Default Access Modifier in Java
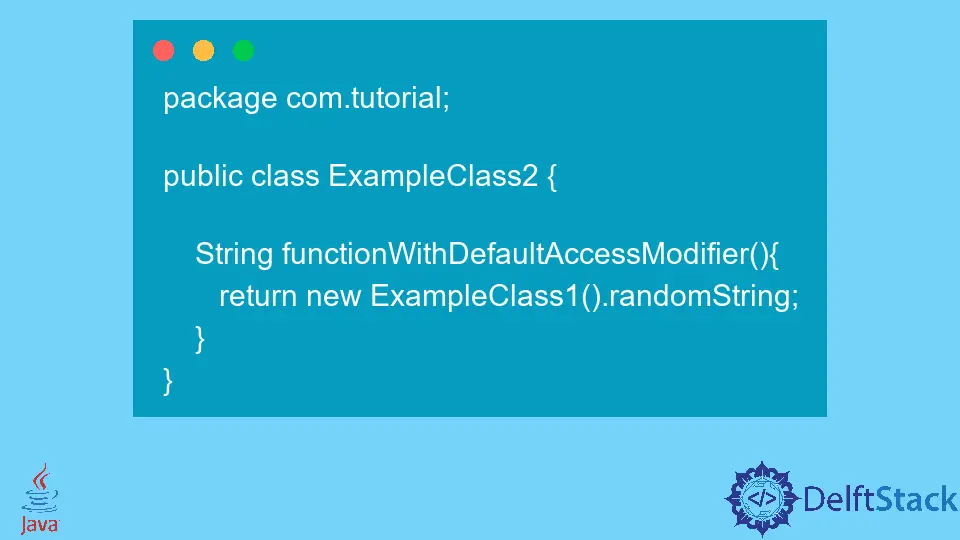
In this tutorial, we will talk about the default access modifier in Java. The name suggests that the access modifier is used when we do not specify anyone with the variable or a function.
Default Access Modifier In Java
There are four types of access
modifiers in Java, private
, protected
, public
, and default
. To understand the default
access modifier, we need to know why we need it and the other modifiers in different conditions.
The private
access modifier is the most strict because it only allows access to the same class. We use it when we don’t know that the class
variable or function will not be used anywhere else in the future besides the class we are in.
The protected
access modifier allows access within the class or the same package, but only the subclasses of the other packages can have access.
It is used when we want it to be available to all the classes in the package but does not want any other package
access.
As you can guess, the public
access modifier allows global access, which means we can access it anywhere, even from other packages.
The default
access modifier comes into play when we don’t use any above. Its access level is restricted to the same package, and other packages cannot access it even by creating subclasses.
Let us see an example of the default access
modifier and how it works. We have two classes, ExampleClass1
and ExampleClass2
. The first class contains a variable without any access modifier called randomString
.
Now in the ExampleClass2
class, we create a function and access the randomString
variable by making the object of ExampleClass1
. We can do it, and there is no error. It happens because both the classes are in the same package.
Notice the package name com.package
at the top of the classes.
Use the ExampleClass1
in Java
package com.tutorial;
public class ExampleClass1 {
String randomString = "This is a string from Example Class1";
public static void main(String[] args) {
String getReturnedString = new ExampleClass2().functionWithDefaultAccessModifier();
System.out.println(getReturnedString);
}
}
Use the ExampleClass2
in Java
package com.tutorial;
public class ExampleClass2 {
String functionWithDefaultAccessModifier() {
return new ExampleClass1().randomString;
}
}
Output:
This is a string from Example Class1
The randomString
can be accessed from another class in the same package in the above scenario.
Still, when we try to access the randomString
variable of the ExampleClass1
that is located in the com.tutorial
package from a class that is located in another package com.tutorial2
, the IDE
throws an error saying that the randomString
is not public and cannot be accessed from outside its package.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn