How to Insert a Tab Space in Java
-
Use the
- Use a Unicode Character to Insert a Tab in Java
-
Use the
String.format
Method to Insert a Tab in Java -
Use
StringBuilder
orStringBuffer
to Insert a Tab in Java -
Use
System.out.print
With Multiple Statements to Insert a Tab in Java - Conclusion
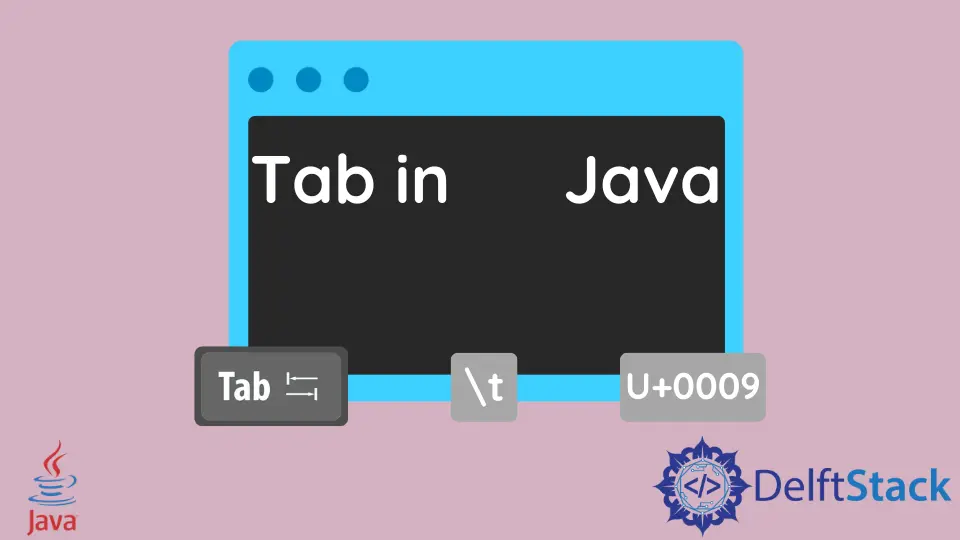
Inserting a tab in a Java program is a common requirement when you need to format text output. A tab character is represented by '\t'
in Java, and it typically advances the cursor to the next tab stop.
When a character in Java appears after the \
backslash, it is referred to as a Java escape character or escape sequence. This article will teach more about the \t
escape sequence and explore various methods to insert a tab in Java, along with detailed examples for each approach.
Use the
Tab Escape Sequence Character to Insert a Tab in Java
Java escape sequences are valid character literals used to perform a specific task. The escape sequence, \t
, is used for tab space.
In other words, \t
inserts a tab. We use an escape sequence when we need to format a string.
If we use \t
at a specific point in a string, it will insert a new tab at that point. The escape sequence for tab, \t
, can be used inside a print
statement, as given in the code below.
The string to be formatted is placed inside the double quotes. The escape sequence for tab \t
is placed between the words inside the ""
.
public class Test {
public static void main(String[] args) {
System.out.println("Happy\tCoding");
}
}
Output:
Happy Coding
As we can see in the output, it inserts a tab between the two words. We can format the string and insert a tab at any point in the text.
Use a Unicode Character to Insert a Tab in Java
We can also use the Unicode character U+0009
to insert a tab at a given point in the text to format it. Unicode is a standard to encode text representing almost every character of well-known languages globally.
It is a 16-bit character encoding standard. The Unicode that represents tab space is U+0009
.
The code below creates a string named tab
containing a Unicode escape sequence \u0009
, which represents a horizontal tab character. It then prints the string "Hello"
, followed by a tab character, and then "Everyone"
.
We used the +
operator to concatenate the two strings with the tab
variable in between them.
We pass the strings inside the print
function. The tab
variable will format the string and print it as an output.
public class Test {
public static void main(String[] args) {
String tab = "\u0009";
System.out.println("Hello" + tab + "Everyone");
}
}
Output:
Hello Everyone
In this output, the horizontal tab character (\u0009
) causes the cursor to move to the next tab stop after the word "Hello"
, creating a space between "Hello"
and "Everyone"
.
Use the String.format
Method to Insert a Tab in Java
The String.format
method is a member of the String
class in Java. It allows you to create formatted strings using a format specifier pattern.
This pattern enables you to control the alignment, width, precision, and other formatting options for various data types.
Its syntax is as follows:
String formattedString = String.format(format, arg1, arg2, ..., argn);
Here’s an explanation of the components:
format
: This is a string that specifies the format of the output. It may contain placeholders (format specifiers) that the values of the provided arguments will replace.arg1, arg2, ..., argn
: These are the arguments that will be inserted into the format string. They can be of various types, such as integers, floating-point numbers, strings, and more.
Format Specifiers
Format specifiers begin with a percent sign (%
) and are followed by optional format flags, width, precision, and a conversion character.
The format specifier syntax follows the pattern:
% [ flags ][width][.precision] conversion
%
: Denotes the start of the format specifier.flags
(optional): Optional flags for formatting (e.g.,-
for left-justification,0
for zero-padding).width
(optional): Specifies the minimum width of the formatted field. It can be an integer that represents the minimum number of characters the output should occupy..precision
(optional): For floating-point values, this specifies the number of decimal places. For strings, it specifies the maximum number of characters to be written.conversion
: Specifies the type of data to be formatted (e.g.,s
for string,d
for decimal,f
for floating point).
The String.format
method allows you to specify the width of the field, which can be used to insert tabs.
public class TabExample2 {
public static void main(String[] args) {
String formattedString = String.format("%-10s%-10s", "Hello", "World!");
System.out.println(formattedString);
}
}
This code demonstrates the use of the String.format
method to create a formatted string with tabs.
The code defines a class named TabExample2
. In the main
method, a string variable named formattedString
is declared and assigned a value using String.format
.
The format string "%-10s%-10s"
is used, specifying left-justification and a minimum field width of 10
characters for two strings. The strings "Hello"
and "World!"
are inserted into the format string.
The formatted string is then printed to the console. When executed, the output is: "Hello World!"
, with spaces used to create the appearance of a tab between "Hello"
and "World!"
.
The output will be:
Hello World!
Use StringBuilder
or StringBuffer
to Insert a Tab in Java
StringBuilder
and StringBuffer
are both classes that provide methods to manipulate strings. They allow you to efficiently build and modify strings in a mutable manner.
The main difference between StringBuffer
and StringBuilder
is that StringBuffer
is synchronized, making it thread-safe. This means that it can be safely used in a multi-threaded environment. StringBuilder
, on the other hand, is not synchronized and is preferred in single-threaded scenarios for its higher performance.
For most cases, StringBuilder
is preferred due to its higher performance unless thread safety is specifically required.
Using StringBuilder
The StringBuilder
class in Java is used to create and manipulate strings in a mutable way. It belongs to the java.lang
package and is part of the Java Standard Library.
Here is the basic syntax for using StringBuilder
in Java:
StringBuilder sb = new StringBuilder();
This creates a new instance of StringBuilder
with an initial capacity of 16 characters. If you know in advance that you will be appending a large number of characters, you can specify an initial capacity like this:
StringBuilder sb = new StringBuilder(initialCapacity);
Example of using StringBuilder
to insert a tab in Java:
public class StringBuilderTabExample {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder();
sb.append("Hello");
sb.append('\t'); // Insert a tab
sb.append("World!");
String result = sb.toString();
System.out.println(result);
}
}
In this example, we create a StringBuilder
named sb
. We use the append
method to concatenate "Hello"
and "World!"
.
To insert a tab, we append the tab character ('\t'
). Finally, we convert the StringBuilder
to a string using toString()
and print it.
The output will be:
Hello World!
Using StringBuffer
The StringBuffer
class in Java is used to create and manipulate strings in a mutable way, similar to StringBuilder
. It also belongs to the java.lang
package and is part of the Java Standard Library.
Below is the basic syntax for using StringBuffer
in Java:
StringBuffer sb = new StringBuffer();
This creates a new instance of StringBuffer
with an initial capacity of 16 characters. If you know in advance that you will be appending a large number of characters, you can specify an initial capacity like this:
StringBuffer sb = new StringBuffer(initialCapacity);
Example of using StringBuffer
to insert a tab in Java:
public class StringBufferTabExample {
public static void main(String[] args) {
StringBuffer sb = new StringBuffer();
sb.append("Hello");
sb.append('\t'); // Insert a tab
sb.append("World!");
String result = sb.toString();
System.out.println(result);
}
}
This example is similar to the StringBuilder
example, but we use a StringBuffer
instead.
The usage of StringBuffer
is essentially the same as StringBuilder
, but it provides thread safety at the cost of slightly lower performance.
The output will be:
Hello World!
Use System.out.print
With Multiple Statements to Insert a Tab in Java
The idea behind using multiple System.out.print
statements to insert a tab is to print different parts of the text separately. By doing this, we can control the spacing between the elements.
This method allows for precise control over the layout of the output.
Let’s start by looking at an example code that demonstrates this method:
public class TabExample {
public static void main(String[] args) {
System.out.print("Hello\t");
System.out.println("World!");
}
}
In this code example, the System.out.print("Hello\t");
line uses System.out.print
to print the string "Hello"
followed by a tab character (\t
). The print
method does not move to the next line after printing, so the cursor remains on the same line.
Then, the System.out.println("World!");
line prints the "World!"
string using the System.out.println
. The println
method moves to the next line after printing.
When you run this program, the output will be:
Hello World!
While using multiple System.out.print
statements is a straightforward way to insert a tab, it may not be the most efficient method if you need to format a large amount of text. In such cases, using other formatting techniques like String.format
or StringBuilder
may be more suitable.
Conclusion
This article has covered various methods for inserting tabs in a Java program.
We started with the \t
escape sequence, a direct way to represent a tab character in strings. We then explored using Unicode characters (U+0009
) as an alternative method.
Next, we discussed the String.format
method, which provides advanced formatting options, including tab insertion. We also examined the StringBuilder
and StringBuffer
classes, which are useful for efficiently constructing and modifying strings.
Finally, we looked at the technique of using multiple System.out.print
statements for precise control over text layout. Each method offers different levels of flexibility and efficiency, allowing developers to choose the most suitable approach for their specific needs.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn