Const Function Expression in JavaScript
- What Is a Function Declaration in JavaScript
- What Is a Function Expression in JavaScript
-
Use the
const
Function Expression in JavaScript
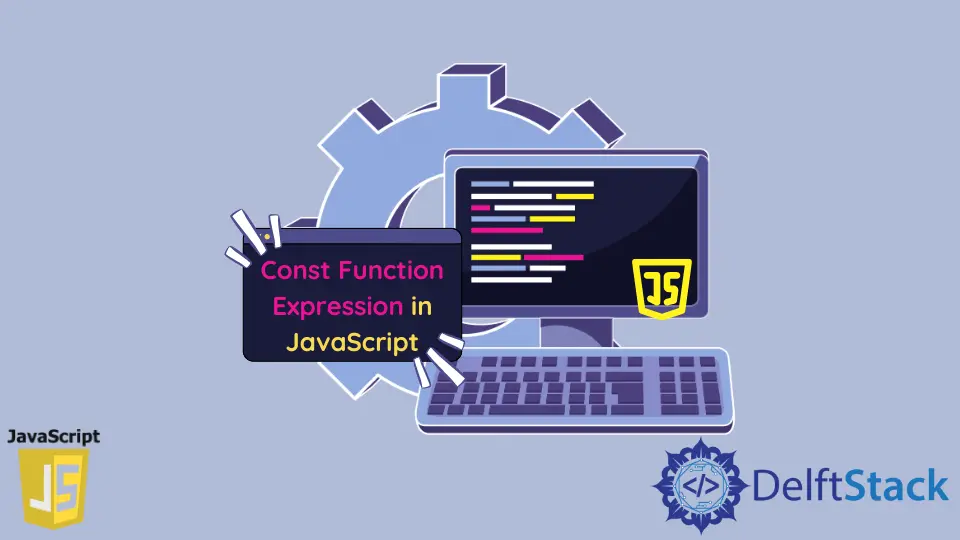
The Function statement declares a function that will only execute when called. This article will discuss the different use of constant function expressions in JavaScript.
What Is a Function Declaration in JavaScript
Function Declaration means defining a function with a name
and parameters
. Let’s create a function with the name sum
, which will take two arguments,
function sum(a, b) {
return a + b;
}
The declared functions are hoisted to the global scope or top of the enclosing block. Due to this, we can call the function before the declaration.
console.log('sum(1,2) :', sum(1, 2));
function sum(a, b) {
return a + b;
}
Output:
sum(1,2) :3
In the above code, the function sum
will be available before executing the first line of the code.
What Is a Function Expression in JavaScript
Function Expression is very similar to function declaration. The only differences are:
- Assign the function to a variable and execute the function by using the variable name.
var sumFn1 = function sum(a, b) {
return a + b;
};
console.log('sumFn1(1,3) :', sumFn1(1, 3));
Output:
sumFn1(1,3) :4
- Function name may be omitted.
var sumFn2 = function(a, b) {
return a + b;
};
console.log('sumFn2(1,3) :', sumFn2(1, 3));
Output:
sumFn2(1,3) :4
- Can be used to create a self-invoking function (Immediately Invoking Function Expression), executed as soon as the function is defined.
(function(a, b) {
return a + b;
})(3, 4); // 7
Output:
7
- Function expressions are not hoisted until the function is declared, so we cannot call the function until the function expression is evaluated.
sumFn3(1, 3); // TypeError: sumFn3 is not a function
var sumFn3 = function sum(a, b) {
return a + b;
};
Output:
TypeError: sumFn3 is not a function
- We can use the arrow function expression and assign it to a variable. It will make the code shorter and more understandable.
var sumFn4 = (a, b) => a + b;
console.log('sumFn4(10,20) :', sumFn4(10, 20));
Output:
sumFn4(10,20) :30
Use the const
Function Expression in JavaScript
The keyword const
was introduced in JavaScript ES6.
Assigning a function expression to a const
variable ensures that the function definition is unchanged because you cannot change the const
variable.
var changableSumFn = function(a, b) {
return a + b;
} changableSumFn(2, 3); // 5
// Function definition of a non-constant variable can be changed
changableSumFn = function(a, b) {
return a * b;
} changableSumFn(2, 3); // 6
// using const function expression
const constSumFn = function(a, b) {
return a + b;
} constSumFn(1, 3); // 4
// when we try to chanage const value we will get error
constSumFn = function(a, b) { // TypeError:Assignment to constant variable.
return a * b;
}
Output:
5
6
4
TypeError: Assignment to constant variable.
In the above code,
- Create a function expression to calculate the sum of two values and assign it to a non-constant variable
changableSumFn
. - Assigned a new function expression (which will calculate the product of the two values) to the variable
changableSumFn
. The product of two values is returned when we executechangableSumFn
. - Then, we created another function expression and assigned it to a
const
variableconstSumFn
. When we assign a new function expression to theconstSumFn
variable, we will getTypeError
.