How to Set Java Logger Levels
- Logger Levels in Java
- Logger Levels Working Mechanism
- Logger Levels Configuration
- Logger Levels Example in Java
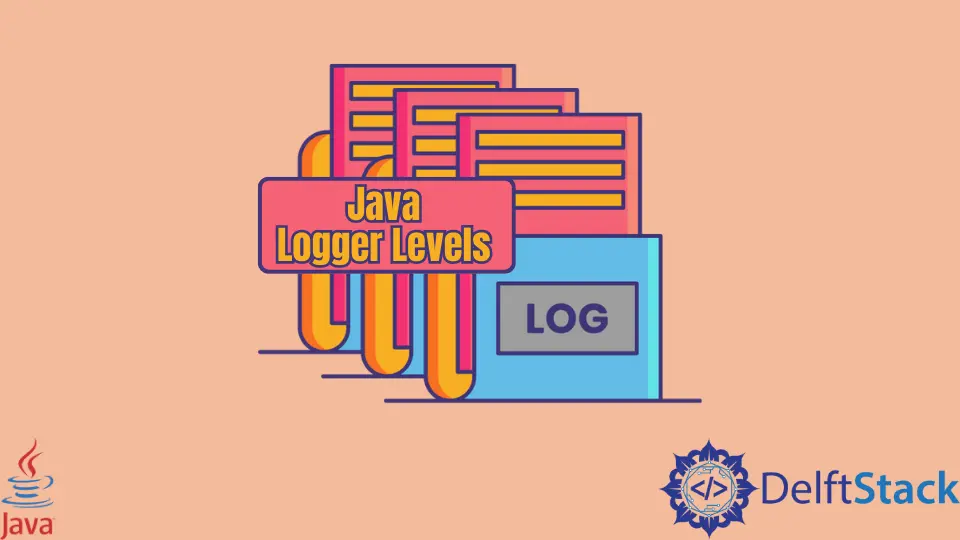
Logger is used for recording some text to some destination in Java, and the logging levels are the labels that can be attached to each log entry which shows their severity.
This tutorial demonstrates how to use logger levels in Java.
Logger Levels in Java
Log4j
API is used to implement the logger levels in Java. The logging level is employed to categorize the entries in the log file.
The categorization is done in a specific way and by urgency. With Logger Level, we can filter the log files during the search and manage the amount of information we logged.
The type and amount of information given in the event logs and system can be controlled by the log4j
level setting in the config file. The level of the message labels every log message.
In Java, Logging levels are the instances of the org.apache.log4j.Level
class. The topic below describes each logger level used in Java.
Logger Level | Description |
---|---|
ALL |
This includes all levels, including the custom levels. |
DEBUG |
Useful to debug an application; this level designates fine-grained informational events. |
INFO |
Used for informational messages that highlight the application’s progress at the coarse level. |
WARN |
Used for potentially harmful situations. |
ERROR |
Used for the errors, which can still allow the application to continue running. |
FATAL |
Used for the errors which can stop the application from running, which means to abort the application. |
OFF |
Used for the highest possible rank and intended to turn off the logging. |
TRACE |
Used for fine-grained informational events compared to the DEBUG . |
Logger Levels Working Mechanism
The Log Levels work very simply. The application code creates logging requests during the runtime; each will have a level.
At the same time, the logging framework will have a log level configured which will be used as a threshold. If the request level is configured or higher, it will be logged to the configured target.
And if it doesn’t configure, that means it is denied. The log levels are considered in the following rank order.
ALL < TRACE < DEBUG < INFO < WARN < ERROR < FATAL < OFF
Logger Levels Configuration
Log4j
provides a configuration file-based level setting that allows us to change the source code based on the change in debugging level.
The following setting is the standard approach written to the log4j.properties file and the path of this file should be the classpath.
# Set root logger level to DEBUG, and its only appender to A1.
log4j.rootLogger=DEBUG, A1
# A1 is set to be a ConsoleAppender.
log4j.appender.A1=org.apache.log4j.ConsoleAppender
# A1 uses PatternLayout.
log4j.appender.A1.layout=org.apache.log4j.PatternLayout
log4j.appender.A1.layout.ConversionPattern=%-4r [%t] %-5p %c %x - %m%n
Logger Levels Example in Java
Let’s try an example in Java to show Logger Level using log4j
.
package delftstack;
import org.apache.log4j.*;
public class Logger_Level {
private static org.apache.log4j.Logger log_Level = Logger.getLogger(Logger_Level.class);
public static void main(String[] args) {
log_Level.trace("This is Trace Message!");
log_Level.debug("This is Debug Message!");
log_Level.info("This is Info Message!");
log_Level.warn("This is Warn Message!");
log_Level.error("This is Error Message!");
log_Level.fatal("This is Fatal Message!");
}
}
The code will run all the logger levels described and generate the result.
0 [main] DEBUG delftstack.Logger_Level - This is Debug Message!
2 [main] INFO delftstack.Logger_Level - This is Info Message!
2 [main] WARN delftstack.Logger_Level - This is Warn Message!
2 [main] ERROR delftstack.Logger_Level - This is Error Message!
2 [main] FATAL delftstack.Logger_Level - This is Fatal Message!
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook