How to Print Stack in Java
- Understanding the Stack Class in Java
- Printing Stack Values Using a Loop
-
Printing Stack Values Using the
pop()
Method - Printing Stack Values Using the Stream API
- Conclusion
- FAQ
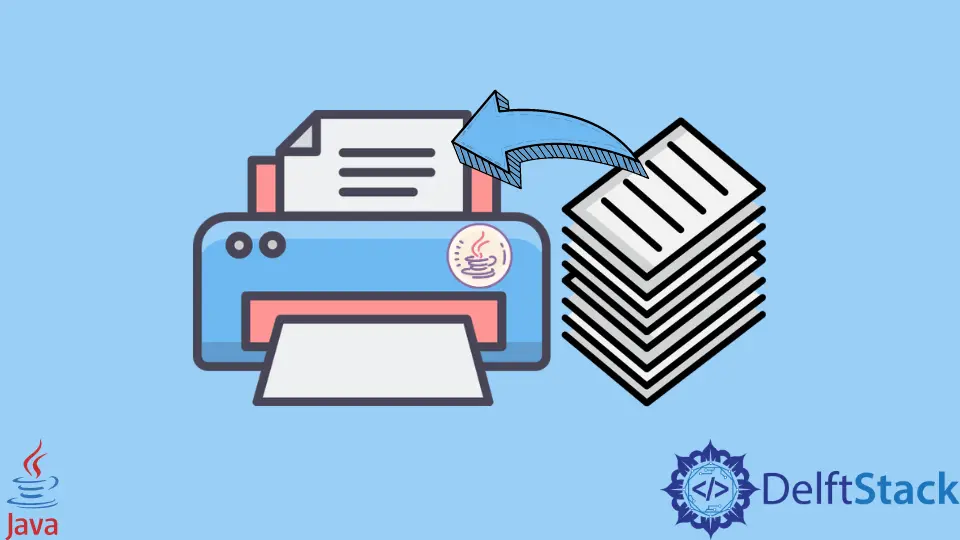
Printing stack values in Java can be a fundamental task, especially when you’re debugging or trying to understand the flow of your application. A stack is a collection of elements that follows the Last In First Out (LIFO) principle, meaning the last element added is the first one to be removed.
In this article, we will explore various methods to print stack values in Java, including how to utilize built-in classes and custom implementations. Whether you’re a beginner or an experienced developer, you’ll find practical examples and explanations that will enhance your Java programming skills. Let’s dive into the world of stacks and learn how to effectively print their values.
Understanding the Stack Class in Java
Before we dive into printing stack values, it’s essential to understand what a stack is in Java. The Stack class is a part of the Java Collections Framework and extends the Vector class. It represents a last-in, first-out stack of objects. You can perform various operations on a stack, such as pushing, popping, and peeking at elements.
Here’s a simple way to create a stack and add some elements to it:
import java.util.Stack;
public class StackExample {
public static void main(String[] args) {
Stack<Integer> stack = new Stack<>();
stack.push(10);
stack.push(20);
stack.push(30);
System.out.println("Stack: " + stack);
}
}
Output:
Stack: [10, 20, 30]
In this example, we created a stack of integers and pushed three values onto it. The System.out.println
statement prints the entire stack. This method is straightforward and provides a quick view of the stack’s contents.
Printing Stack Values Using a Loop
Another effective way to print stack values is by using a loop. This method allows you to customize the output format, which can be particularly useful for debugging purposes. Here’s how you can implement it:
import java.util.Stack;
public class LoopStackExample {
public static void main(String[] args) {
Stack<String> stack = new Stack<>();
stack.push("Apple");
stack.push("Banana");
stack.push("Cherry");
System.out.println("Printing stack values using a loop:");
for (int i = 0; i < stack.size(); i++) {
System.out.println(stack.get(i));
}
}
}
Output:
Printing stack values using a loop:
Apple
Banana
Cherry
In this code, we use a for loop to iterate through the stack’s elements. The get(i)
method retrieves each element based on its index. This approach gives you more control over how you present the stack’s contents, allowing for additional formatting or processing if needed.
Printing Stack Values Using the pop()
Method
If you want to print the stack values in reverse order (from top to bottom), you can use the pop()
method. This method removes the top element from the stack and returns it. Here’s how you can do this:
import java.util.Stack;
public class PopStackExample {
public static void main(String[] args) {
Stack<String> stack = new Stack<>();
stack.push("One");
stack.push("Two");
stack.push("Three");
System.out.println("Printing stack values using pop():");
while (!stack.isEmpty()) {
System.out.println(stack.pop());
}
}
}
Output:
Printing stack values using pop():
Three
Two
One
In this example, we continuously call pop()
until the stack is empty. This way, we print the elements in the reverse order of how they were added. This method is particularly useful when you need to process and remove elements from the stack simultaneously.
Printing Stack Values Using the Stream API
For those using Java 8 or later, the Stream API offers a modern and elegant way to handle collections, including stacks. You can easily convert a stack to a stream and print its elements. Here’s how:
import java.util.Stack;
import java.util.stream.Collectors;
public class StreamStackExample {
public static void main(String[] args) {
Stack<Integer> stack = new Stack<>();
stack.push(1);
stack.push(2);
stack.push(3);
System.out.println("Printing stack values using Stream API:");
String stackValues = stack.stream()
.map(String::valueOf)
.collect(Collectors.joining(", "));
System.out.println(stackValues);
}
}
Output:
Printing stack values using Stream API:
1, 2, 3
In this example, we convert the stack into a stream and use map
to convert each integer to a string. The collect
method then joins these strings into a single output string. This method is concise and leverages the power of functional programming in Java.
Conclusion
Printing stack values in Java is a straightforward task, yet it can be approached in various ways depending on your needs. Whether you prefer using loops, the pop()
method, or the Stream API, each method provides unique advantages. By understanding these different techniques, you can choose the best one for your specific use case, whether for debugging or displaying data. As you continue to explore Java, mastering these fundamental concepts will undoubtedly enhance your programming skills.
FAQ
-
What is a stack in Java?
A stack in Java is a collection that follows the Last In First Out (LIFO) principle, where the last element added is the first to be removed. -
How do I create a stack in Java?
You can create a stack in Java using the Stack class from the Java Collections Framework by callingnew Stack<Type>()
. -
Can I print a stack in reverse order?
Yes, you can print a stack in reverse order using thepop()
method, which removes and returns the top element of the stack. -
What is the Stream API in Java?
The Stream API is a feature introduced in Java 8 that allows for functional-style operations on collections, making it easier to process and manipulate data. -
Are there any performance considerations when using stacks?
Yes, while stacks are generally efficient, operations likepop()
can affect performance if used excessively in a large stack. Always consider the context of your application.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn