Stack Pop Push in Java
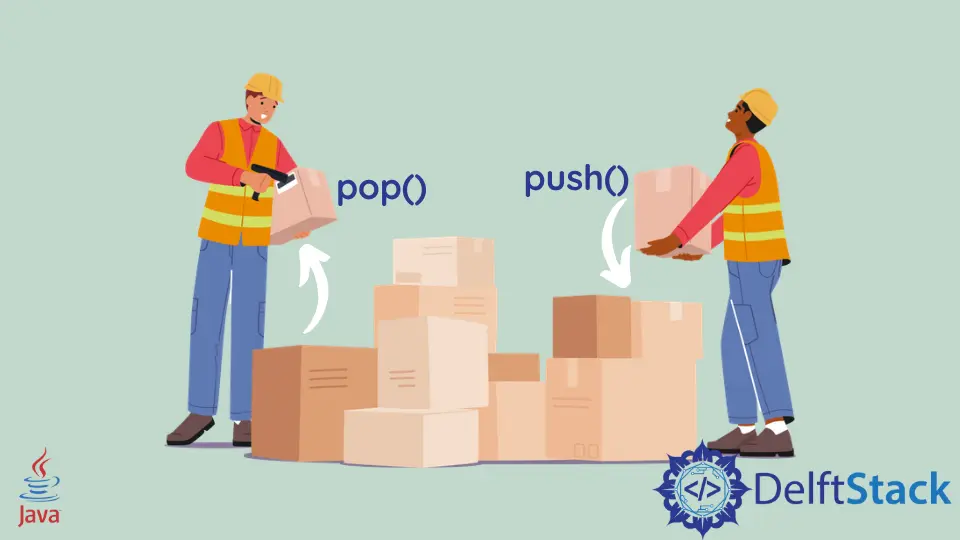
A push operation adds an element to the topmost position of the stack, while the pop operation deletes the topmost element of the stack.
We’ll go through how to use the concept of a stack with push and pop operations in the sections below.
Stack With Push Pop Using ArrayList
in Java
The following example uses an ArrayList
to implement a stack. First, we create two classes, one is the ExampleClass1
, and the other is StackPushPopExample
, in which we create the logic for push and pop operations in the stack.
- The
push()
method: takes anint
parameter type and adds it to the first position of the list we created. A stack follows the LIFO concept for Last In First Out, adding every new item at the first position and shifting the older items. - The
pop()
function: first checks if the stack is empty and, if not, proceeds to remove the element at the zeroth index of the list or the topmost element of the stack.
Example:
import java.util.ArrayList;
import java.util.List;
public class ExampleClass1 {
public static void main(String[] args) {
StackPushPopExample stackPushPopExample = new StackPushPopExample(5);
stackPushPopExample.push(2);
stackPushPopExample.push(3);
stackPushPopExample.push(4);
stackPushPopExample.push(7);
stackPushPopExample.push(1);
System.out.println("Topmost Element of the stack: " + stackPushPopExample.peek());
System.out.println("All Stack Items:");
for (Integer allItem : stackPushPopExample.getAllItems()) {
System.out.println(allItem);
}
stackPushPopExample.pop();
System.out.println("All Stack Items After popping one item:");
for (Integer allItem : stackPushPopExample.getAllItems()) {
System.out.println(allItem);
}
}
}
class StackPushPopExample {
private final List<Integer> intStack;
public StackPushPopExample(int stackSize) {
intStack = new ArrayList<>(stackSize);
}
public void push(int item) {
intStack.add(0, item);
}
public int pop() {
if (!intStack.isEmpty()) {
int item = intStack.get(0);
intStack.remove(0);
return item;
} else {
return -1;
}
}
public int peek() {
if (!intStack.isEmpty()) {
return intStack.get(0);
} else {
return -1;
}
}
public List<Integer> getAllItems() {
return intStack;
}
}
Output:
Topmost Element of the stack:: 1
All Stack Items:
1
7
4
3
2
All Stack Items After popping one item:
7
4
3
2
To see the elements in the stack, we create two functions, the peek()
method that returns the top item of the stack and the getAllItems()
that returns all the items of the stack.
Lastly, the pop()
function to delete the first element of the stack and then print the stack again to see if the element was deleted.
Stack With Push Pop Using the Stack
Class in Java
The Collections Framework in Java provides a class called Stack
that gives us methods to perform all the basic operations in a stack. In the following snippet, we’ll create an object of Stack
with a type parameter of String
.
Example:
import java.util.Stack;
public class ExampleClass1 {
public static void main(String[] args) {
Stack<String> stack = new Stack<>();
stack.push("Item 1");
stack.push("Item 2");
stack.push("Item 3");
stack.push("Item 4");
stack.push("Item 5");
System.out.println("Topmost Element of the stack: " + stack.peek());
stack.pop();
System.out.println("After popping one item:");
System.out.println("Topmost Element of the stack: " + stack.peek());
}
}
Output:
Topmost Element of the stack: Item 5
After popping one item:
Topmost Element of the stack: Item 4
To add the elements to the stack, we call the push()
method, and to print the first element of the stack, we call the peek()
function.
We then delete the top item using the pop()
method, and then we again call the peek()
method to check if the pop()
method removed the top item.
The benefit of this method over the previous example is that it takes less code to do the same operations.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn