Delegation 作为 Java 中继承的替代品
Sheeraz Gul
2023年10月12日
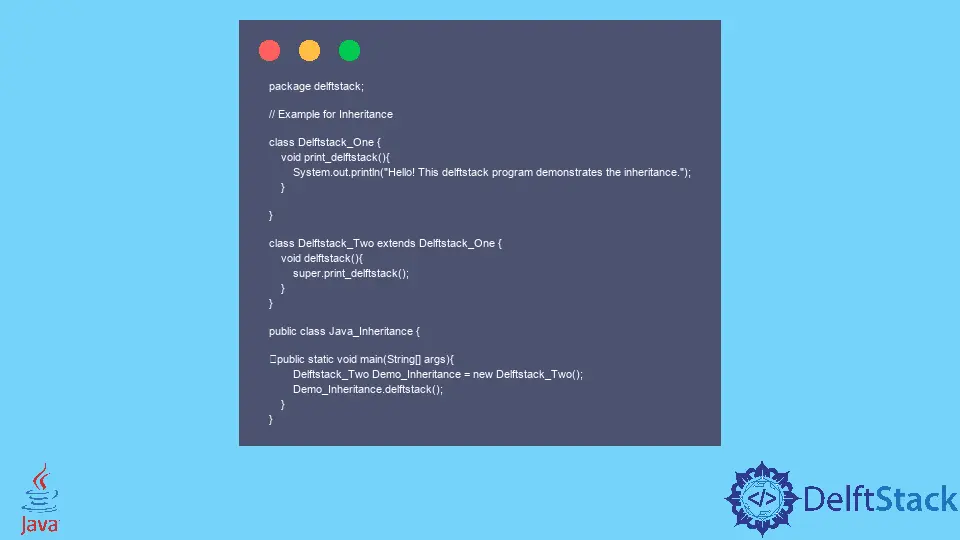
Java 中继承的替代品是 Delegation
。Delegation
可以定义为将任务或职责分配给次要方。
在 Java 中,Delegation
是使用类变量的实例并将消息传递给实例来实现的。本教程演示了 Delegation
如何可以替代 Java 中的继承。
在 Java 中使用 Delegation
作为继承的替代品
Delegation
更多地关注实例之间的关系。Delegation
与继承相比有几个优点,例如 Delegation
即使在运行时也是动态的,这意味着它可以在运行时进行编辑。
我们可以使用 Delegation
实现以下目标。
Delegation
可以减少方法与其类的耦合。- 组件在
Delegation
中的行为可以相同,这里也应该提到,这种情况将来可以改变。 - 如果我们需要在另一个类中使用功能而不改变该功能,那么
Delegation
是比继承更好的选择。 - 需要增强
demo
时可以使用Delegation
和 composition,但是demo
是 final 的,不能进一步细分。 - 使用
Delegation
,我们可以重用方法的功能而不覆盖它。
首先,让我们看一个 Java 中的继承示例并将其转换为 Delegation
。
package delftstack;
// Example for Inheritance
class Delftstack_One {
void print_delftstack() {
System.out.println("Hello! This delftstack program demonstrates the inheritance.");
}
}
class Delftstack_Two extends Delftstack_One {
void delftstack() {
super.print_delftstack();
}
}
public class Java_Inheritance {
public static void main(String[] args) {
Delftstack_Two Demo_Inheritance = new Delftstack_Two();
Demo_Inheritance.delftstack();
}
}
上面的代码是 inheritance
的一个例子,我们称其为子类中的父类方法。
见输出:
Hello! This delftstack program demonstrates the inheritance.
现在让我们尝试使用 Delegation
来实现相同的功能。
package delftstack;
// Example for delegation
class Delftstack_One {
void print_delftstack() { // this method is the "delegate"
System.out.println("Hello! This delftstack program demonstrates the delegation.");
}
}
class Delftstack_Two {
Delftstack_One Demo_Delegation = new Delftstack_One(); // this is how a "delegator" is created
void delftstack() { // This method is used to create the delegate
Demo_Delegation.print_delftstack(); // This is the delegation
}
}
public class Java_Delegation {
// The delegation will work similarly to inheritance but with more dynamic properties
public static void main(String[] args) {
Delftstack_One printer = new Delftstack_One();
printer.print_delftstack();
}
}
上面的代码演示了委托的整个过程。
输出:
Hello! This delftstack program demonstrates the delegation.
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook