How to Get Browser Width in JavaScript
- Use One Line of Code in The Browser Console to Get Width in JavaScript
-
Use
inner.width
andinner.height
to Get Browser Width and Height in JavaScript
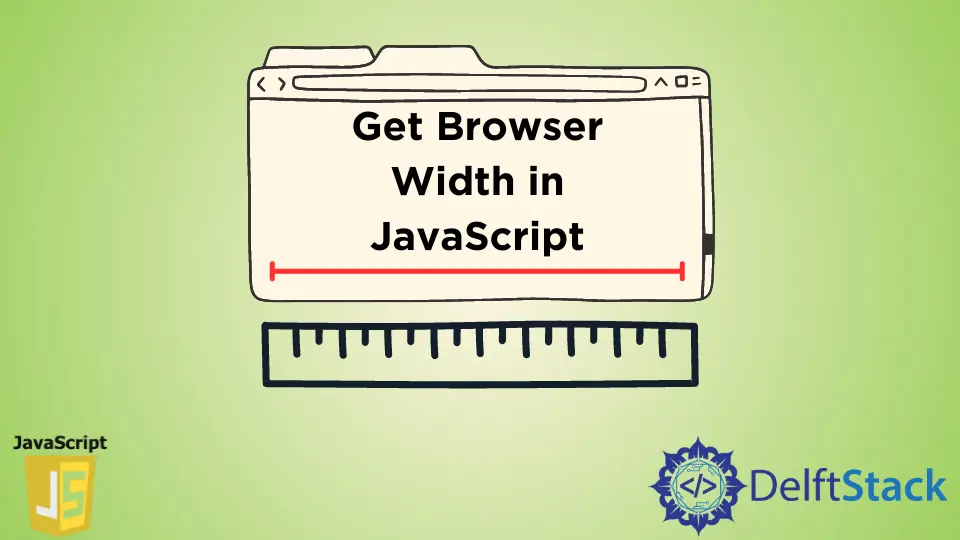
The browser width shows the actual width of the browser window in the unit of pixels. The browser width is different than the screen width.
It shows the number of pixels in the viewable browser space, and the screen width is the total size of the screen in pixels.
Use One Line of Code in The Browser Console to Get Width in JavaScript
First, open your browser console and copy-paste the following code in the browser console.
The browser’s width is always smaller or equal to the screen’s width because it doesn’t include scroll bars and borders.
'Browser width: ' + window.innerWidth +
' pixels\nScreen width: ' + screen.width + ' pixels';
Output:
'Browser width: 982 pixels\nScreen width: 1536 pixels'
Use inner.width
and inner.height
to Get Browser Width and Height in JavaScript
We will create an HTML page in which we will do scripting inside the body of the code.
In the scripting section, we will write a code that will display the inner width of the browser in the output.
When we run the HTML code, a page opens in the browser. We used the alert method in the scripting to display a message on the page.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Browser width</title>
<h1>Getting width in HTML page</h1>
</head>
<body>
<p id="demo"></p>
<script>
var w = window.innerWidth
|| document.documentElement.clientWidth
|| document.body.clientWidth;
var h = window.innerHeight
|| document.documentElement.clientHeight
|| document.body.clientHeight;
var x = document.getElementById("demo");
x.innerHTML = "Your Browser inner window width: " + w + ", height: " + h + ".";
alert("Your Browser inner window width: " + w + ", height: " + h + ".");
</script>
</body>
</html>
The display message will show the inner width and height of the browser. The width and height are default in this case.
But when we change the width of the browser. The display message will show different widths and heights of the browser.
Output:
Your Browser inner window width: 362, height: 450.
The inner.width
gives us the inner width of the browser. The inner.height
gives us the inner height of the browser.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn