How to Detect Browser Version in JavaScript
-
Use the
userAgent
to Detect Browser Version in JavaScript - Why Browser Version Detection Should Be Avoided in JavaScript
- Another Option to Detect Browser Version in JavaScript
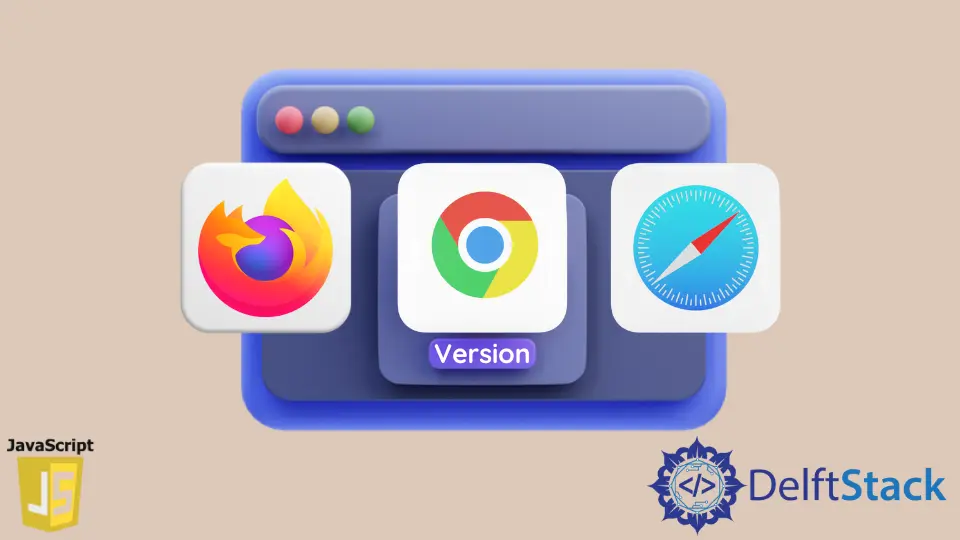
There are many devices in today’s world with various screen sizes.
But the problem is that not all the devices can support various features implemented on the website.
To detect the browser version and browser name, we can use the userAgent
in JavaScript.
Use the userAgent
to Detect Browser Version in JavaScript
The navigator is the property of the window object.
To access the userAgent
, you can use navigator.userAgent
or use object destructuring to get the userAgent
from the navigator.
const {userAgent} = navigator
console.log(userAgent);
Using the includes
method will take a string as a parameter to return it. This string helps in detecting the browser as follows.
if (userAgent.includes('Firefox/')) {
console.log(userAgent)
} else if (userAgent.includes('Edg/')) {
console.log(userAgent)
} else if (userAgent.includes('Chrome/')) {
console.log(userAgent)
} else if (userAgent.includes('Safari/')) {
// Safari
}
Output:
//Mozilla Firefox
Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:98.0) Gecko/20100101 Firefox/98.0
//Microsoft Edge
Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/98.0.4758.80 Safari/537.36 Edg/98.0.1108.50
// Google Chrome
Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/98.0.4758.102 Safari/537.36
The browser version and its name are present at the end of the string provided by the userAgent
.
You can run the code below if you want to get the end part, i.e., the browser version and name and not the entire string.
if (userAgent.includes('Firefox/')) {
console.log(`Firefox v${userAgent.split('Firefox/')[1]}`)
} else if (userAgent.includes('Edg/')) {
console.log(`Edg v${userAgent.split('Edg/')[1]}`)
} else if (userAgent.includes('Chrome/')) {
console.log(`Chrome v${userAgent.split('Chrome/')[1]}`)
} else if (userAgent.includes('Safari/')) {
// Safari
}
Output:
//Mozilla Firefox
Firefox v98.0
//Microsoft Edge
Edg v98.0.1108.50
// Google Chrome
Chrome v98.0.4758.102 Safari/537.36
All browser displays the same output. It is because all are built on chromium.
Why Browser Version Detection Should Be Avoided in JavaScript
It’s not a good idea to detect the browser name and its version using userAgent
because it’s not 100% accurate.
Every browser sets this data differently, and all browsers do not follow a particular standard.
Another Option to Detect Browser Version in JavaScript
Feature detection in a browser-
It can be a better idea to detect whether a particular browser supports a particular feature or not. And based on whether it supports a feature or not, you can take further action and write your code accordingly.
Progressively developing a website-
Following a design technique, you develop a website for smaller devices first with fewer features and move your way up to the top while increasing the features. It is known as a bottom-up approach.
Building for modern browsers-
Develop a full-fledged website with all the features for modern browsers and then tweak some changes so that it is supported on older browsers. It can be difficult to implement and less effective than the progressive or bottom-up approach.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn