Delegation as a Substitute for Inheritance in Java
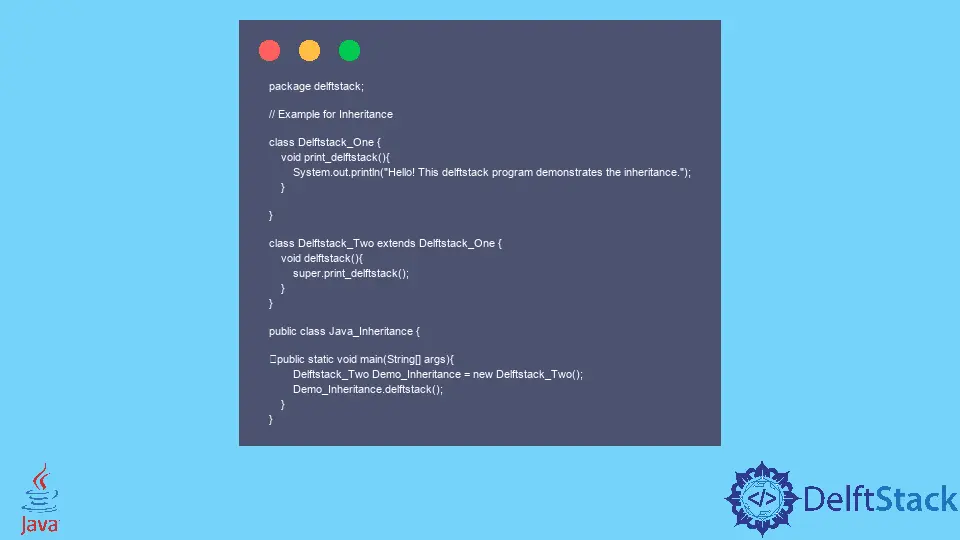
The substitute for Inheritance in Java is Delegation
. Delegation
can be defined as assigning a task or duty to a secondary party.
In Java, Delegation
is achieved using the instance of a class variable and passing messages to the instance. This tutorial demonstrates how Delegation
can be a substitute for inheritance in Java.
Use Delegation
as a Substitute for Inheritance in Java
Delegation
concentrates more on how instances are related to each other. Delegation
has several advantages over inheritance, such as Delegation
being dynamic even at the run-time, which means it can be edited at run-time.
We can achieve the following using Delegation
.
Delegation
can reduce the coupling of methods to their class.- Components can behave identically in
Delegation
, and it should also be mentioned here that this situation can be changed in the future. - If we need to use functionality in another class without changing that functionality, then
Delegation
is the better choice over inheritance. - We can use
Delegation
and composition when we have to enhancedemo
, but thedemo
is final, which cannot be further sub-classed. - With
Delegation
, we can reuse the functionality of a method without overriding it.
First, let’s see an example for inheritance in Java and convert it to Delegation
.
package delftstack;
// Example for Inheritance
class Delftstack_One {
void print_delftstack() {
System.out.println("Hello! This delftstack program demonstrates the inheritance.");
}
}
class Delftstack_Two extends Delftstack_One {
void delftstack() {
super.print_delftstack();
}
}
public class Java_Inheritance {
public static void main(String[] args) {
Delftstack_Two Demo_Inheritance = new Delftstack_Two();
Demo_Inheritance.delftstack();
}
}
The code above is an example of inheritance
, which we call a method of parent inside the child.
Output:
Hello! This delftstack program demonstrates the inheritance.
Now let’s try to achieve the same functionality using Delegation
.
package delftstack;
// Example for delegation
class Delftstack_One {
void print_delftstack() { // this method is the "delegate"
System.out.println("Hello! This delftstack program demonstrates the delegation.");
}
}
class Delftstack_Two {
Delftstack_One Demo_Delegation = new Delftstack_One(); // this is how a "delegator" is created
void delftstack() { // This method is used to create the delegate
Demo_Delegation.print_delftstack(); // This is the delegation
}
}
public class Java_Delegation {
// The delegation will work similarly to inheritance but with more dynamic properties
public static void main(String[] args) {
Delftstack_One printer = new Delftstack_One();
printer.print_delftstack();
}
}
The code above demonstrates the whole process of delegation.
Output:
Hello! This delftstack program demonstrates the delegation.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook