The ... Parameter in Java
- What are Varargs?
- How to Use Varargs in Java
- Best Practices for Using Varargs
- Common Use Cases for Varargs
- Conclusion
- FAQ
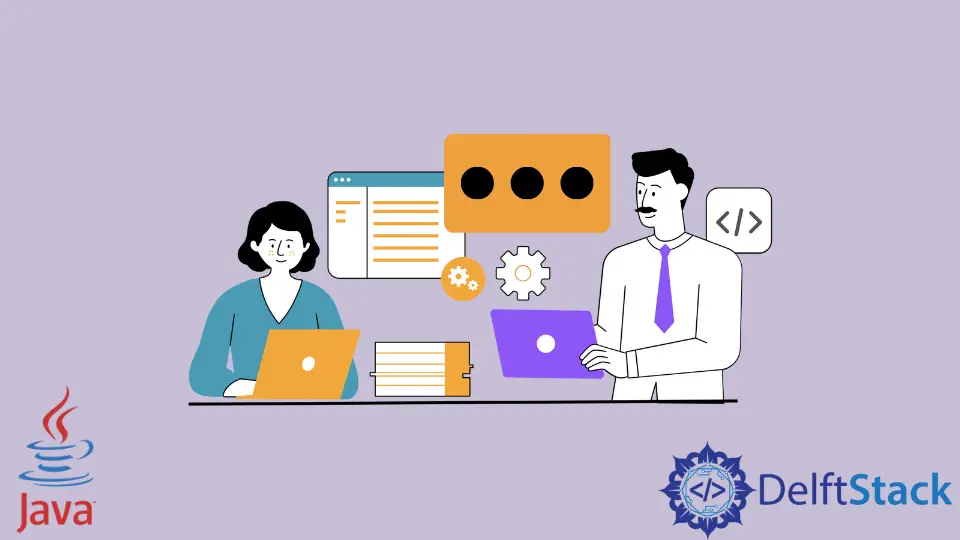
In the world of Java programming, the varargs parameter is a powerful feature that simplifies the way we handle method arguments. Short for “variable-length arguments,” varargs allows developers to pass a variable number of arguments to a method without the need for cumbersome array handling. This not only enhances code readability but also provides flexibility in method calls. Whether you’re a seasoned Java developer or just starting your journey, understanding varargs can significantly improve your coding efficiency.
In this article, we’ll explore what varargs is, how to use it effectively, and some common use cases that demonstrate its versatility. So, let’s dive into the world of varargs in Java!
What are Varargs?
Varargs, or variable-length arguments, is a feature in Java introduced in version 5. It allows you to pass zero or more arguments to a method. This is particularly useful when the number of arguments can vary. When declaring a method with varargs, you simply use an ellipsis (…) followed by the type of the arguments. For instance, if you want to create a method that takes multiple integers, you would declare it like this:
public void displayNumbers(int... numbers) {
for (int number : numbers) {
System.out.println(number);
}
}
In this example, the method displayNumbers
can accept any number of integer arguments, including none at all. The arguments passed to the method are treated as an array, making it easy to iterate through them. This feature is particularly beneficial in scenarios where the number of inputs is not fixed, such as in logging, mathematical calculations, or when handling user input.
How to Use Varargs in Java
Using varargs in Java is straightforward. To declare a method that accepts variable-length arguments, follow these steps:
- Declare the Method: Use the ellipsis (…) in the method signature.
- Call the Method: You can pass any number of arguments, or none at all.
- Access the Arguments: Inside the method, treat the varargs as an array.
Here’s an example:
public class VarargsExample {
public static void main(String[] args) {
VarargsExample example = new VarargsExample();
example.printNames("Alice", "Bob", "Charlie");
example.printNames("Dave");
example.printNames();
}
public void printNames(String... names) {
if (names.length == 0) {
System.out.println("No names provided.");
return;
}
for (String name : names) {
System.out.println(name);
}
}
}
When you run this code, you’ll see:
Alice
Bob
Charlie
Dave
No names provided.
This example demonstrates how the printNames
method can handle different numbers of string arguments. If no names are provided, it gracefully informs the user. The flexibility of varargs makes it an excellent choice for methods that require varying input sizes.
Best Practices for Using Varargs
While varargs is a powerful tool, there are best practices to keep in mind to ensure your code remains clean and efficient:
-
Use Varargs as the Last Parameter: If a method has multiple parameters, place the varargs parameter at the end of the parameter list. This is essential because varargs can only be used once in a method signature.
-
Avoid Overloading with Varargs: Be cautious when overloading methods that use varargs. It can lead to ambiguity, making it difficult for the compiler to determine which method to invoke.
-
Consider Performance: While varargs is convenient, it does involve creating an array behind the scenes. If performance is a critical concern, especially in tight loops, consider using fixed-length arrays instead.
-
Type Safety: Since varargs can accept any number of arguments, ensure that you’re passing the correct types to avoid
ClassCastException
at runtime.
By following these best practices, you can leverage the full potential of varargs while maintaining code quality and performance.
Common Use Cases for Varargs
Varargs can be particularly useful in various programming scenarios. Here are some common use cases:
-
Logging: When creating a logging method, you might want to log multiple messages at once. Using varargs allows you to pass as many messages as needed without creating an array each time.
public void logMessages(String... messages) { for (String message : messages) { System.out.println("Log: " + message); } }
-
Mathematical Operations: If you’re writing a method to compute the sum of numbers, varargs makes it easy to pass any number of integers.
public int sum(int... numbers) { int total = 0; for (int number : numbers) { total += number; } return total; }
-
Event Handling: In GUI applications, you might want to handle multiple events simultaneously. Varargs can simplify the event handling method by allowing multiple event sources.
In each of these cases, varargs enhances code readability and flexibility, making your methods more versatile and easier to maintain.
Conclusion
Understanding the varargs parameter in Java is essential for any developer looking to write cleaner and more efficient code. This feature not only simplifies method declarations but also enhances the flexibility of method calls. By leveraging varargs, you can create methods that handle a variable number of arguments seamlessly, making your code more adaptable to different scenarios. As you continue your Java journey, keep exploring the various applications of varargs, and don’t hesitate to implement these best practices in your projects.
FAQ
-
What is varargs in Java?
Varargs in Java allows a method to accept a variable number of arguments, making it easier to handle different input sizes without using arrays. -
How do I declare a varargs method?
You declare a varargs method by using an ellipsis (…) followed by the type of the arguments in the method signature. -
Can I use multiple varargs in a method?
No, you can only use one varargs parameter in a method, and it must be placed at the end of the parameter list. -
Are varargs type-safe?
Yes, varargs are type-safe, but you must ensure that you pass the correct types to avoid runtime exceptions. -
What are some best practices for using varargs?
Use varargs as the last parameter, avoid overloading methods with varargs, consider performance implications, and ensure type safety.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn