How to Use a Logger in Java
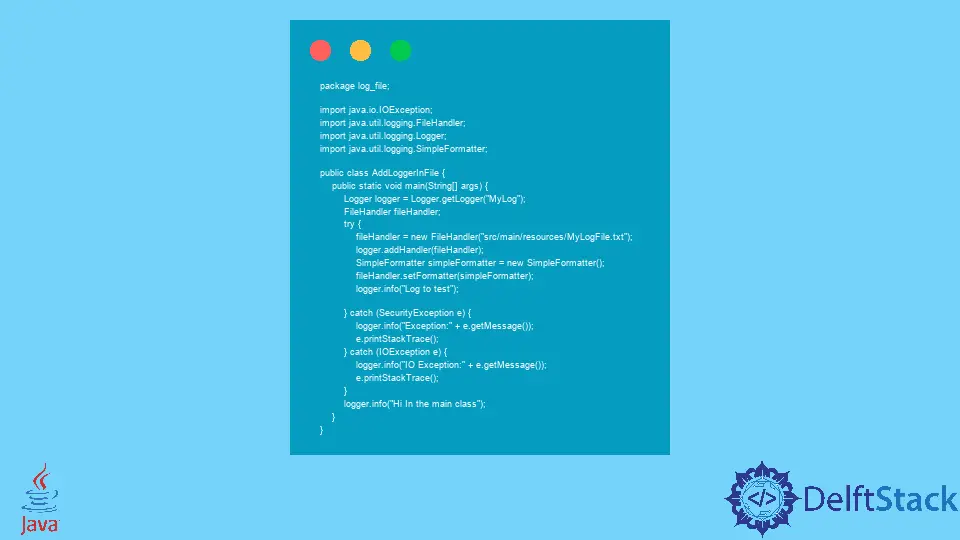
In the Java programming language, Logging
is an API that allows users to trace out the error generated from the specific classes. A logger helps in the real-time logging mechanism by adding timestamps and the endpoints where it gets populated. The API helps in maintaining the track of events as a record of a log. The records help locate the root cause of the issues that happened and regenerate the same event later.
This article shows you a method to utilize the Java logs better.
the Functions of a Logger in Java
The logger and its function are demonstrated in the program below.
package log_file;
import java.io.IOException;
import java.util.logging.FileHandler;
import java.util.logging.Logger;
import java.util.logging.SimpleFormatter;
public class AddLoggerInFile {
public static void main(String[] args) {
Logger logger = Logger.getLogger("MyLog");
FileHandler fileHandler;
try {
fileHandler = new FileHandler("src/main/resources/MyLogFile.txt");
logger.addHandler(fileHandler);
SimpleFormatter simpleFormatter = new SimpleFormatter();
fileHandler.setFormatter(simpleFormatter);
logger.info("Log to test");
} catch (SecurityException e) {
logger.info("Exception:" + e.getMessage());
e.printStackTrace();
} catch (IOException e) {
logger.info("IO Exception:" + e.getMessage());
e.printStackTrace();
}
logger.info("Hi In the main class");
}
}
In the code block above, a logger gets instantiated initially.
The class is present in the java.util.logging
package. The getLogger
is a static method present in the Logger
class that creates a logger if it’s not present in the system with the given name. The function else
returns the existing logger instance if it’s already present.
The function throws NullPointerException
if the name given is the null value. Once the logger instance gets instantiated, a file handler instance gets created.
The FileHandler
class is present in the java.util.logging
package and gets specifically designed and configured to handle the log printed using the logger
instance. The constructor FileHandler
initializes the handler to write the information to the given file name. The constructor takes the name of the output file as a parameter.
In the code above, a relative path gets provided with the file name. The size is not defined in the file, as it can get extended to the limit the logs get into it. The constructor throws IOException
when the compiler faces a problem opening the files. It throws SecurityException
if a security manager does not have LoggingPermission
, and IllegalArgumentException
if the pattern is an empty string.
The addHandler
method maps a file handler to receive the logging messages into the file. The function is of the void
return type, as it does not return any value. Instead, it consumes the logs and events only. It throws SecurityException
if a security manager does not have enough Logging Permissions.
The SimpleFormatter
instance gets initiated; the class is also present in the java.util.logging
package. The formatter allows the privilege of autoformatting the logs using the logging.SimpleFormatter.format
property. This property allows the log messages to be in a defined format and hence, maintaining uniformity.
The setFormatter
is a function that sets a formatter on the logger instance. The info
is a function present in the Logger
class to print the INFO
log messages into the file. There can be various log levels present in the Level class. Based on the use, we can use INFO
, DEBUG
, FINE
, CONFIG
, and SEVERE
logs.
The log levels
are the logging details that define the program log severity. The levels have some defined number and a specified meaning. For example, SEVERE
has a log value of 1000
, and it denotes the failure in the system and must be taken care of on an immediate basis.
Severity occurs when some network failure or database connectivity loses. Similarly, the WARNING
Level holds a value of 900
and denotes the potential risk in the system.
Below is the output that gets populated in the standard console output and the MyLogFile.txt
file.
Output:
Jul 04, 2021 12:05:29 AM log_file.AddLoggerInFile main
INFO: Log to test
Jul 04, 2021 12:05:29 AM log_file.AddLoggerInFile main
INFO: Hi In the main class
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn