How to Implement HTTP Post in Java
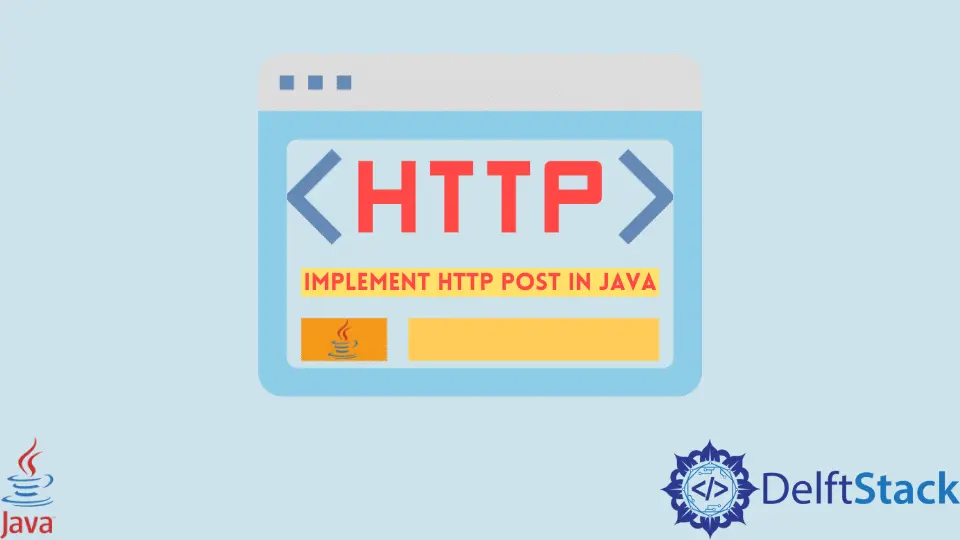
In this tutorial, we will discuss how to send an HTTP POST
request using different methods in Java. There are a few request methods of HTTP, and POST
is one of them.
The POST
is used to send some data to the server. We will use the website https://postman-echo.com
to test our dummy data for the examples.
HTTP Post Using HttpURLConnection
in Java
The first example uses the HttpURLConnection
class that extends the URLConnection
abstract class with various functions to perform network operations.
In the program, we create an object dummyUrl
of URL
and pass the endpoint we use, https://postman-echo.com/post
. When we send a post request, we send some data with it.
The dummyData
holds the data with the firstname
and lastname
as a key and john
and doe
as their values with both the key-value pairs appended with a &
symbol.
Now, we call the openConnection()
method of the URL
class that returns an URLConnection
object which we can cast and hold as an object of the HttpURLConnection
type.
To set the request
method, we call the setRequestMethod()
function and pass the request type. If we want to send the data to the API, we need to enable the doOutput
field to enable output connection.
The next step is to set the request properties using the setRequestProperty()
function that accepts a key and a value as its arguments.
The first request property is the Content-Type that specifies the type of content we want to send, the second property tells the charset to use, and the last property tells the Content-Length of the post data.
To get the response data we need to get the output stream from the httpUrlConnection
using getOutputStream()
function and pass it as an argument for DataOutputStream
constructor.
That returns a DataOutputStream
object. We write the data of dataOutputStream
using the writeBytes()
method.
Next, we get the input stream using the getInputStream()
function and use it in the InputStreamReader()
to get the BufferedReader
object.
To read the data from the stream, we create a loop and read every line from the BufferedReader
and output it on the console.
import java.io.*;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
class ExampleClass1 {
public static void main(String[] args) throws MalformedURLException {
URL dummyUrl = new URL("https://postman-echo.com/post");
String dummyData = "firstname=john&lastname=doe";
try {
HttpURLConnection httpUrlConnection = (HttpURLConnection) dummyUrl.openConnection();
httpUrlConnection.setRequestMethod("POST");
httpUrlConnection.setDoOutput(true);
httpUrlConnection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
httpUrlConnection.setRequestProperty("charset", "utf-8");
httpUrlConnection.setRequestProperty("Content-Length", Integer.toString(dummyData.length()));
DataOutputStream dataOutputStream = new DataOutputStream(httpUrlConnection.getOutputStream());
dataOutputStream.writeBytes(dummyData);
InputStream inputStream = httpUrlConnection.getInputStream();
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream));
String stringLine;
while ((stringLine = bufferedReader.readLine()) != null) {
System.out.println(stringLine);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
{"args":{},"data":"","files":{},"form":{"firstname":"john","lastname":"doe"},"headers":{"x-forwarded-proto":"https","x-forwarded-port":"443","host":"postman-echo.com","x-amzn-trace-id":"Root=1-6204df8b-078f0e863f06000030e99563","content-length":"27","content-type":"application/x-www-form-urlencoded","charset":"utf-8","user-agent":"Java/15.0.1","accept":"text/html, image/gif, image/jpeg, *; q=.2, */*; q=.2"},"json":{"firstname":"john","lastname":"doe"},"url":"https://postman-echo.com/post"}
HTTP Post Using Apache HttpClient
in Java
In this section of the tutorial, we will use an external library to send an HTTP post request. We import the Apache library using the below maven dependency.
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
The HttpClient
is a collection of methods that helps in performing HTTP operations.
In the following code, we call createDefault()
method of the HttpClients
to build create HttpClientBuilder
that returns an object of CloseableHttpClient
.
We need to set the URI and the parameters to send with the post request. To do this, we call RequestBuilder.post()
and set the URI using setURI()
.
Then two parameters use the addParameter()
function that takes the key and the value.
We call the execute()
function using the closeableHttpClient
object and pass the httpUriRequest
as its argument which returns a CloseableHttpResponse
object.
Now we call the getEntity()
function to get the HttpEntity
from the closeableHttpClientResponse
and convert it to string using EntityUtils.toString()
.
Next, we print the response from the closeableHttpClientResponse
.
import java.io.*;
import java.net.*;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpUriRequest;
import org.apache.http.client.methods.RequestBuilder;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
class ExampleClass1 {
public static void main(String[] args) {
CloseableHttpClient closeableHttpClient = HttpClients.createDefault();
try {
URI dummyUri = new URI("https://postman-echo.com/post");
HttpUriRequest httpUriRequest = RequestBuilder.post()
.setUri(dummyUri)
.addParameter("firstname", "Dwayne")
.addParameter("lastname", "Johnson")
.build();
CloseableHttpResponse closeableHttpClientResponse =
closeableHttpClient.execute(httpUriRequest);
String getResponse = EntityUtils.toString(closeableHttpClientResponse.getEntity());
System.out.println(getResponse);
} catch (IOException | URISyntaxException e) {
e.printStackTrace();
} finally {
try {
closeableHttpClient.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Output:
{"args":{},"data":"","files":{},"form":{"firstname":"Dwayne","lastname":"Johnson"},"headers":{"x-forwarded-proto":"https","x-forwarded-port":"443","host":"postman-echo.com","x-amzn-trace-id":"Root=1-6204eaa0-7444d40757db202e2815744d","content-length":"33","content-type":"application/x-www-form-urlencoded; charset=UTF-8","user-agent":"Apache-HttpClient/4.5.13 (Java/15.0.1)","accept-encoding":"gzip,deflate"},"json":{"firstname":"Dwayne","lastname":"Johnson"},"url":"https://postman-echo.com/post"}
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn