Java 输出参数
Sheeraz Gul
2023年10月12日
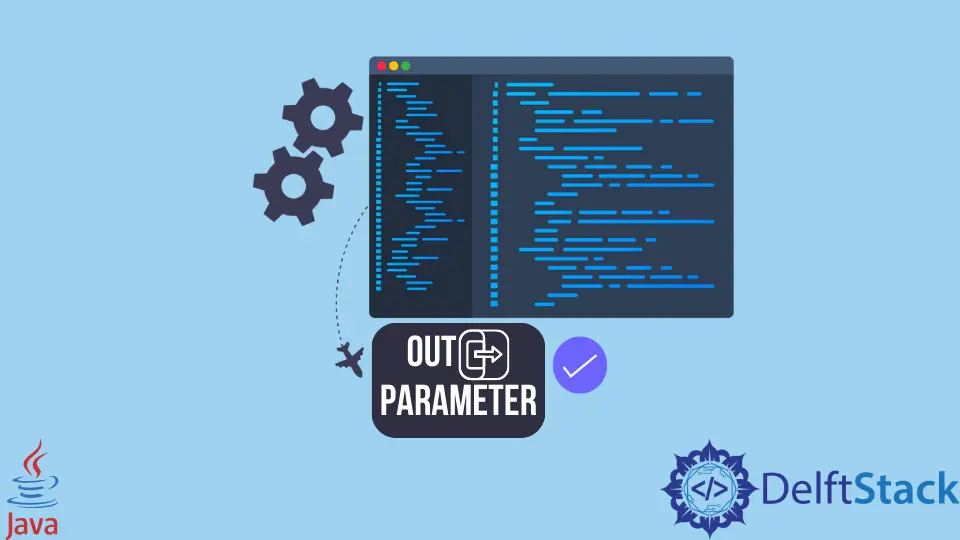
Java 不支持像 C# 中的 out
和 ref
之类的关键字在方法中通过引用传递,因为值仅在 Java 中传递参数。该值甚至通过引用。
为了在 Java 中实现类似 C# 的 out
和 ref
以通过引用传递,我们必须将参数包装在对象中并将该对象引用作为参数传递。本教程演示了如何在 Java 中实现与 C# 的 out
参数相同的输出。
Java 中的 Out
参数
如上所述,Java 不支持 out
参数。我们可以通过将原语包装到类或使用数组来保存多个返回值来实现此 C# 功能。我们可以通过引用传递来回调该值。
看一个例子;首先,带有 out
关键字的 C# 程序和 Java 程序通过简单地按值传递来执行相同的操作。
using System;
class Out_Parameter {
static void Divide(int x, int y, out int divide_result, out int divide_remainder) {
divide_result = x / y;
divide_remainder = x % y;
}
static void Main() {
for (int x = 1; x < 5; x++)
for (int y = 1; y < 5; y++) {
int result, remainder;
Divide(x, y, out result, out remainder);
Console.WriteLine("{0} / {1} = {2}r{3}", x, y, result, remainder);
}
}
}
上面的 C# 程序使用 out
参数来计算除法和余数。
见输出:
1 / 1 = 1r0
1 / 2 = 0r1
1 / 3 = 0r1
1 / 4 = 0r1
2 / 1 = 2r0
2 / 2 = 1r0
2 / 3 = 0r2
2 / 4 = 0r2
3 / 1 = 3r0
3 / 2 = 1r1
3 / 3 = 1r0
3 / 4 = 0r3
4 / 1 = 4r0
4 / 2 = 2r0
4 / 3 = 1r1
4 / 4 = 1r0
现在让我们尝试通过按值传递参数来在 Java 中实现相同的 out
参数功能。
package delftstack;
public class Out_Parameter {
static void divide(int x, int y, int divide_result, int divide_remainder) {
divide_result = x / y;
divide_remainder = x % y;
System.out.println(x + "/" + y + " = " + divide_result + " r " + divide_remainder);
}
public static void main(String[] args) {
for (int x = 1; x < 5; x++)
for (int y = 1; y < 5; y++) {
int result = 0, remainder = 0;
divide(x, y, result, remainder);
}
}
}
上面的代码将给出与 C# out
相同的输出。
1/1 = 1 r 0
1/2 = 0 r 1
1/3 = 0 r 1
1/4 = 0 r 1
2/1 = 2 r 0
2/2 = 1 r 0
2/3 = 0 r 2
2/4 = 0 r 2
3/1 = 3 r 0
3/2 = 1 r 1
3/3 = 1 r 0
3/4 = 0 r 3
4/1 = 4 r 0
4/2 = 2 r 0
4/3 = 1 r 1
4/4 = 1 r 0
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook