varargs in Java
-
Syntax of Variable Arguments
Varargs
Ib Java -
Using the
Varargs
Arguments in Java - Overloading the Variable Arguments Method in Java
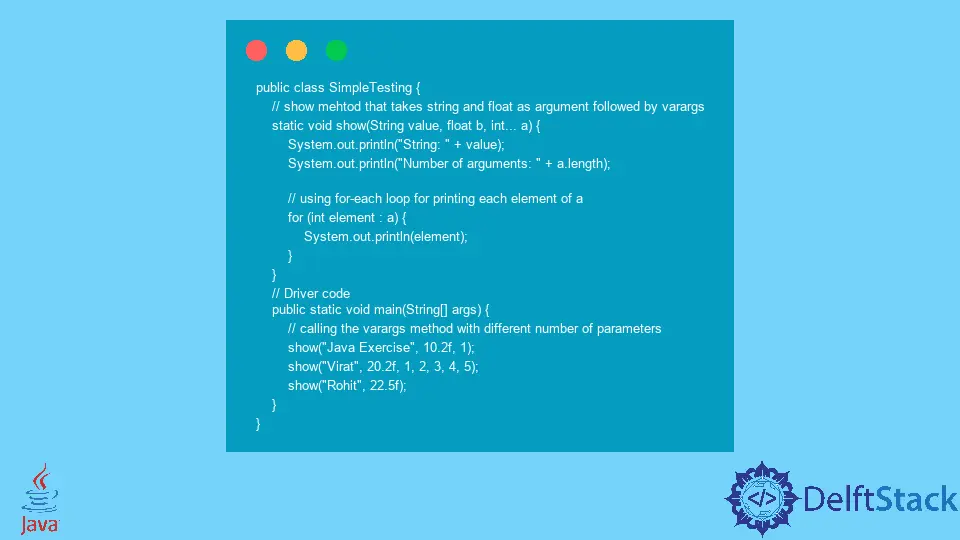
Variable arguments varargs
is a concept in Java. We can give the variable number of arguments zero or multiple arguments
to the method.
Generally, a method takes the same number of arguments mentioned in its signature, but using the varargs
, we can pass any number of arguments.
We can also use the overloaded
method, or we can also use the method that takes an array as a parameter for the same purpose, but it is not considered good because it leads to a maintenance problem.
There are reasons why we need to use varargs
in Java, which are:
- We don’t know the number of arguments to pass for a method.
- We want to pass the unlimited number of arguments to a method.
Syntax of Variable Arguments Varargs
Ib Java
public static void show(int... a) {
// body of the method
}
In varargs
, we use three dots after the data type. Let’s understand with code examples.
Using the Varargs
Arguments in Java
In this example, we created a show()
method that takes varargs
arguments, and during a call, we passed the different numbers of arguments. This method works fine for all the arguments length.
public class SimpleTesting {
// show method that takes the variable number of integers as arguments
static void show(int... a) {
System.out.println("Number of arguments: " + a.length);
// using for-each loop for printing each element of a
for (int element : a) {
System.out.println(element);
}
}
// Driver code
public static void main(String[] args) {
// one parameter
show(25);
// four parameters
show(10, 20, 30, 40);
// zero parameters
show();
}
}
Output:
Number of arguments: 1
25
Number of arguments: 4
10
20
30
40
Number of arguments: 0
We have created a method show()
that takes the variable number of integer arguments. We have declared the data type of an array as int
so it can take only integers
values.
We used the a.length()
method to determine the number of arguments. Then we have created each loop to display the content of a
.
Then, we called the varargs
method with one parameter
, four parameters
, and no parameters
. We can give the variable length of parameters to the method.
Still, we have to remember that we can give only one varargs
parameter to the method and should write that last in the parameter list of the method declaration.
For example:
static void show(String value, float b, int... a)
In this case, we must provide the first argument as a string, the second argument is a float, and then we can provide the remaining arguments as an integer.
public class SimpleTesting {
// show mehtod that takes string and float as argument followed by varargs
static void show(String value, float b, int... a) {
System.out.println("String: " + value);
System.out.println("Number of arguments: " + a.length);
// using for-each loop for printing each element of a
for (int element : a) {
System.out.println(element);
}
}
// Driver code
public static void main(String[] args) {
// calling the varargs method with different number of parameters
show("Java Exercise", 10.2f, 1);
show("Virat", 20.2f, 1, 2, 3, 4, 5);
show("Rohit", 22.5f);
}
}
Output:
String: Java Exercise
Number of arguments: 1
1
String: Virat
Number of arguments: 5
1
2
3
4
5
String: Rohit
Number of arguments: 0
Overloading the Variable Arguments Method in Java
This example demonstrates how to override a method that accepts varargs
arguments. See the example below.
class SimpleTesting {
// method overloading example takes integers as a variable arguments
private void show(int... a) {
int sum = 0;
for (int element : a) {
sum += element;
}
System.out.println("sum = " + sum);
}
// takes boolean as a argument followed by varargs
private void show(boolean p, String... args) {
boolean negate = !p;
System.out.println("negate = " + negate);
System.out.println("args.length = " + args.length);
}
// Driver code
public static void main(String[] args) {
SimpleTesting obj = new SimpleTesting();
obj.show(11, 22, 33);
obj.show(true, "virat", "Rohit");
}
}
Output:
sum = 66
negate = false
args.length = 2
The Rules for varargs
are:
- We can provide only one variable argument in the method.
- Variable argument must be the last argument.
Some example of varargs
that fails to compile:
void method(float... a, int... b) {} // Compile time error
void method(double... a, String b) {} // Compile time error
In this article, we have learned about the varargs
. We have seen why there is a need for the varargs
syntax, and we have taken three examples to understand the concept of varargs
in depth.