Java Out Parameter
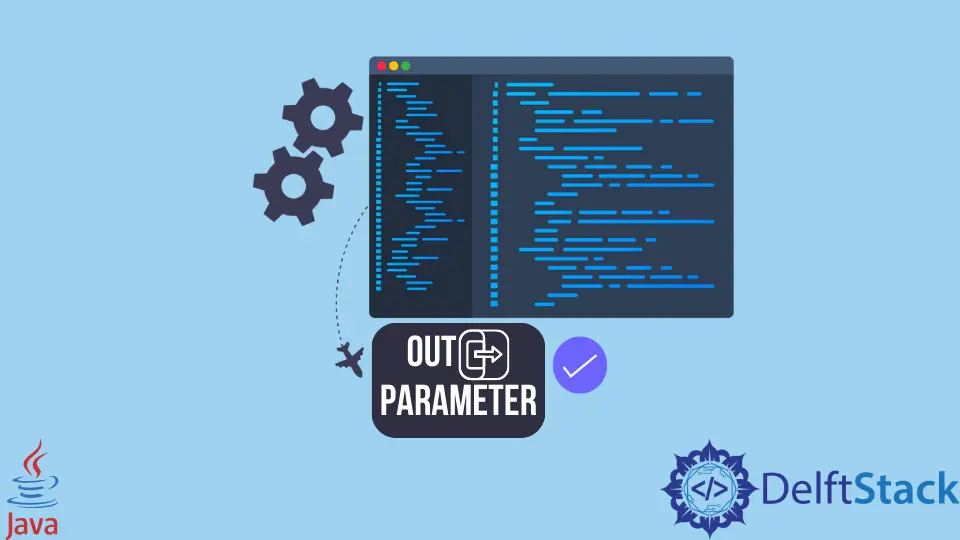
In programming languages like Java and C#, how method parameters are handled can be quite distinctive.
In Java, parameters are passed by value, even when dealing with object references, which sets it apart from C#. In C#, you have the convenience of keywords like out
and ref
for passing parameters by reference, facilitating multiple value returns from methods.
However, Java takes a different approach, lacking these keywords. To achieve similar outcomes in Java, you’ll learn how to encapsulate parameters within objects and pass references to these objects as method arguments.
This tutorial sheds light on this technique, offering insights into how to manipulate variables indirectly within Java methods and extend the impact of these changes beyond their immediate scope.
the Out
Parameter in Java
In C#, the out
parameter allows a method to return multiple values. However, Java does not support this feature natively.
As mentioned above, in Java, parameters are always passed by value, meaning the values of the variables are passed to the method, not the actual variables themselves.
Suppose we have the following C# code example with the out
parameter:
using System;
class Out_Parameter {
static void Divide(int x, int y, out int divide_result, out int divide_remainder) {
divide_result = x / y;
divide_remainder = x % y;
}
static void Main() {
for (int x = 1; x < 5; x++)
for (int y = 1; y < 5; y++) {
int result, remainder;
Divide(x, y, out result, out remainder);
Console.WriteLine("{0} / {1} = {2}r{3}", x, y, result, remainder);
}
}
}
Output:
1 / 1 = 1r0
1 / 2 = 0r1
1 / 3 = 0r1
1 / 4 = 0r1
2 / 1 = 2r0
2 / 2 = 1r0
2 / 3 = 0r2
2 / 4 = 0r2
3 / 1 = 3r0
3 / 2 = 1r1
3 / 3 = 1r0
3 / 4 = 0r3
4 / 1 = 4r0
4 / 2 = 2r0
4 / 3 = 1r1
4 / 4 = 1r0
To achieve similar functionality in Java, we can use the approach of wrapping the parameters inside an object or using an array to hold the values we want to modify and return.
Let’s have an example to see how this can be done:
First, we create a simple wrapper class that holds the values we want to modify. In this case, we’ll create a Result
class to hold the division result
and remainder
.
class Result {
int result;
int remainder;
public Result(int result, int remainder) {
this.result = result;
this.remainder = remainder;
}
}
Next, we modify the divide
method to accept an instance of the Result
class instead of primitive int
variables.
static void divide(int x, int y, Result result) {
result.result = x / y;
result.remainder = x % y;
System.out.println(x + "/" + y + " = " + result.result + " r " + result.remainder);
}
In the main
method, we create an instance of the Result
class, pass it to the divide
method, and then retrieve the division result and remainder from the Result
instance.
public static void main(String[] args) {
for (int x = 1; x < 5; x++) {
for (int y = 1; y < 5; y++) {
Result result = new Result(0, 0);
divide(x, y, result);
}
}
}
By following these steps and utilizing the Result
class as a wrapper to hold the result and remainder values, we achieve a similar functionality to the out
parameter in C# in Java.
Complete example code:
package delftstack;
public class Out_Parameter {
static void divide(int x, int y, int divide_result, int divide_remainder) {
divide_result = x / y;
divide_remainder = x % y;
System.out.println(x + "/" + y + " = " + divide_result + " r " + divide_remainder);
}
public static void main(String[] args) {
for (int x = 1; x < 5; x++)
for (int y = 1; y < 5; y++) {
int result = 0, remainder = 0;
divide(x, y, result, remainder);
}
}
}