Difference Between Private and Public Modifiers in Java
-
Method With the
public
Modifier in Java -
Variable With the
public
Modifier in Java -
Constructor With the
public
Modifier in Java -
Method With the
private
Modifier in Java -
Variable With the
private
Modifier in Java -
Constructor With the
private
Modifier in Java
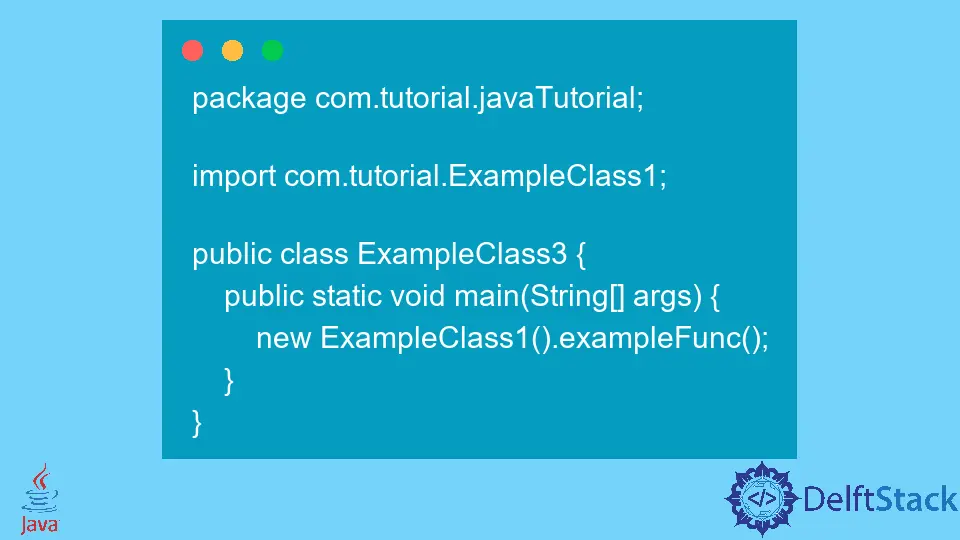
Today we will look at the significant differences between the two access modifiers called private
and public
, using some examples to understand better.
Method With the public
Modifier in Java
When we use the keyword public
with a method, its scope expands to the whole program, which means that this method can be accessed by any class and function from other packages too in the same project.
To demonstrate this scenario, we create three classes. The two classes ExampleClass1
and ExampleClass2
are in the same package called com.tutorial
while the third class ExampleClass3
is in another package named com.tutorial.javcaTutorial
.
In the ExampleClass1
class, we create a method exampleFunc()
with the public
keyword with a print statement inside. Next, we call this function in the ExampleClass2
class by creating an ExampleClass1
class object.
We also call the exampleFunc()
function from ExampleClass3
which is in another package. The whole program runs without any error, which means that the public
method is accessible to all these classes.
package com.tutorial;
public class ExampleClass1 {
public void exampleFunc() {
System.out.println("exampleFunc() called");
}
}
package com.tutorial;
public class ExampleClass2 {
public static void main(String[] args) {
new ExampleClass1().exampleFunc();
}
}
package com.tutorial.javaTutorial;
import com.tutorial.ExampleClass1;
public class ExampleClass3 {
public static void main(String[] args) {
new ExampleClass1().exampleFunc();
}
}
Output:
exampleFunc() called
Variable With the public
Modifier in Java
A public variable can be accessed from anywhere in the program. As shown in the below example, we can access the showMsg
variable from another class by creating its object.
public class ExampleClass1 {
public String showMsg = "This is a public variable";
}
class ExampleCLass extends ExampleClass1 {
public static void main(String[] args) {
System.out.println(new ExampleCLass().showMsg);
}
}
Output:
This is a public variable
Constructor With the public
Modifier in Java
A constructor can also be made public
and private
like the method and variable. If it is public
, we can create an object of the class anywhere like we do in this code.
package com.tutorial;
public class ExampleClass1 {
public String showMsg;
public ExampleClass1() {
showMsg = "Inside a constructor";
}
}
class ExampleCLass extends ExampleClass1 {
public static void main(String[] args) {
System.out.println(new ExampleCLass().showMsg);
}
}
Output:
Inside a constructor
Method With the private
Modifier in Java
Unlike the public
access modifier, the private
modifier restricts its members by making them non-accessible for out-of-scope classes.
Just like we did in the above section, we create three classes and try to call a function from both the classes. But we use the private
modifier instead of public
, and we get an error when running the program.
It happens because a private
modifier allows access to its members only inside the class that they were declared.
package com.tutorial;
public class ExampleClass1 {
private void exampleFunc() {
System.out.println("exampleFunc() called");
}
}
package com.tutorial;
public class ExampleClass2 {
public static void main(String[] args) {
new ExampleClass1().exampleFunc();
}
}
package com.tutorial.javaTutorial;
import com.tutorial.ExampleClass1;
public class ExampleClass3 {
public static void main(String[] args) {
new ExampleClass1().exampleFunc();
}
}
Output:
java: exampleFunc() has private access in com.tutorial.ExampleClass1
Variable With the private
Modifier in Java
We cannot access a private variable from another class even if the variable is in a parent class. It is shown in the following example that when accessed from an of ExampleCLass
it throws an error.
public class ExampleClass1 {
private String showMsg = "This is a public variable";
}
class ExampleCLass extends ExampleClass1 {
public static void main(String[] args) {
System.out.println(new ExampleCLass().showMsg);
}
}
Constructor With the private
Modifier in Java
A constructor used with the private
keyword restricts us from creating an object of the class as a constructor is needed to create an object. We cannot extend the class as its constructor is not available to the subclasses.
The code has two classes, ExampleClass1
contains a variable initialized in the class constructor. We make this constructor private.
When we extend ExampleClass1
in ExampleCLass
, we get an error saying that ExampleCLass1()
has private
access because its constructor is private.
package com.tutorial;
public class ExampleClass1 {
public String showMsg;
private ExampleClass1() {
showMsg = "Inside a constructor";
}
}
class ExampleCLass extends ExampleClass1 {
public static void main(String[] args) {
System.out.println(new ExampleCLass().showMsg);
}
}
Output:
java: ExampleClass1() has private access in com.tutorial.ExampleClass1
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn