How to Create A Roster Application in Java
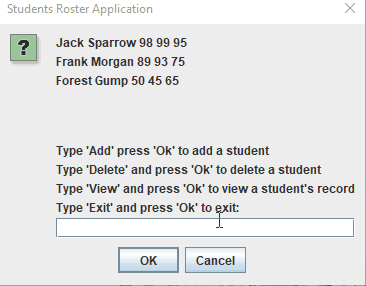
This tutorial demonstrates how to develop a roster application in Java.
Roster Application in Java
A Roster application gives details of the candidates’ records or the order details in which certain candidates have to perform a job. The Roster application is used to maintain the record of anything.
We have created an example for a simple Roster application that is used for the student record; the application can add/update, view, and delete a student’s record.
Student Roster Application in Java
As mentioned above, we have created a simple Roster application limited to some record-maintaining operations. A Roster application can contain as many options as used for record maintenance.
Our application performs the following operations:
- Add/Update - Add a record for new students, which includes names with three grades; it also updates the record of previous students.
- Delete - Delete a student record by just giving the name as input.
- View - View the record of a student with the top grade mentioned.
- Show - Show the list of students with grades on the main page.
Our application contains two classes; one is to create the student record, and the other is to maintain the student record. The system is based on Java Swing
.
Let us see the code for our student roster application:
Code- CreateStudent.java
:
package delftstack;
public class CreateStudent {
private String Student_Name;
private int[] Student_Grades;
// Default constructor
public CreateStudent() {
Student_Name = "";
for (int i = 0; i < Student_Grades.length; i++) {
Student_Grades[i] = 0;
}
}
// Constructor with inputs
public CreateStudent(String Name1, int[] Grade1) {
Student_Grades = new int[3];
SetStudentName(Name1);
SetStudentGrades(Grade1);
}
// Return the name of the students
public String GetStudentName() {
return Student_Name;
}
// Return the grades of the student
public int[] GetStudentGrades() {
return Student_Grades;
}
// To store the Name
public void SetStudentName(String Name1) {
Student_Name = Name1;
}
// To store or set the grades
public void SetStudentGrades(int[] Grade2) {
for (int i = 0; i < Grade2.length; i++) {
Student_Grades[i] = Grade2[i];
}
}
// Return the name and grades in string
public String ConverttoString() {
return (
Student_Name + " " + Student_Grades[0] + " " + Student_Grades[1] + " " + Student_Grades[2]);
}
// Returns the top grade
public int GetTopGrade(int[] Grade3) {
int top = Grade3[0];
for (int x = 0; x < Grade3.length; x++) {
if (top < Grade3[x]) {
top = Grade3[x];
}
}
return top;
}
}
The above class is used to create and retrieve the student record. It also returns the top grade of a particular student.
Code- MaintainStudent.java
:
package delftstack;
import java.text.*;
import java.util.Vector;
import javax.swing.*;
public class MaintainStudent {
// to determine the user input errors
static int Error_Flag = 0;
public static void main(String args[]) {
// The default value for grades
int[] Grade1 = {98, 99, 95};
int[] Grade2 = {89, 93, 75};
int[] Grade3 = {50, 45, 65};
// The default Student record
CreateStudent Student1 = new CreateStudent("Jack Sparrow", Grade1);
CreateStudent Student2 = new CreateStudent("Frank Morgan", Grade2);
CreateStudent Student3 = new CreateStudent("Forest Gump", Grade3);
// Create A vector of 'students' objects
Vector Students_Vector = new Vector();
Students_Vector.add(Student1);
Students_Vector.add(Student2);
Students_Vector.add(Student3);
String Option_Choice = "";
String Option_Message = "";
String Option_Text = "";
String Option_Input = "";
String Option_Record = "";
// To keep track of the top grade
int Top_Grade = 0;
// To Hold the student's grade
int[] Students_Grade = {0, 0};
int Student_Index1 = 0;
int Student_Index2 = 0;
int Student_Index3 = 0;
String Student_Grade_1 = "";
String Student_Grade_2 = "";
String Student_Grade_3 = "";
int Gr_1 = 0;
int Gr_2 = 0;
int Gr_3 = 0;
String Student_Name = "";
// To validate of input
boolean Retry_Student = false;
// To validate if the name is found
boolean Found_Student = false;
try {
while (!(Option_Choice.equalsIgnoreCase("Exit"))) {
Option_Message = "";
for (int i = 0; i < Students_Vector.size(); i++) {
Option_Message =
Option_Message + ((CreateStudent) Students_Vector.get(i)).ConverttoString() + "\n";
}
Option_Message = Option_Message
+ "\n\n\nType 'Add' press 'Ok' to add a student\nType 'Delete' and press 'Ok' to delete a student\nType 'View' and press 'Ok' to view a student's record\nType 'Exit' and press 'Ok' to exit:";
Option_Choice = JOptionPane.showInputDialog(
null, Option_Message, "Students Roster Application", JOptionPane.QUESTION_MESSAGE);
if (Option_Choice.equalsIgnoreCase("Add")) {
try {
Option_Input = JOptionPane.showInputDialog(null,
"Please enter student's name and grades\nwith no spaces between entries:\nFor Example: Sheeraz,87,88,99",
"Input", JOptionPane.QUESTION_MESSAGE);
Option_Input = Option_Input.trim();
// Reset the flag
Error_Flag = 0;
Retry_Student = Input_Validation(Option_Input);
if (1 == Error_Flag) {
JOptionPane.showMessageDialog(null,
"Wrong Entry: Please Enter a name which begins with a Letter!\nPlease click OK to retry.",
"Error", JOptionPane.ERROR_MESSAGE);
} else if (2 == Error_Flag) {
JOptionPane.showMessageDialog(null,
"Wrong Entry: The student's grade is missing!\nPlease click OK to retry.",
"Error", JOptionPane.ERROR_MESSAGE);
} else if (3 == Error_Flag) {
JOptionPane.showMessageDialog(null,
"Worng Entry: Student's grades digit limit exceeded, please enter grades with three digits.!\nPlease click OK to retry.",
"Error", JOptionPane.ERROR_MESSAGE);
} else if (4 == Error_Flag) {
JOptionPane.showMessageDialog(null,
"Worng Entry: Please enter a Student name it cannot be blank or ommitted!\nPlease click OK to retry.",
"Error", JOptionPane.ERROR_MESSAGE);
} else if (5 == Error_Flag) {
JOptionPane.showMessageDialog(null,
"Wrong Entry: Please do not add Blank spaces \nbefore or after the full name, commas and grades!\nPlease click OK to retry.",
"Error", JOptionPane.ERROR_MESSAGE);
}
// Check if the first input was invalid
if (true == Retry_Student) {
// ask the user for valid input
while (true == Retry_Student) {
Option_Input = JOptionPane.showInputDialog(null,
"Please Re-Enter student's info\nwith no spaces between entries:\nFor example: (Sheeraz Gul,87,88,99)",
"Input", JOptionPane.QUESTION_MESSAGE);
Option_Input = Option_Input.trim();
Retry_Student = Input_Validation(Option_Input);
if (1 == Error_Flag) {
JOptionPane.showMessageDialog(null,
"Wrong Entery: Please Enter a name which begins with a Letter!\nPlease click OK to retry.",
"Error", JOptionPane.ERROR_MESSAGE);
} else if (2 == Error_Flag) {
JOptionPane.showMessageDialog(null,
"Wrong Entry: The student's grade is missing!\nPlease click OK to retry.",
"Error", JOptionPane.ERROR_MESSAGE);
} else if (3 == Error_Flag) {
JOptionPane.showMessageDialog(null,
"Worng Entry: Student's grades digit limit exceeded, please enter grades with three digits.!\nPlease click OK to retry.",
"Error", JOptionPane.ERROR_MESSAGE);
} else if (4 == Error_Flag) {
JOptionPane.showMessageDialog(null,
"Worng Entry: Please enter a Student name it cannot be blank or ommitted!\nPlease click OK to retry.",
"Error", JOptionPane.ERROR_MESSAGE);
} else if (5 == Error_Flag) {
JOptionPane.showMessageDialog(null,
"Wrong Entry: Please do not add Blank spaces \\nbefore or after the full name, commas and grades!\nPlease click OK to retry.",
"Error", JOptionPane.ERROR_MESSAGE);
}
// Reset the flag
Error_Flag = 0;
}
}
} catch (IndexOutOfBoundsException e) {
Option_Input = JOptionPane.showInputDialog(null,
"Please Re-Enter the student's Infor \nwith no spaces between entries:\nFor example: (Sheeraz,87,88,99)",
"Input", JOptionPane.QUESTION_MESSAGE);
Option_Input = Option_Input.trim();
}
// All the validation is done above; if everything is fine, process the user input.
Student_Index1 = Option_Input.indexOf(",");
Student_Index2 = Option_Input.indexOf(",", Student_Index1 + 1);
Student_Index3 = Option_Input.indexOf(",", Student_Index2 + 1);
Student_Name = Option_Input.substring(0, Student_Index1);
Student_Grade_1 = Option_Input.substring(Student_Index1 + 1, Student_Index2);
Student_Grade_2 = Option_Input.substring(Student_Index2 + 1, Student_Index3);
Student_Grade_3 = Option_Input.substring(Student_Index3 + 1);
// Remove the spaces
Student_Name = Student_Name.trim();
Student_Grade_1 = Student_Grade_1.trim();
Student_Grade_2 = Student_Grade_2.trim();
Student_Grade_3 = Student_Grade_3.trim();
Gr_1 = Integer.parseInt(Student_Grade_1);
Gr_2 = Integer.parseInt(Student_Grade_2);
Gr_3 = Integer.parseInt(Student_Grade_3);
Grade1[0] = Gr_1;
Grade1[1] = Gr_2;
Grade1[2] = Gr_3;
Found_Student = false;
// To update the student's record if it exists
for (int p = 0; p < Students_Vector.size(); p++) {
if (Student_Name.equals(((CreateStudent) Students_Vector.get(p)).GetStudentName())) {
((CreateStudent) Students_Vector.get(p)).SetStudentGrades(Grade1);
Found_Student = true;
}
}
// add the student to the roster if it doesn't exist.
if (!Found_Student) {
CreateStudent student = new CreateStudent(Student_Name, Grade1);
Students_Vector.add(student);
}
// Inform the user
else {
JOptionPane.showMessageDialog(null,
"The student, " + Student_Name + " is updated!\nPlease click OK to go back.",
"Info", JOptionPane.INFORMATION_MESSAGE);
}
} else if (Option_Choice.equalsIgnoreCase("Delete")) {
Option_Input = JOptionPane.showInputDialog(null,
"Please enter student's name you want to delete:", "Input",
JOptionPane.QUESTION_MESSAGE);
Found_Student = false;
// Search for a name and delete it.
for (int i = 0; i < Students_Vector.size(); i++) {
if (Option_Input.equals(((CreateStudent) Students_Vector.get(i)).GetStudentName())) {
Students_Vector.remove(i);
Found_Student = true;
break;
}
}
// Go back to the menu if the user info is not found.
if (!Found_Student) {
JOptionPane.showMessageDialog(null,
"Please enter the correct name, this student does not exist!\nPlease click OK to go back.",
"Error", JOptionPane.ERROR_MESSAGE);
}
} else if (Option_Choice.equalsIgnoreCase("View")) {
Option_Input = JOptionPane.showInputDialog(null,
"Please enter the student's name to view the record:", "Input",
JOptionPane.QUESTION_MESSAGE);
Found_Student = false;
// Search for a name and show the record
for (int m = 0; m < Students_Vector.size(); m++) {
if (Option_Input.equals(((CreateStudent) Students_Vector.get(m)).GetStudentName())) {
Students_Grade = ((CreateStudent) Students_Vector.get(m)).GetStudentGrades();
Top_Grade = ((CreateStudent) Students_Vector.get(m)).GetTopGrade(Students_Grade);
Option_Record = ((CreateStudent) Students_Vector.get(m)).ConverttoString()
+ "\nThe Top Grade: " + Top_Grade;
JOptionPane.showMessageDialog(
null, Option_Record, Option_Input, JOptionPane.INFORMATION_MESSAGE);
Found_Student = true;
break;
}
}
// If no record is found, go back to the menu.
if (!Found_Student) {
JOptionPane.showMessageDialog(null,
"Please enter the correct name, this student does not exist!\nPlease click OK to go back.",
"Error", JOptionPane.ERROR_MESSAGE);
}
} else {
// If the user types anything other than "Add", "Delete", "View", or "Exit", then inform
// him.
if (!(Option_Choice.equalsIgnoreCase("Exit"))) {
if (Option_Choice.trim().equals("")) {
JOptionPane.showMessageDialog(null,
"Please first make a selection then click OK!\nPlease click OK to go back.",
"Error", JOptionPane.ERROR_MESSAGE);
} else {
JOptionPane.showMessageDialog(null,
"Please enter the correct option, this option does not exist!\nPlease click OK to go back.",
"Error", JOptionPane.ERROR_MESSAGE);
}
}
}
}
} catch (NullPointerException e) {
System.exit(0);
}
System.exit(0);
}
// For input validation
private static boolean Input_Validation(String Demo_String) {
boolean R_Value = false;
int Comma_Count = 0;
int Index1_Comma = 0;
int Index2_Comma = 0;
int Index3_Comma = 0;
char Comma_Char;
boolean Comma_Position = true;
String String_Name = "";
String String_Grade1 = "";
String String_Grade2 = "";
String String_Grade3 = "";
int String_Grade_1 = 0;
int String_Grade_2 = 0;
int String_Grade_3 = 0;
// Check for a blank string
if ((0 == Demo_String.length()) || (Demo_String.equals(""))) {
R_Value = true;
}
// Check for commas
for (int i = 0; i < Demo_String.length(); i++) {
if ((Demo_String.charAt(i)) == ',') {
Comma_Count++;
}
}
// Check for the positions of commas and make sure there are three commas
if (3 == Comma_Count) {
Index1_Comma = Demo_String.indexOf(",");
Index2_Comma = Demo_String.indexOf(",", Index1_Comma + 1);
Index3_Comma = Demo_String.indexOf(",", Index2_Comma + 1);
if (Index2_Comma == Index1_Comma + 1) {
R_Value = true;
Comma_Position = false;
} else if (Index3_Comma == Index2_Comma + 1) {
R_Value = true;
Comma_Position = false;
}
} else {
R_Value = true;
}
// Dissects the 'string' to store the data
if ((3 == Comma_Count) && (true == Comma_Position)) {
String_Name = Demo_String.substring(0, Index1_Comma);
String_Grade1 = Demo_String.substring(Index1_Comma + 1, Index2_Comma);
String_Grade2 = Demo_String.substring(Index2_Comma + 1, Index3_Comma);
String_Grade3 = Demo_String.substring(Index3_Comma + 1);
// Compare the string with the trimmed string because white spaces are not allowed.
if (!(String_Name.equals(String_Name.trim()))) {
Error_Flag = 5;
R_Value = true;
}
if (!(String_Grade1.equals(String_Grade1.trim()))) {
Error_Flag = 5;
R_Value = true;
}
if (!(String_Grade2.equals(String_Grade2.trim()))) {
Error_Flag = 5;
R_Value = true;
}
if (!(String_Grade3.equals(String_Grade3.trim()))) {
Error_Flag = 5;
R_Value = true;
}
// Once the flag is set, return to call the program.
if (0 < Error_Flag) {
return R_Value;
}
// Remove the white spaces
String_Name = String_Name.trim();
String_Grade1 = String_Grade1.trim();
String_Grade2 = String_Grade2.trim();
String_Grade3 = String_Grade3.trim();
// Check for the empty names or null values
if ((String_Name.equals("")) || (null == String_Name)) {
Error_Flag = 4;
R_Value = true;
}
if (0 < Error_Flag) {
return R_Value;
}
// Make sure the name starts with a letter.
if (!((String_Name.equals("")) || (null == String_Name))) {
int ASCII = Demo_String.charAt(0);
if (ASCII < 65) {
Error_Flag = 1;
R_Value = true;
} else if ((ASCII > 58) && (ASCII < 65)) {
Error_Flag = 1;
R_Value = true;
} else if (ASCII > 122) {
Error_Flag = 1;
R_Value = true;
}
}
if (0 < Error_Flag) {
return R_Value;
}
// Check for empty grades or null values
if ((String_Grade1.equals("")) || (null == String_Grade1)) {
Error_Flag = 2;
R_Value = true;
} else if ((String_Grade2.equals("")) || (null == String_Grade2)) {
Error_Flag = 2;
R_Value = true;
} else if ((String_Grade3.equals("")) || (null == String_Grade3)) {
Error_Flag = 2;
R_Value = true;
}
if (0 < Error_Flag) {
return R_Value;
}
// Make sure the grades contain only numbers
try {
String_Grade_1 = Integer.parseInt(String_Grade1);
String_Grade_2 = Integer.parseInt(String_Grade2);
String_Grade_3 = Integer.parseInt(String_Grade3);
} catch (NumberFormatException e) {
R_Value = true;
}
// Make sure the grades have only three digits
if (3 < String_Grade1.length()) {
Error_Flag = 3;
R_Value = true;
} else if (3 < String_Grade2.length()) {
Error_Flag = 3;
R_Value = true;
} else if (3 < String_Grade3.length()) {
Error_Flag = 3;
R_Value = true;
}
}
return R_Value;
}
}
The MaintainStudent
class is used to maintain the student’s record; it performs the add/update, deletes, view, and exit operations. This class also contains the driver’s main method.
Let’s try to run the application and see the output for different operations:
-
Main Page:
-
Add/Update Student Record:
To add a new student record, type
Add
and pressOk
. If you want to update a record, typeAdd
, pressOk
, and type the student’s name with new grades, and the record will be updated.Add:
Update:
-
Delete Student Record:
To delete a student record, type
Delete
, pressOk
, and then type the student’s name to delete a record: -
View Student Record:
To view a student record, type
View
, pressOk
, and then type the student’s name to display a student record. It will also display the top grade of the student. -
Exit Roster:
To exit the student roster application, type
Exit
and pressOk
.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook