How to Create Random Colors in Java
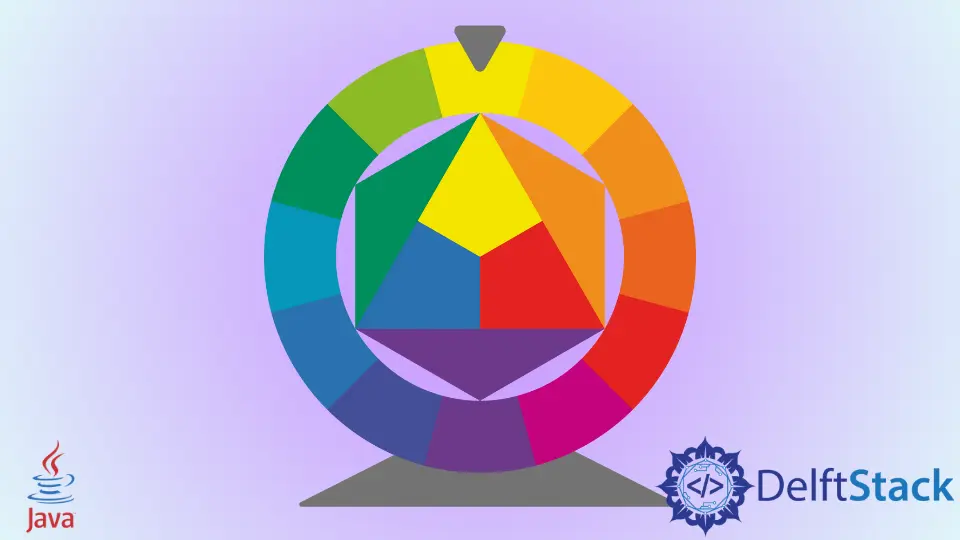
Color class is used to define colors that are in the standard RGB
color space or colors in any color space defined by the term color space. Each color is assigned an alpha number that is implicitly set to 1.0 as well as an explicit value that the constructor specifies. Alpha values define the transparency of a color. It is represented as an arbitrary float within the range of 0.0 between 1.0 or 0 to 255. A number that is 1.0 or 255 indicates that the hue is opaque, while the alpha values of zero or 0.0 indicate that it’s transparent.
When creating a color using an explicit alpha or when acquiring the alpha and color components of the color components, do not get pre-multiplied by an alpha component. The default color space of using the2D(tm) Java API 2D(tm) API will be sRGB, a proposed standard RGB color space.
We should make use of the random
library.
import java.util.Random;
Create a random generator.
Random rand = new Random();
Since colors are divided into blue, red, and green, it is possible to create an entirely new color by making random primary colors. Here Java Color
class accepts 3 floats, starting from zero to one.
float r = rand.nextFloat();
float g = rand.nextFloat();
float b = rand.nextFloat();
To create the final color, feed the primary colors into the constructor:
Color randomColor = new Color(r, g, b);
It is also possible to create various random effects by using this method by making random colors with a greater focus on specific colors. Pass in more blue and green to create a pinker random color. The following code can create a random color with more red (usually pinkish).
float r = rand.nextFloat();
float g = rand.nextFloat() / 2f;
float b = rand.nextFloat() / 2f;
To make sure there are only light colors are generated, you can generate colors that have a minimum of 0.5 of each element in the color spectrum. The following code will produce only light or bright shades.
float r = rand.nextFloat() / 2f + 0.5;
float g = rand.nextFloat() / 2f + 0.5;
float b = rand.nextFloat() / 2f + 0.5;
Many other color capabilities can be utilized within this Color
class, for example, making the color more bright.
randomColor.brighter();
Example Code
import java.awt.*;
import javax.swing.*;
class color extends JFrame {
// constructor
color() {
super("color");
Color c = Color.yellow;
JPanel p = new JPanel();
p.setBackground(c);
setSize(200, 200);
add(p);
show();
}
// Main Method
public static void main(String args[]) {
color c = new color();
}
}
Some Helpful Tips for Java Colors
- A strange place to start
random()
- This is either generatedcolor()
function or in a static initialization. At the moment, it is a bit odd that you have to add the random generator as a parameter for your program. You should consider using static initialization. - Strangely magic number
16777215
. What’s this number? It’s not entirely certain that it is the0xFFFFFF
that is the highest color value. It is important to note that nextInt(n)
gives an integer in the range between 0 and n; however, it does not include the number n. Also, you must use0x1000000
. - Tricking into appearing to be 6characters. If you multiply
0x1000000
by the number before converting it into Hexadecimal, you’ll get six digits. It means you do not require the trick to get six characters. You can also return as a substring (instead of using a loop)。