Java의 클래스 경로에서 파일 읽기
- Java의 클래스 경로에서 파일 읽기
-
getResourceAsStream()
메서드를 사용하여 Java의 클래스 경로에서 파일 읽기 -
getResource()
메서드를 사용하여 Java의 클래스 경로에서 파일 읽기
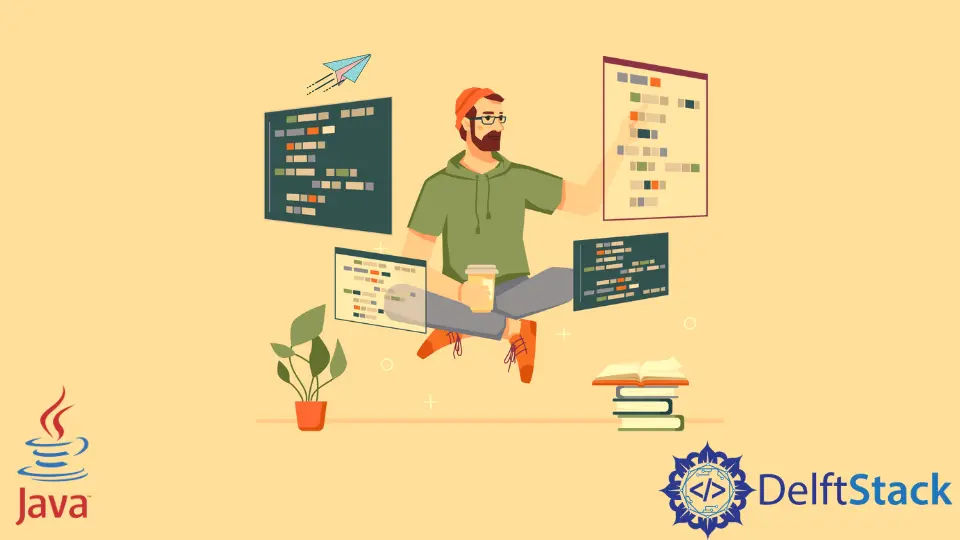
Java에서 클래스 경로에서 파일을 읽는 방법에는 두 가지가 있습니다. 리소스 폴더에 있는 파일을 inputstream
으로 로드하거나 URL 형식으로 로드한 다음 원하는 대로 할 수 있습니다.
이 자습서는 Java의 클래스 경로에서 파일을 읽는 방법을 보여줍니다.
Java의 클래스 경로에서 파일 읽기
위에서 언급했듯이 두 가지 방법이 있습니다. 하나는 obj.getClass().getClassLoader().getResourceAsStream()
이고 다른 하나는 obj.getClass().getClassLoader().getResource()
입니다.
이러한 메서드는 스트림이나 URL 또는 해당 파일을 가져온 다음 파일을 읽을 수 있습니다. 이러한 작업을 적용하기 전에 다음 두 가지 사항을 고려해야 합니다.
public
클래스의 객체를 선언해야 합니다.getClass()
메서드는 정적이 아니므로 호출할 객체가 필요합니다.- 클래스 경로는 예제가 실행되는 위치와 동일하므로 파일이 올바른 경로에 있어야 합니다.
먼저 getResourceAsStream()
메서드부터 시작하겠습니다.
getResourceAsStream()
메서드를 사용하여 Java의 클래스 경로에서 파일 읽기
package delftstack;
import java.io.*;
public class Read_Files {
public static void main(String[] args) throws Exception {
// creating object of the class for getClass method
Read_Files Class_Object = new Read_Files();
String Resource_File = "delftstack.txt";
System.out.println("Reading the file " + Resource_File + " from classpath");
InputStream Input_Stream =
Class_Object.getClass().getClassLoader().getResourceAsStream(Resource_File);
InputStreamReader Input_Stream_Reader = new InputStreamReader(Input_Stream);
BufferedReader reader = new BufferedReader(Input_Stream_Reader);
String Content;
while ((Content = reader.readLine()) != null) {
System.out.println(Content);
}
}
}
위의 코드는 getResourceAsStream
메서드를 사용하여 inputstream
을 가져온 다음 BufferedReader
의 도움으로 클래스 경로에서 파일을 읽습니다. 파일이 클래스 경로에 없으면 null 포인터 예외를 반환합니다. 출력 참조:
Reading the file delftstack.txt from classpath
Hello This is delftstack.com
The best online platform for learning different programming languages.
getResource()
메서드를 사용하여 Java의 클래스 경로에서 파일 읽기
package delftstack;
import java.io.*;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.util.List;
public class Read_Files {
public static void main(String[] args) throws Exception {
// creating object of the class for getClass method
Read_Files Class_Object = new Read_Files();
String Resource_File = "delftstack.txt";
System.out.println("Reading the file " + Resource_File + " from classpath");
URL Class_URL = Class_Object.getClass().getClassLoader().getResource(Resource_File);
File Class_File = new File(Class_URL.toURI());
List<String> Content;
Content = Files.readAllLines(Class_File.toPath(), StandardCharsets.UTF_8);
for (String Line : Content) {
System.out.println(Line);
}
}
}
위의 코드는 getResource()
메서드를 사용하여 클래스 경로에 대한 URL을 가져온 다음 URL의 도움으로 파일을 읽습니다. 출력 참조:
Reading the file delftstack.txt from classpath
Hello This is delftstack.com
The best online platform for learning different programming languages.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook