在 Java 中创建实用程序类
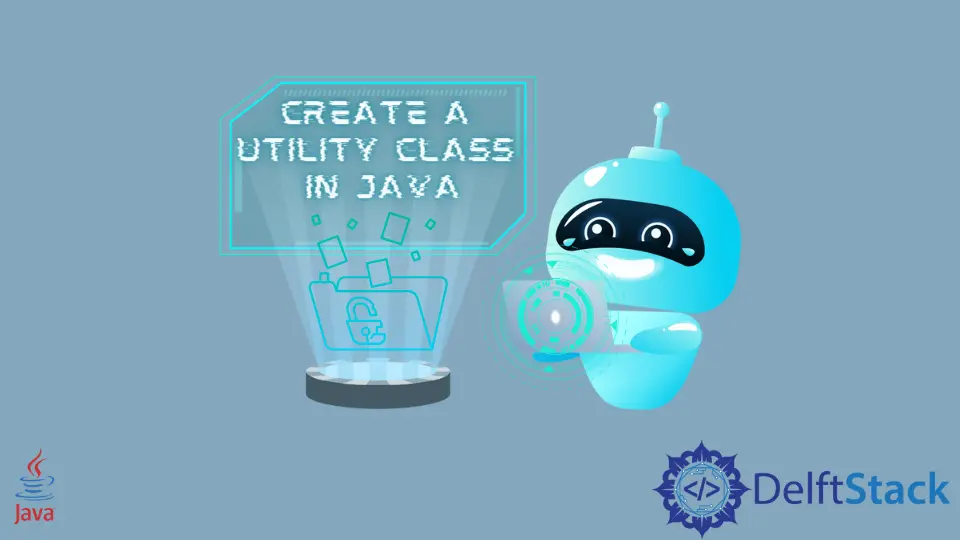
Java 中的实用程序类也称为 Helper 类。这是创建可重用方法的有效方法。我们需要反复使用的代码可以放在实用程序类中。
Java 中实用程序类的使用
Java 实用程序类是一个无状态类,不能使用 final
和 public
关键字进行实例化和声明。在下面给出的示例中,我们有一个 UtilityClassExample
,它有一个防止实例化的私有构造函数。例如,Java 中有许多 Util 类的示例,如 Apache StringUtils
、CollectionUtils
或 java.lang.Math
。
实用程序类中的方法应声明为静态
而不是抽象
,因为对象方法需要实例化。final 关键字防止子类化。在这里,我们使用 private
构造函数创建自己的 Utility 类,该构造函数在调用时会引发异常。由于我们声明了一个 private
构造函数,所以不能创建默认值;因此类不能被实例化。
在下面给出的代码中,我们拥有 UtilityClassExample
静态的所有成员。如果我们需要添加或减去两个 int
或 float
类型变量,我们在实用程序类中创建方法以重用代码。我们还有一个方法可以返回一个乘以 10 的数字。
在方法 addFloatValues()
中,我们还使用 Math.round()
将结果四舍五入到最接近的 int。Float
类具有 sum()
方法,该方法返回两个浮点参数的总和。我们调用这个实用程序类的每个成员方法传递参数,并在类 TestUtitity
的 main()
方法中打印输出。因此,该实用程序类具有经常使用的方法。
public final class UtilityClassExample {
private static final int constantValue = 10;
private UtilityClassExample() {
throw new java.lang.UnsupportedOperationException("Utility class and cannot be instantiated");
}
public static int addIntValues(int i, int j) {
int sum = i + j;
return sum;
}
public static int subIntValues(int i, int j) {
int diff = 0;
if (i > j) {
diff = i - j;
} else {
diff = j - i;
}
return diff;
}
public static float addFloatValues(float i, float j) {
float sum = Float.sum(i, j);
return Math.round(sum);
}
public static float subFloatValues(float i, float j) {
float diff = 0.00f;
if (i > j) {
diff = i - j;
} else {
diff = j - i;
}
return diff;
}
public static int returnValAfterMultiplying(int i) {
return i * constantValue;
}
}
class TestUtility {
public static void main(String[] args) {
int a = 4;
int b = 9;
int c = 7;
float d = 3.12f;
float e = 6.85f;
System.out.println(+a + " multiplied by ten is : "
+ UtilityClassExample.returnValAfterMultiplying(a));
System.out.println(b + "+" + c + " is : " + UtilityClassExample.addIntValues(b, c));
System.out.println(d + "+" + e + " is : " + UtilityClassExample.addFloatValues(d, e));
System.out.println(b + "-" + a + " is : " + UtilityClassExample.subIntValues(b, a));
System.out.println(e + "-" + d + " is : " + UtilityClassExample.subFloatValues(e, d));
}
}
输出:
4 multiplied by ten is : 40
9+7 is : 16
3.12+6.85 is : 10.0
9-4 is : 5
6.85-3.12 is : 3.73
不建议使用你自己的 Utility
类,因为它会降低灵活性。
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn