elements are found; otherwise, it returns empty NodeList.
Example code:
<div>
<label>FirstName</label>
<input type="text" id="firstName" value="John">
</div>
<div>
<label>Last Name</label>
<input type="text" id="lastName" value="Doe">
</div>
const selectors = document.querySelectorAll('input[type=text]');
console.log(selectors.length, selectors[0].value, selectors[1].value);
Output:
2
John
Doe
Get Element by getElementsByTagName()
in JavaScript
This is a built-in document
method provided by JavaScript and returns the element objects/NodeList whose tag
matches the specified tag name. The root node is also included in the search, and live HTML collections are returned.
Syntax
document.getElementsByTagName($name);
Paramater
$name
: It is a mandatory parameter that specifies thetagName
of an HTML attribute that must match.
Return Value
Returns the corresponding DOM element object if the corresponding elements are found; otherwise, it returns null
.
The sole difference between getElementsByTagName
and querySelectorAll
is that the first one only selects elements whose specified tag name matches with the given tag name. In contrast, querySelectorAll
can use any selector, which gives it much more flexibility and power.
Also, getElementsByTagName
returns live node list while querySelectorAll
returns static node list. Live node list helps to update the result as soon as DOM changes, and it does not require you to fire the query.
Example code:
<div>
<label>FirstName</label>
<input type="text" id="firstName" value="John">
</div>
<div>
<label>Last Name</label>
<input type="text" id="lastName" value="Doe">
</div>
const inputs = document.getElementsByTagName('input');
for (let i = 0; i < inputs.length; i++) {
if (inputs[i].type.toLowerCase() == 'text') {
console.log(inputs[i].value);
}
}
Output:
John
Doe
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn