How to Set Color in Java
- the Importance of Setting Color
- Common Java Color Constants and RGB Values
- Setting Color in Java Swing GUI Components
-
Setting Color in Java With
Graphics2D
-
Setting Color With
java.awt.Color
- Best Practices With Setting Color
- Conclusion
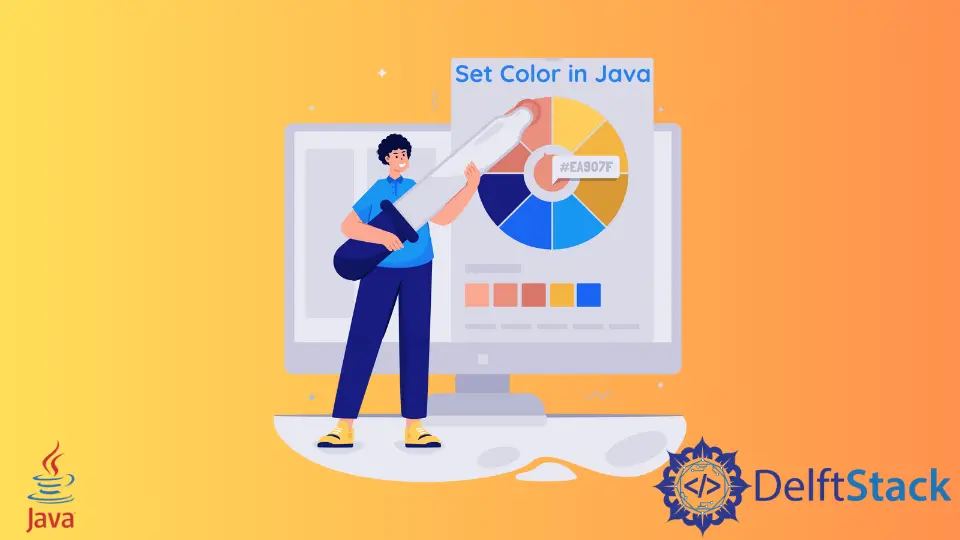
This article explores the significance of color setting in Java, emphasizing the usage of Java Swing GUI components, Graphics2D
, and java.awt.Color
, while incorporating best practices for effective and visually appealing application development.
the Importance of Setting Color
Setting color in Java is crucial for visual aesthetics, user experience, and effective data representation. In graphical user interfaces (GUIs), colors enhance clarity and highlight elements.
In data visualization, distinct colors aid in conveying information. Consistent and purposeful color choices contribute to a more appealing and comprehensible application, ultimately improving usability and user engagement.
In Java, there isn’t a specific setcolor
method that universally applies to all classes or objects. The way to set color depends on the context, such as whether you are working with GUI components, graphics, or other specific libraries.
Common Java Color Constants and RGB Values
Creating an exhaustive table of all possible Java color codes and their RGB values is not practical due to the vast number of colors and shades. However, I can provide you with an example table featuring some common Java Color constants and their corresponding RGB values:
Java Color Constant | RGB Value |
---|---|
Color.RED |
(255, 0, 0) |
Color.GREEN |
(0, 255, 0) |
Color.BLUE |
(0, 0, 255) |
Color.YELLOW |
(255, 255, 0) |
Color.CYAN |
(0, 255, 255) |
Color.MAGENTA |
(255, 0, 255) |
Color.BLACK |
(0, 0, 0) |
Color.WHITE |
(255, 255, 255) |
Color.GRAY |
(128, 128, 128) |
Color.LIGHT_GRAY |
(192, 192, 192) |
Color.DARK_GRAY |
(64, 64, 64) |
Color.ORANGE |
(255, 165, 0) |
Note: RGB values are represented as (Red, Green, Blue), each ranging from 0 to 255.
This is just a small subset of Java’s predefined colors. You can use these constants from the java.awt.Color
class or other color representations in different Java libraries.
If you have specific color requirements, you can use tools like the Color Picker in your IDE or external color pickers to get the RGB values for a particular color.
Setting Color in Java Swing GUI Components
Java Swing GUI components provide a user-friendly and platform-independent way to create graphical interfaces. They offer a consistent look and feel across different operating systems, enhancing the user experience.
Setting colors using Swing components ensures a standardized and cohesive design, simplifying development and promoting code portability. The extensive set of pre-built components and layout managers in Swing facilitates rapid application development with built-in support for color customization, making it a preferred choice for creating visually appealing and responsive Java applications.
Code Example:
import java.awt.*;
import javax.swing.*;
public class ColorSettingExample extends JFrame {
public ColorSettingExample() {
super("Setting Color in Java Swing");
// Creating a JPanel
JPanel panel = new JPanel();
// Setting background color of the panel
panel.setBackground(Color.CYAN);
// Creating a JButton
JButton button = new JButton("Click me");
// Setting foreground (text) color of the button
button.setForeground(Color.BLACK);
// Adding the button to the panel
panel.add(button);
// Adding the panel to the frame
getContentPane().add(panel);
// Setting frame properties
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setVisible(true);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new ColorSettingExample());
}
}
In this example, we’re creating a simple Swing application with a JFrame
containing a JPanel
and a JButton
. The crucial aspect here is the setting of colors for the panel and the button.
In this code breakdown, the initial step involves importing necessary classes from the javax.swing
and java.awt
packages to facilitate GUI components and color handling. Subsequently, a class named ColorSettingExample
is defined, extending JFrame
to serve as the main application window.
The constructor of this class is responsible for initializing the frame and includes the code for setting colors.
Within the constructor, a JPanel
is created, serving as a container for other components. The background color of this panel is set to cyan using the setBackground()
method.
Additionally, a JButton
is instantiated with the label Click me
, and its text color is set to black using the setForeground()
method.
The button is added to the panel, and the panel is, in turn, added to the content pane of the frame. To complete the setup, the frame’s properties, including size, close operation, and visibility, are configured.
Output:
Upon running the code, a GUI window will appear with a cyan-colored panel containing a black Click me
button. This visually demonstrates the effective setting of colors in Swing components.
The ability to set colors in Java Swing GUI components is a fundamental aspect of creating visually appealing and user-friendly applications. By understanding and utilizing methods like setBackground()
and setForeground()
, developers can enhance the aesthetics and usability of their graphical interfaces.
Setting Color in Java With Graphics2D
Graphics2D
in Java provides advanced drawing capabilities, allowing precise control over color, shapes, and rendering. It supports transformations, gradients, and complex graphics operations, offering a versatile platform for creating intricate and visually appealing user interfaces.
Unlike simpler methods, Graphics2D
enhances flexibility and creativity in color handling, making it indispensable for sophisticated graphics applications and custom visualizations.
Example Code:
import java.awt.*;
import javax.swing.*;
public class ColorSettingWithGraphics2D extends JPanel {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
// Setting drawing color to red
g2d.setColor(Color.RED);
// Drawing a filled rectangle
g2d.fillRect(50, 50, 100, 80);
// Setting drawing color to blue
g2d.setColor(Color.BLUE);
// Drawing an oval
g2d.fillOval(200, 50, 100, 80);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame("Color Setting with Graphics2D");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 200);
frame.setContentPane(new ColorSettingWithGraphics2D());
frame.setVisible(true);
});
}
}
In this example, we create a Swing application with a custom JPanel
. The paintComponent
method of this panel is overridden to draw various shapes with different colors using Graphics2D
.
In this code demonstration, the initial step involves importing essential classes for handling GUI components and graphic operations. Following this, a class is defined that extends JPanel
, serving as the designated canvas for drawing.
The paintComponent
method is then overridden to enable customized painting, with the Graphics
object cast to Graphics2D
for advanced drawing capabilities.
Subsequently, the drawing color is set to red using the setColor
method, initiating the rendering of a filled rectangle at specified coordinates (50, 50)
with a width of 100 and height of 80 through the fillRect
method. The color is subsequently changed to blue in preparation for the next drawing operation, where a filled oval is created at coordinates (200, 50)
with dimensions of 100 by 80 using the fillOval
method.
The main method orchestrates the setup of the application window by creating a JFrame
, configuring its properties, and establishing the content pane with an instance of the custom JPanel
. This sequential explanation provides a comprehensive understanding of the code’s structure and the purpose behind each step in creating a graphical interface with dynamically set colors in a Java Swing application.
Output:
Running this code will display a GUI window with a red-filled rectangle and a blue-filled oval. This showcases the effective use of the Graphics2D
class to set colors and draw various shapes dynamically, bringing life to the visual elements of a Java application.
Setting Color With java.awt.Color
In Java programming, the java.awt.Color
class serves as a fundamental component for managing colors. This class allows developers to represent and manipulate colors in various ways, making it a cornerstone for graphical and visual applications.
This article explores how to set color in Java using the java.awt.Color
method, emphasizing its significance in achieving color precision and flexibility in different contexts.
Example Code:
import java.awt.*;
import javax.swing.*;
public class ColorSettingWithAWTColor extends JPanel {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// Creating Color objects
Color redColor = new Color(255, 0, 0);
Color greenColor = new Color(0, 255, 0);
// Setting drawing color using Color objects
g.setColor(redColor);
// Drawing a filled rectangle
g.fillRect(50, 50, 100, 80);
// Setting drawing color using Color constants
g.setColor(Color.BLUE);
// Drawing an oval
g.fillOval(200, 50, 100, 80);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame("Color Setting with java.awt.Color");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 200);
frame.setContentPane(new ColorSettingWithAWTColor());
frame.setVisible(true);
});
}
}
In this illustration, a Swing application is crafted, incorporating a custom JPanel
to serve as the canvas for graphical rendering. The paintComponent
method within this JPanel
is intentionally overridden to exemplify the process of defining and applying colors using both Color
objects and predefined Color
constants.
With the necessary import statements, the code ensures access to essential packages, facilitating GUI components and efficient color management. Following this, a class is defined, extending JPanel
to establish the designated area for graphical content.
The paintComponent
method is a pivotal override, enabling customized painting operations. Within this method, Color
objects are instantiated, representing specific colors with defined RGB values.
Subsequently, the setColor
method is employed to set the drawing color to red, initiating the rendering of a filled rectangle at specified coordinates (50, 50)
with dimensions of 100 by 80 using the fillRect
method.
The code then demonstrates a seamless transition to utilizing Color
constants, with the color switched to blue in preparation for the subsequent drawing operation. By employing the fillOval
method, a filled oval is rendered at coordinates (200, 50)
with dimensions of 100 by 80.
Finally, the main method orchestrates the setup of the application window by creating a JFrame
, configuring its properties, and establishing the content pane with an instance of the custom JPanel
. This comprehensive explanation elucidates the sequence of actions and decisions involved in creating a graphical interface with dynamically set colors in a Java Swing application.
In this example, we create a Swing application with a custom JPanel
. The paintComponent
method is overridden to demonstrate setting colors using both Color
objects and predefined Color
constants.
The main method creates a JFrame
, sets its properties, and sets the content pane to an instance of our custom JPanel.
Output:
Running this code will display a GUI window with a red-filled rectangle and a blue-filled oval. The use of java.awt.Color
allows for color specification using RGB values and provides versatility in defining colors both through objects and predefined constants, showcasing the effectiveness of this method in achieving precise and flexible color representation.
Best Practices With Setting Color
Consistent Color Scheme
Maintain a consistent color scheme throughout your application to provide a visually cohesive and professional appearance. Use a defined set of colors that align with your application’s branding or design guidelines.
Use Named Colors
Whenever possible, use named colors from the java.awt.Color
class or equivalent color constants from GUI libraries. This enhances code readability and makes it easier for developers to understand the intended color.
Consider Accessibility
Be mindful of accessibility standards when choosing colors. Ensure that text and graphical elements have sufficient contrast for readability. Consider users with color vision deficiencies and aim for designs that are inclusive and accessible.
Separation of Concerns
Separate the concerns of color specification from the business logic. Utilize constants or configuration files to define colors, allowing for easy modification without altering the core application logic.
Utilize Color Constants
Leverage predefined color constants provided by GUI libraries or frameworks, such as Swing or JavaFX. This promotes consistency and reduces the likelihood of errors introduced by manual color specification.
Provide Flexibility
Allow for dynamic color changes when applicable. Implement options for users to customize colors or support themes to accommodate different preferences and user needs.
Document Color Choices
Document the rationale behind color choices in your code or project documentation. This is particularly important when using custom or non-standard colors, providing insights for maintenance and future development.
Use Color Classes Effectively
When working with the java.awt.Color
class or similar color classes, take advantage of their methods for manipulating colors. Methods like brighter()
, darker()
, and deriveColor()
can be useful for creating variations while maintaining a consistent base color.
Testing Across Devices
Test your application’s color choices on different devices and screens to ensure a consistent appearance. Account for variations in display technologies, such as different monitor types, resolutions, and color profiles.
Understand Color Spaces
Have a basic understanding of color spaces and models like RGB, HEX, and HSL. This knowledge can be valuable when specifying and manipulating colors programmatically.
Code Reviews for Color Usage
Incorporate color-related aspects into code reviews. This ensures that multiple team members review and align color choices, promoting a unified and cohesive visual design.
Avoid Hardcoding
Avoid hardcoding color values directly into your code. Instead, use constants or variables to represent colors, making it easier to update or change colors without modifying the code extensively.
Consider Cultural Implications
Be mindful of cultural associations with colors. Certain colors may have different meanings or significance in various cultures. Research cultural color symbolism to avoid unintended misinterpretations.
Responsive to User Feedback
Be open to user feedback regarding color choices. Users may have preferences or insights that can enhance the overall user experience. Consider incorporating user feedback into future updates or releases.
Conclusion
Setting color in Java is pivotal for creating visually appealing and user-friendly applications. Java Swing GUI components, Graphics2D
, and java.awt.Color
provide versatile tools for precise color control.
By following best practices, such as maintaining a consistent color scheme and considering accessibility, developers can ensure code clarity, accessibility, and a cohesive user experience. Whether using predefined colors or customizing hues, thoughtful color choices contribute significantly to the overall aesthetics and usability of Java applications.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn