How to Create a Utility Class in Java
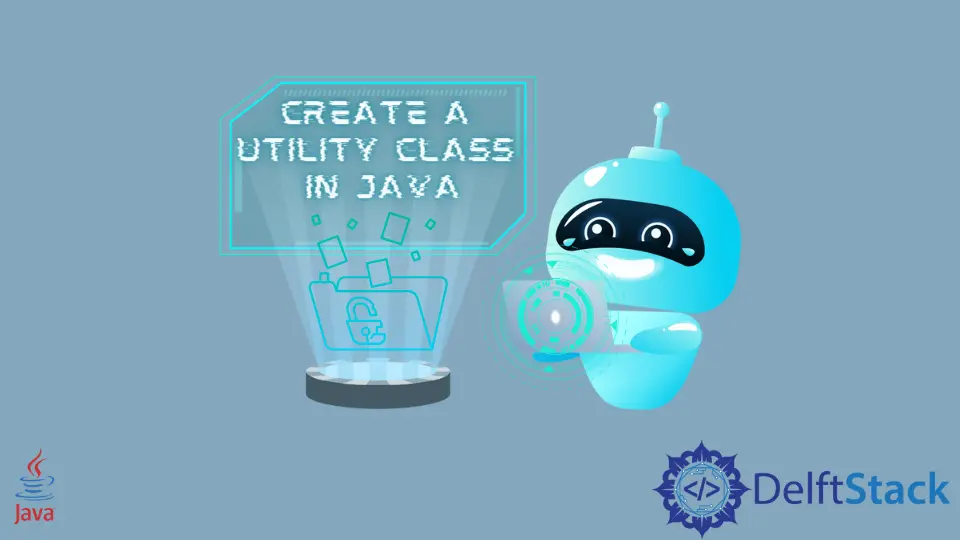
Utility classes in Java are also known as Helper Class. It is an efficient way to create methods that can be re-used. The code we need to use over and over again can be put inside a utility class.
Usage of Utility Class in Java
The Java utility class is a stateless class that cannot be instantiated and declared using final
and public
keywords. In the example given below, we have a UtilityClassExample
, which has a private constructor that prevents instantiation. For example, there are many examples of Util classes in Java like Apache StringUtils
, CollectionUtils
, or java.lang.Math
.
The methods in the utility class should be declared static
and not abstract
as object methods need instantiation. The final keyword prevents subclassing. Here, we create our own Utility class with a private
constructor, which, when invoked, throws an exception. Since we declared a private
constructor, default can not be created; hence class can not be instantiated.
In the code given below, we have all members of the UtilityClassExample
static. If we need to add or subtract two int
or float
type variables, we created methods in the utility class to re-use the code. We also have a method that returns a number multiplied by ten.
In the method addFloatValues()
, we have also used Math.round()
to round off the result to the nearest int. The Float
class has the sum()
method that returns the sum of two float arguments. We call each member method of this utility class passing arguments and print the output in the main()
method of the class TestUtitity
. Thus this utility class has methods that are used very often.
public final class UtilityClassExample {
private static final int constantValue = 10;
private UtilityClassExample() {
throw new java.lang.UnsupportedOperationException("Utility class and cannot be instantiated");
}
public static int addIntValues(int i, int j) {
int sum = i + j;
return sum;
}
public static int subIntValues(int i, int j) {
int diff = 0;
if (i > j) {
diff = i - j;
} else {
diff = j - i;
}
return diff;
}
public static float addFloatValues(float i, float j) {
float sum = Float.sum(i, j);
return Math.round(sum);
}
public static float subFloatValues(float i, float j) {
float diff = 0.00f;
if (i > j) {
diff = i - j;
} else {
diff = j - i;
}
return diff;
}
public static int returnValAfterMultiplying(int i) {
return i * constantValue;
}
}
class TestUtility {
public static void main(String[] args) {
int a = 4;
int b = 9;
int c = 7;
float d = 3.12f;
float e = 6.85f;
System.out.println(+a + " multiplied by ten is : "
+ UtilityClassExample.returnValAfterMultiplying(a));
System.out.println(b + "+" + c + " is : " + UtilityClassExample.addIntValues(b, c));
System.out.println(d + "+" + e + " is : " + UtilityClassExample.addFloatValues(d, e));
System.out.println(b + "-" + a + " is : " + UtilityClassExample.subIntValues(b, a));
System.out.println(e + "-" + d + " is : " + UtilityClassExample.subFloatValues(e, d));
}
}
Output:
4 multiplied by ten is : 40
9+7 is : 16
3.12+6.85 is : 10.0
9-4 is : 5
6.85-3.12 is : 3.73
It is not recommended to use a Utility
class of your own as it reduces flexibility.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn