How to Read File From Classpath in Java
-
Importance of Reading Files From
Classpath
in Java -
Reading Files From
Classpath
UsinggetResource()
Method in Java -
Reading Files From
Classpath
UsingClassLoader.getResourceAsStream()
in Java -
Reading Files From
Classpath
UsingClass.getResourceAsStream()
in Java -
Reading Files From
Classpath
UsingPaths
andFiles
(Java 7 and Later) -
Best Practices for Reading Files From
Classpath
in Java - Conclusion
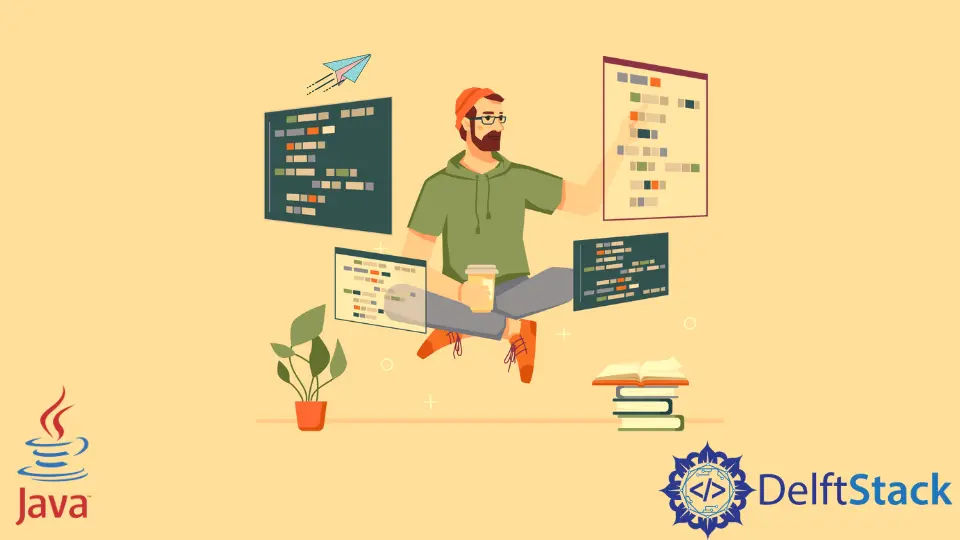
Explore the significance, methods, and best practices of reading files from the classpath
in Java, understanding the crucial aspects that contribute to flexible and maintainable code.
Importance of Reading Files From Classpath
in Java
Reading files from the classpath
in Java is crucial for portability and accessibility. By utilizing the classpath
, Java applications can access resources irrespective of their absolute file system paths, ensuring adaptability across various deployment environments.
This approach simplifies code maintenance and enhances the versatility of applications, making them independent of specific directory structures. Reading files from the classpath
is especially vital in scenarios like JAR packaging, where resources are bundled, allowing developers to create more robust and portable Java applications with ease.
Reading Files From Classpath
Using getResource()
Method in Java
The getResource()
method in Java is crucial for reading files from the classpath
as it provides a concise and portable way to obtain a resource’s URL. This method simplifies resource access, making the code more flexible and independent of specific file system paths.
Unlike absolute paths, getResource()
ensures adaptability across deployment environments, facilitating code modularity. It is particularly advantageous in scenarios where the file’s location might vary, such as when packaged within JAR files.
The following code exemplifies how to utilize the getResource()
method to read a file from the classpath
.
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.URL;
public class FileReadFromClasspath {
public static void main(String[] args) {
// Obtaining the URL of the resource
URL resourceUrl = FileReadFromClasspath.class.getResource("/sample.txt");
if (resourceUrl != null) {
try (InputStream inputStream = resourceUrl.openStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
// Reading and printing each line from the file
reader.lines().forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
} else {
System.out.println("File not found!");
}
}
}
The code uses the getResource()
method to obtain a URL pointing to the specified resource, in this case, a file named sample.txt
. The leading /
in FileReadFromClasspath.class.getResource("/sample.txt")
denotes an absolute path within the classpath
.
The obtained URL is then used to open an InputStream
, serving as a conduit for reading the file’s content. To efficiently read the file line by line, a BufferedReader
is wrapped around an InputStreamReader
.
The lines are read and printed to the console using reader.lines().forEach(System.out::println)
with a concise lambda expression. Exception handling is implemented through a try-with-resources
block, ensuring proper resource closure and catching any potential IOException
.
This approach simplifies file access from the classpath
, promoting flexibility and modularity in Java applications.
Output:
Assuming the sample.txt
file contains the following content:
Hello! My name is Pillow and I am a Chihuahua!
The output of the program would be:
This method provides a straightforward way to obtain a URL pointing to a resource, allowing developers to read files with ease. By incorporating this approach, your Java applications gain flexibility and modularity, enabling seamless resource access in various deployment scenarios.
The example code demonstrates the simplicity and effectiveness of using getResource()
, making it a valuable technique in the toolkit of every Java developer.
Reading Files From Classpath
Using ClassLoader.getResourceAsStream()
in Java
The ClassLoader.getResourceAsStream()
method in Java is vital for reading files from the classpath
, offering a streamlined and resource-efficient approach. It provides an InputStream
directly, avoiding the need for complex file path constructions.
This method ensures code portability, as it is not dependent on the underlying file system structure. Particularly useful in JAR-packaged applications, it simplifies access to resources, promoting cleaner and more adaptable code.
The approach is concise, reducing boilerplate code and enhancing the overall readability of Java applications, making it an essential choice for efficient file handling in diverse deployment environments.
Let’s dive into a practical example to demonstrate how to use ClassLoader.getResourceAsStream()
to read a file from the classpath
. Suppose we have a text file named sample.txt
located in the resources
directory of our project.
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
public class FileReadFromClasspath {
public static void main(String[] args) {
// Using the ClassLoader to load the resource
InputStream inputStream =
FileReadFromClasspath.class.getClassLoader().getResourceAsStream("sample.txt");
if (inputStream != null) {
try (BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
String line;
// Reading and printing each line of the file
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
} else {
System.out.println("File not found!");
}
}
}
The process involves obtaining the class loader using FileReadFromClasspath.class.getClassLoader()
. We then call getResourceAsStream("sample.txt")
on the class loader, specifying the file’s relative path in the classpath
.
This yields an InputStream
enabling file content reading. To efficiently read line by line, we use a BufferedReader
wrapped around an InputStreamReader
for byte-to-character conversion.
Within a try
block, a while
loop reads each line, printing it to the console. A try-with-resources
block ensures proper resource closure and catches potential IOExceptions
during file reading.
This method simplifies file access, promoting clean and concise code in Java applications.
Assuming the sample.txt
file contains the following content:
Hello! My name is Pillow and I am a Chihuahua!
The output of the program would be:
This technique enhances the flexibility and portability of Java applications, allowing developers to access resources seamlessly, irrespective of the deployment environment. By mastering this approach, developers can build more robust and adaptable solutions in their Java projects.
Reading Files From Classpath
Using Class.getResourceAsStream()
in Java
The Class.getResourceAsStream()
method in Java is crucial for reading files from the classpath
, offering simplicity and direct resource access. It eliminates the need for obtaining a class loader explicitly, streamlining code.
Unlike ClassLoader.getResourceAsStream()
, it’s invoked directly on the class, making the code more concise. This method enhances readability and maintains a clear connection between the resource and the associated class.
It is a preferred choice when the class itself is known and readily available. Overall, Class.getResourceAsStream()
simplifies file handling in the classpath
, providing an efficient and straightforward approach in Java applications.
Let’s embark on a practical journey by exploring a code snippet that showcases the application of Class.getResourceAsStream()
. Assume we have a text file named sample.txt
nestled in a directory named config
within our project structure.
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
public class FileReadFromClasspath {
public static void main(String[] args) {
// Using the Class's getResourceAsStream() method
InputStream inputStream = FileReadFromClasspath.class.getResourceAsStream("/config/sample.txt");
if (inputStream != null) {
try (BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
String line;
// Reading and printing each line of the file
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
} else {
System.out.println("File not found!");
}
}
}
The key to our approach is using FileReadFromClasspath.class.getResourceAsStream("/config/sample.txt")
, invoking the method directly on the class with a path relative to the classpath
. This method returns an InputStream
, serving as our access point to the file content.
To efficiently read line by line, we encapsulate the InputStream
in a BufferedReader
and use an InputStreamReader
for byte-to-character conversion. Within a try
block, a while
loop iterates through each line, printing it to the console.
Exception handling is managed by a try-with-resources
block, ensuring proper resource closure and handling any potential IOException
during file reading. This method streamlines file access, contributing to cleaner and more readable Java code.
Suppose the sample.txt
file contains the following content:
Hello! My name is Pillow and I am a Chihuahua!
The output of the program would be:
This method empowers developers to create more modular and resilient code, allowing resources to be seamlessly accessed relative to the classpath
. By incorporating this approach, your Java applications gain a level of flexibility that is invaluable, enabling smoother resource handling across different deployment scenarios.
Reading Files From Classpath
Using Paths
and Files
(Java 7 and Later)
The Paths
and Files
methods introduced in Java 7 offer a modern and robust approach to reading files from the classpath
. With streamlined APIs, they simplify file operations, reducing boilerplate code.
These methods, such as Paths.get()
and Files.readAllLines()
, enhance code readability and maintainability. Unlike traditional methods, they provide a cleaner way to handle file paths and operations, making Java applications more adaptable.
Particularly beneficial in modular projects, these methods ensure efficient and concise file access, contributing to the overall simplicity and effectiveness of Java programming in version 7 and later.
The code snippet below exemplifies how to use Paths
and Files
to read a file from the classpath
.
import java.io.IOException;
import java.net.URISyntaxException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.List;
public class FileReadFromClasspath {
public static void main(String[] args) throws URISyntaxException {
// Constructing the path to the file
Path filePath =
Paths.get(FileReadFromClasspath.class.getClassLoader().getResource("sample.txt").toURI());
try {
// Reading all lines from the file
List<String> lines = Files.readAllLines(filePath);
// Printing each line to the console
lines.forEach(System.out::println);
} catch (IOException | NullPointerException e) {
e.printStackTrace();
}
}
}
Our process begins by using FileReadFromClasspath.class.getClassLoader().getResource("sample.txt")
to obtain a URL pointing to the resource. We then construct a Path
instance from this URL using Paths.get(...)
, converting it into a file path.
The file content is read using the Files.readAllLines(filePath)
method, which returns a List<String>
with each line. To print each line to the console, a forEach
loop with System.out::println
is employed.
Exception handling is integrated into a try-catch
block, addressing potential IOExceptions
for file reading issues and guarding against a null URL with a NullPointerException
if the resource is not found. This method ensures a straightforward and robust approach to reading files from the classpath
in Java.
Assuming the sample.txt
file contains the following content:
Hello! My name is Pillow and I am a Chihuahua!
The output of the program would be:
This approach simplifies file handling by providing concise and powerful APIs. By employing Paths
and Files
, developers can seamlessly read file content from the classpath
, enhancing the readability and maintainability of their Java applications.
The example code demonstrates the elegance and effectiveness of this method, showcasing its utility in real-world scenarios.
Best Practices for Reading Files From Classpath
in Java
Use Class.getResourceAsStream()
Leverage Class.getResourceAsStream()
for direct access to resources. This method simplifies code and ensures a straightforward path relative to the class.
Handle IOExceptions
Implement proper exception handling, especially for IOExceptions
during file reading operations. Use try-with-resources
for resource management.
Utilize ClassLoader.getResourceAsStream()
When needed, use ClassLoader.getResourceAsStream()
. It’s effective when the class reference is not available, offering flexibility in resource access.
Verify Resource Existence
Check for null when obtaining URLs with getResource()
or getResourceAsStream()
. Ensure the resource exists before proceeding.
Consider Paths
and Files
(Java 7+)
For modern projects, explore the Paths
and Files
methods introduced in Java 7+. They offer concise and robust file handling capabilities.
Maintain Project Structure
Organize your project structure, placing resources in designated directories. This ensures a clear and consistent approach to resource access.
Handle Encoding Appropriately
When dealing with text files, be mindful of encoding. Specify the correct character set to avoid potential issues with file content interpretation.
Conclusion
Reading files from the classpath
in Java is vital for creating versatile and portable applications. The various methods, including getResource()
, Class.getResourceAsStream()
, ClassLoader.getResourceAsStream()
, and modern approaches like Paths
and Files
, offer flexibility in accessing resources.
Best practices; proper exception handling, maintaining project structure, and considering encoding, contribute to robust and maintainable code. By adhering to these methods and practices, developers ensure efficient file access, enhancing the adaptability and resilience of Java applications across different deployment scenarios.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook