How to Restart a Program in Java
-
Use a
do-while
Conditional Statement -
Use a
do-while
Conditional Statement Without a Stopping Condition - Use Recursion to Restart a Program
- Use Recursion to Restart a Program Without a Stopping Condition
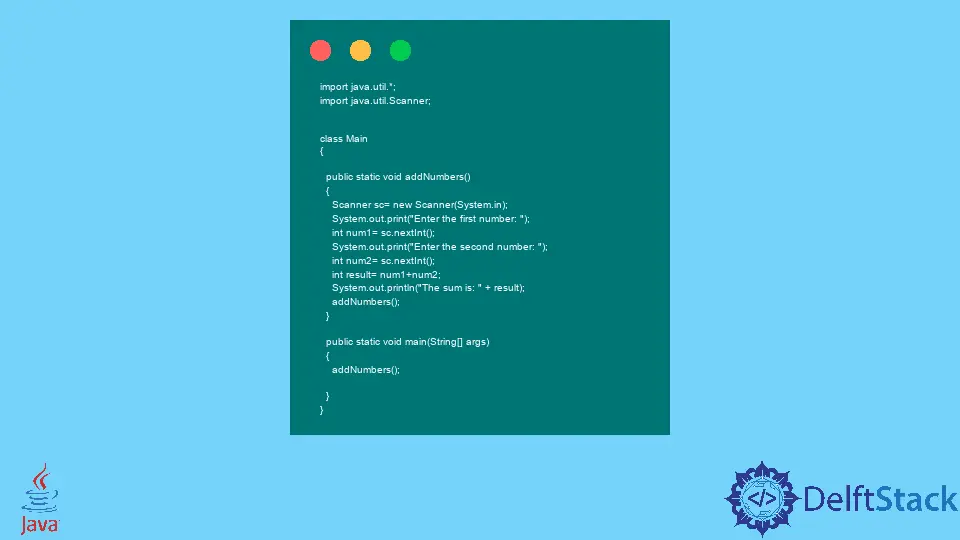
In a menu-driven program or a game, we require an option to restart or reset our program. We can restart a program in Java by recursively calling a function or using conditional loop statements.
Use a do-while
Conditional Statement
import java.util.*;
import java.util.Scanner;
class Main {
public static int addNumbers(int a, int b) {
return a + b;
}
public static void main(String[] args) {
boolean flag = false;
Scanner sc = new Scanner(System.in);
do {
System.out.print("Enter the first number: ");
int num1 = sc.nextInt();
System.out.print("Enter the second number: ");
int num2 = sc.nextInt();
int result = addNumbers(num1, num2);
System.out.println("The sum is: " + result);
System.out.print("Do you want to add more numbers? Enter y for yes and n for no. ");
char input = sc.next().charAt(0);
if (input == 'y') {
flag = true;
} else {
flag = false;
}
} while (flag);
}
}
Output:
Enter the first number: 8
Enter the second number: 7
The sum is: 15
Do you want to add more numbers? Enter y for yes and n for no. y
Enter the first number: 9
Enter the second number: 4
The sum is: 13
Do you want to add more numbers? Enter y for yes and n for no. n
The above code displays the functionality of a conditional loop for restarting a function again; as long as the user inputs y
, the program will restart.
Use a do-while
Conditional Statement Without a Stopping Condition
import java.util.*;
import java.util.Scanner;
class Main {
public static int addNumbers(int a, int b) {
return a + b;
}
public static void main(String[] args) {
boolean flag = true;
Scanner sc = new Scanner(System.in);
do {
System.out.print("Enter the first number: ");
int num1 = sc.nextInt();
System.out.print("Enter the second number: ");
int num2 = sc.nextInt();
int result = addNumbers(num1, num2);
System.out.println("The sum is: " + result);
} while (flag);
}
}
Output:
Enter the first number: 8
Enter the second number: 7
The sum is: 15
Enter the first number: 9
Enter the second number: 4
The sum is: 13
Enter the first number: .....
The above code displays the functionality of a conditional loop for restarting a function an infinite number of times. The only way to stop the program from restarting is to close the program.
Use Recursion to Restart a Program
import java.util.*;
import java.util.Scanner;
class Main {
public static void addNumbers(int a, int b, int c) {
Scanner sc = new Scanner(System.in);
if (c == 0) {
int result = a + b;
System.out.println("The sum is: " + result);
return;
}
int result = a + b;
System.out.println("The sum is: " + result);
System.out.print("Enter the first number: ");
int num1 = sc.nextInt();
System.out.print("Enter the second number: ");
int num2 = sc.nextInt();
c--;
addNumbers(num1, num2, c);
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter the first number: ");
int num1 = sc.nextInt();
System.out.print("Enter the second number: ");
int num2 = sc.nextInt();
System.out.print("How many times do you want to restart this program? : ");
int num3 = sc.nextInt();
addNumbers(num1, num2, num3);
}
}
Output:
Enter the first number: 5
Enter the second number: 6
How many times do you want to restart this program? : 2
The sum is: 11
Enter the first number: 4
Enter the second number: 5
The sum is: 9
Enter the first number: 7
Enter the second number: 8
The sum is: 15
The above code displays the functionality of a recursive function for restarting itself again. The program will restart as many times as the user requires.
Use Recursion to Restart a Program Without a Stopping Condition
import java.util.*;
import java.util.Scanner;
class Main {
public static void addNumbers() {
Scanner sc = new Scanner(System.in);
System.out.print("Enter the first number: ");
int num1 = sc.nextInt();
System.out.print("Enter the second number: ");
int num2 = sc.nextInt();
int result = num1 + num2;
System.out.println("The sum is: " + result);
addNumbers();
}
public static void main(String[] args) {
addNumbers();
}
}
Output:
Enter the first number: 5
Enter the second number: 3
The sum is: 8
Enter the first number: 8
Enter the second number: 11
The sum is: 19
Enter the first number: ...
The above code displays the functionality of a recursive function for restarting itself again. The program will restart an infinite number of times as there is no stopping condition in the recursive call cycle.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn