Java でプログラムを再起動する
Musfirah Waseem
2023年10月12日
-
do-while
条件ステートメントを使用する -
do-while
条件付きステートメントを停止条件なしで使用する - 再帰を使用してプログラムを再起動する
- 再帰を使用して停止条件なしでプログラムを再開する
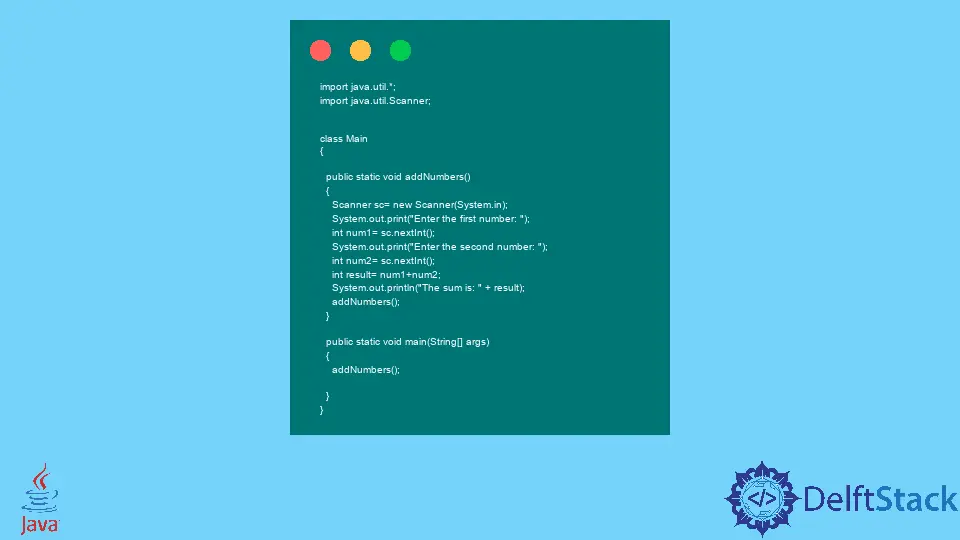
メニュー駆動型のプログラムまたはゲームでは、プログラムを再起動またはリセットするオプションが必要です。 関数を再帰的に呼び出すか、条件付きループ ステートメントを使用することで、Java でプログラムを再開できます。
do-while
条件ステートメントを使用する
import java.util.*;
import java.util.Scanner;
class Main {
public static int addNumbers(int a, int b) {
return a + b;
}
public static void main(String[] args) {
boolean flag = false;
Scanner sc = new Scanner(System.in);
do {
System.out.print("Enter the first number: ");
int num1 = sc.nextInt();
System.out.print("Enter the second number: ");
int num2 = sc.nextInt();
int result = addNumbers(num1, num2);
System.out.println("The sum is: " + result);
System.out.print("Do you want to add more numbers? Enter y for yes and n for no. ");
char input = sc.next().charAt(0);
if (input == 'y') {
flag = true;
} else {
flag = false;
}
} while (flag);
}
}
出力:
Enter the first number: 8
Enter the second number: 7
The sum is: 15
Do you want to add more numbers? Enter y for yes and n for no. y
Enter the first number: 9
Enter the second number: 4
The sum is: 13
Do you want to add more numbers? Enter y for yes and n for no. n
上記のコードは、関数を再起動するための条件付きループの機能を示しています。 ユーザーが y
を入力する限り、プログラムは再起動します。
do-while
条件付きステートメントを停止条件なしで使用する
import java.util.*;
import java.util.Scanner;
class Main {
public static int addNumbers(int a, int b) {
return a + b;
}
public static void main(String[] args) {
boolean flag = true;
Scanner sc = new Scanner(System.in);
do {
System.out.print("Enter the first number: ");
int num1 = sc.nextInt();
System.out.print("Enter the second number: ");
int num2 = sc.nextInt();
int result = addNumbers(num1, num2);
System.out.println("The sum is: " + result);
} while (flag);
}
}
出力:
Enter the first number: 8
Enter the second number: 7
The sum is: 15
Enter the first number: 9
Enter the second number: 4
The sum is: 13
Enter the first number: .....
上記のコードは、関数を無限回再起動するための条件付きループの機能を示しています。 プログラムの再起動を停止する唯一の方法は、プログラムを閉じることです。
再帰を使用してプログラムを再起動する
import java.util.*;
import java.util.Scanner;
class Main {
public static void addNumbers(int a, int b, int c) {
Scanner sc = new Scanner(System.in);
if (c == 0) {
int result = a + b;
System.out.println("The sum is: " + result);
return;
}
int result = a + b;
System.out.println("The sum is: " + result);
System.out.print("Enter the first number: ");
int num1 = sc.nextInt();
System.out.print("Enter the second number: ");
int num2 = sc.nextInt();
c--;
addNumbers(num1, num2, c);
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter the first number: ");
int num1 = sc.nextInt();
System.out.print("Enter the second number: ");
int num2 = sc.nextInt();
System.out.print("How many times do you want to restart this program? : ");
int num3 = sc.nextInt();
addNumbers(num1, num2, num3);
}
}
出力:
Enter the first number: 5
Enter the second number: 6
How many times do you want to restart this program? : 2
The sum is: 11
Enter the first number: 4
Enter the second number: 5
The sum is: 9
Enter the first number: 7
Enter the second number: 8
The sum is: 15
上記のコードは、再起動するための再帰関数の機能を示しています。 プログラムは、ユーザーが必要とする回数だけ再起動します。
再帰を使用して停止条件なしでプログラムを再開する
import java.util.*;
import java.util.Scanner;
class Main {
public static void addNumbers() {
Scanner sc = new Scanner(System.in);
System.out.print("Enter the first number: ");
int num1 = sc.nextInt();
System.out.print("Enter the second number: ");
int num2 = sc.nextInt();
int result = num1 + num2;
System.out.println("The sum is: " + result);
addNumbers();
}
public static void main(String[] args) {
addNumbers();
}
}
出力:
Enter the first number: 5
Enter the second number: 3
The sum is: 8
Enter the first number: 8
Enter the second number: 11
The sum is: 19
Enter the first number: ...
上記のコードは、再起動するための再帰関数の機能を示しています。 再帰呼び出しサイクルには停止条件がないため、プログラムは無限に再起動します。
著者: Musfirah Waseem
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn