How to Request HTTP Client and Get Response in Java
- Send an HTTP Request and Receive a JSON Response From Client in Java
- Execute HTTP Request and Get Response Asynchronously in Java
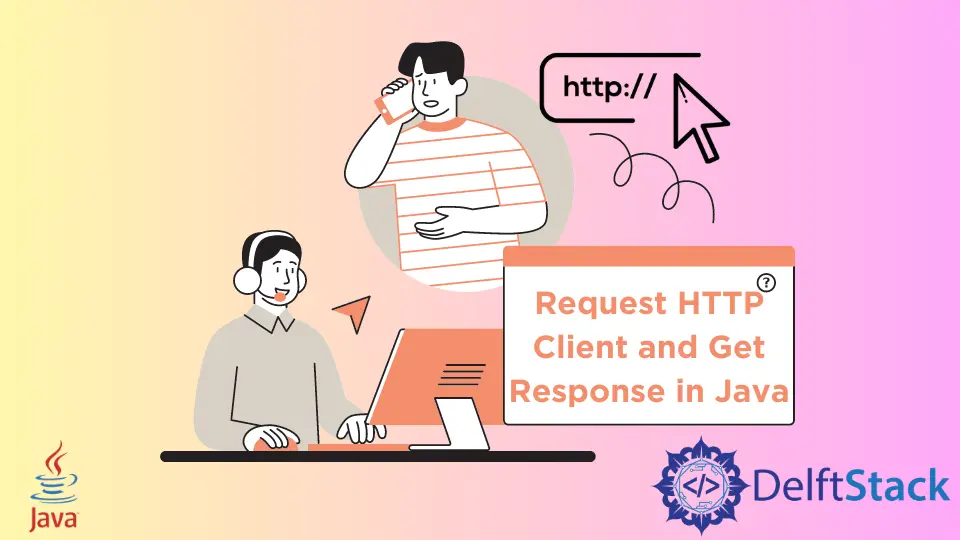
We will use an HTTP Client in Java to send requests and receive responses. Meanwhile, you will also learn how to use the body handlers, builder, and other underlying methods to send an HTTP Client request.
Send an HTTP Request and Receive a JSON Response From Client in Java
We will use a demo JSON website that programmers use to evaluate their HTTP requests. Here it is https://blog.typicode.com/
.
Before that, please make notes of the following methods that will help you along.
HttpClient send2client = HttpClient.newHttpClient();
- We use it to send http requests to clients and receive responses.HttpRequest Req2client = HttpRequest();
- This method helps build http request as an instance with its following parameters.
2.1.newBuilder();
- Generates an Http Request builder. This method returns a builder that builds the standard HTTP Client API objects.
2.2.uri("The Client URL");
- Sets http request’s URL.
2.3.build();
- This parameter builds and returns http request.HttpResponse<String> clientRes = send2client.send(Req2client, HttpResponse.BodyHandlers.ofString());
- The response status code, headers, response body, and the HTTP Request corresponding to this response are all accessible through this class.
Check out the following program that performs everything that we discussed so far.
import java.io.IOException;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class Example1 {
public static void main(String[] args) throws IOException, InterruptedException {
HttpClient send2client = HttpClient.newHttpClient();
HttpRequest Req2client =
HttpRequest.newBuilder().uri(URI.create("https://blog.typicode.com/")).build();
String format = System.getProperty("line.separator");
HttpResponse<String> clientRes =
send2client.send(Req2client, HttpResponse.BodyHandlers.ofString());
System.out.println(" Requested Responses from the client" + format + "1: Status code" + format
+ clientRes.statusCode() + format);
System.out.println(
"2: Uniform Resource Locator (URL) from the client" + clientRes.uri() + format);
System.out.println("3: SSL Session" + format + clientRes.sslSession() + format);
System.out.println("4: HTTP version" + format + clientRes.version() + format);
// System.out.println("5: Response Header" + format + clientRes.headers() + format);
// System.out.println("6: Response Body" + format + clientRes.body() + format);
}
}
Output:
Requested Responses from the client
1: Status code
200
2: Uniform Resource Locator (URL) from the client: https://blog.typicode.com/
3: SSL Session
Optional[jdk.internal.net.http.common.ImmutableExtendedSSLSession@5bcea91b]
4: HTTP version
HTTP_2
Execute HTTP Request and Get Response Asynchronously in Java
We will use the same HttpRequest
method in the following code block but with the following functions.
sendAsync()
- This client sends the specified request asynchronously with the specified response body handlers.
The sendAsync()
and HttpRequest
are sending and retrieving methods. Both are secure for HTTP web handlers.
Syntax:
Cli.sendAsync(RQI, BodyHandlers.ofString())
.thenApply(HttpResponse::body) // optional
.thenAccept(
System.out::println) // The action to take before completing the retrieved completion stage
.join(); // returts the response value
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.net.http.HttpResponse.BodyHandlers;
public class Example2 {
public static void main(String[] args) {
HttpClient Cli = HttpClient.newHttpClient();
HttpRequest RQI =
HttpRequest.newBuilder().uri(URI.create("https://www.delftstack.com")).build();
Cli.sendAsync(RQI, BodyHandlers.ofString())
.thenApply(HttpResponse::body)
.thenAccept(System.out::println)
.join();
}
}
Output:
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn