Enumerator in JavaScript
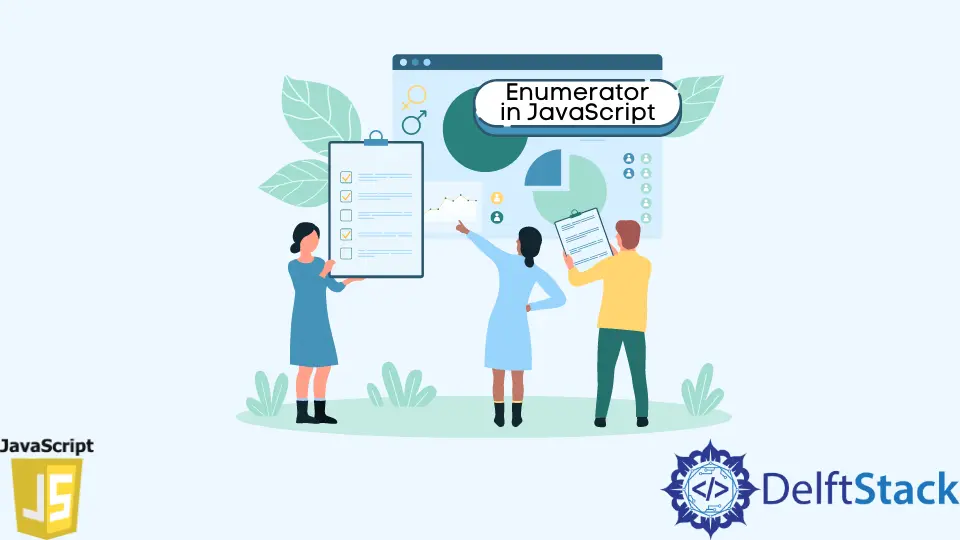
Enumeration is one of the most popular custom data types in the C language that allows the naming of integer constants, making a program easy to read and maintain. Native JavaScript does not have the traditional Enum data type, but it is introduced in TypeScript.
In today’s post, we will get to know enumerators in native JavaScript.
Create Enumerator Using Array
in JavaScript
JavaScript Arrays are special objects with predefined properties. These are the numerical properties that can be assigned to the values passed as input parameters.
JavaScript offers several methods for working with arrays. Some of the most popular array methods are .push()
, .pop()
, .map()
,.reverse()
, etc.
indexOf
in JavaScript
This is a built-in method of JavaScript. This method takes an array value and returns the index/property by iterating over an entire array.
If multiple matching values are found, the first matching value index is returned. And if no value is found, -1
is returned. The search operation performed is a strict
search with the===
operator.
Syntax:
indexOf(searchElement)
indexOf(searchElement, fromIndex)
This method runs through the array to find the searchElement
passed as input. We start the search from a certain index, and we pass the fromIndex
.
This fromIndex
skips the elements before the specified index and starts the search from this index. If this index is outside the array range, -1
is returned, which means the value cannot be searched for.
For more information, read more about the indexOf
method.
const osConfig = ['Linux', 'MacOS', 'Windows', 'Ubuntu'];
console.log(osConfig.indexOf('Linux'));
In the example code above, we defined four values of an OS array. When you pass indexOf("Linux")
, it will iterate the osConfig
, all values in the osConfig
are checked.
The output of the code above will look like the one shown below.
Output:
0
Create an Enumerator With the for
Loop in JavaScript
Another way to create the enum is with the for
loop.
Users can create the function that takes the input arguments and iterate over those arguments using the for
loop. Each argument is then used to create the object with the key as the argument and the value as the index or iteration number.
function Enum() {
for (let i = 0; i < arguments.length; ++i) {
this[arguments[i]] = i;
}
return this;
}
const config = {};
config.type = new Enum('Linux', 'MacOS', 'Windows', 'Ubuntu');
console.log(config);
console.log(config.type.Linux);
In the example above, we created the Enum
function that takes the arguments and iterates the arguments one by one, creating the local object. When you run the code above, you will get the following output.
Output:
{ type: Enum { Linux: 0, MacOS: 1, Windows: 2, Ubuntu: 3 } }
0
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn