How to Send HTTP Requests in Java
- Use HttpURLConnection to Send HTTP Requests in Java
- Use Apache HttpClient to Send HTTP Requests in Java
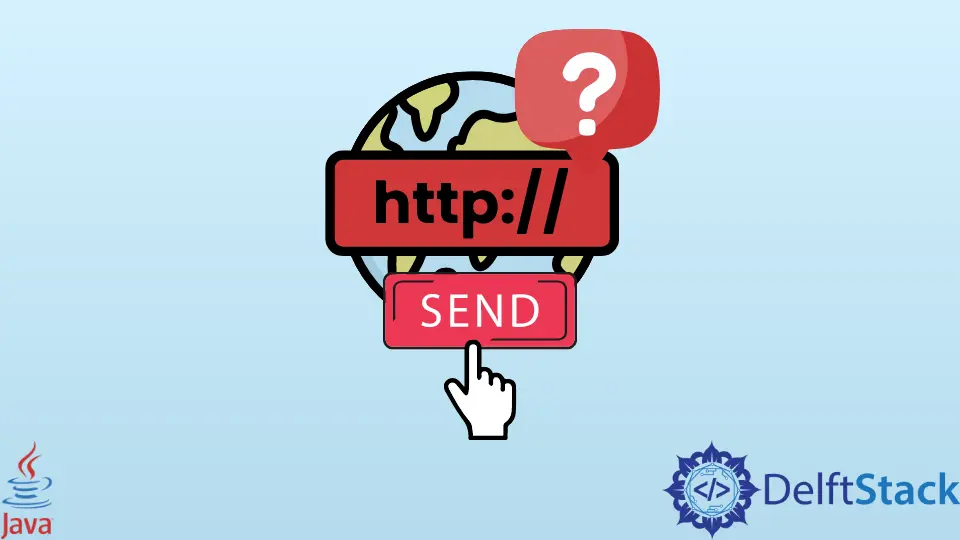
We can send HTTP requests in Java using Java HttpURLConnection, and Apache HttpClient.
This tutorial will demonstrate how to send an HTTP request using the two methods in Java.
Use HttpURLConnection to Send HTTP Requests in Java
HttpURLConnection is a built-in method from the Java.net library. HTTP Requests are of two types, GET and POST.
Let’s try to send both using HttpURLConnection.
Use HttpURLConnection to Send GET HTTP Requests in Java
Let’s try to get the content of our webpage using the GET method HttpURLConnection method.
Example:
package delftstack;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class Get_HttpURLConnection {
private static HttpURLConnection get_connection;
public static void main(String[] args) throws IOException {
String url = "https://www.delftstack.com/";
try {
// We retrieve the contents of our webpage.
URL myurl = new URL(url);
get_connection = (HttpURLConnection) myurl.openConnection();
// Here we specify the connection type
get_connection.setRequestMethod("GET");
StringBuilder webpage_content;
try (BufferedReader webpage =
new BufferedReader(new InputStreamReader(get_connection.getInputStream()))) {
String webpage_line;
webpage_content = new StringBuilder();
while ((webpage_line = webpage.readLine()) != null) {
webpage_content.append(webpage_line);
webpage_content.append(System.lineSeparator());
}
}
System.out.println(webpage_content.toString());
} finally {
// Disconnect the connection
get_connection.disconnect();
}
}
}
The code above will output a large amount of content from https://www.delftstack.com/.
Output:
Use HttpURLConnection to Send POST HTTP Requests in Java
To send HTTP requests by POST method using HttpURLConnection, we must set the request properties. The example below demonstrates sending an HTTP request by HttpURLConnection and POST method, requiring a URL and the URL parameters.
package delftstack;
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.charset.StandardCharsets;
public class POST_HttpURLConnection {
private static HttpURLConnection post_connection;
public static void main(String[] args) throws IOException {
// URL
String Post_URL = "https://httpbin.org/post";
// URL Parameters
String Post_URL_Parameters = "name=Michelle&occupation=Secretory";
// Get Bytes of parameters
byte[] Post_Data = Post_URL_Parameters.getBytes(StandardCharsets.UTF_8);
try {
URL Demo_URL = new URL(Post_URL);
post_connection = (HttpURLConnection) Demo_URL.openConnection();
post_connection.setDoOutput(true);
// Set the method
post_connection.setRequestMethod("POST");
// The request property
post_connection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
// write the bytes or the data to the URL connection.
try (DataOutputStream Data_Output_Stream =
new DataOutputStream(post_connection.getOutputStream())) {
Data_Output_Stream.write(Post_Data);
}
// Get the content
StringBuilder Post_Webpage_Content;
try (BufferedReader webpage =
new BufferedReader(new InputStreamReader(post_connection.getInputStream()))) {
String Webpage_line;
Post_Webpage_Content = new StringBuilder();
while ((Webpage_line = webpage.readLine()) != null) {
Post_Webpage_Content.append(Webpage_line);
Post_Webpage_Content.append(System.lineSeparator());
}
}
System.out.println(Post_Webpage_Content.toString());
} finally {
post_connection.disconnect();
}
}
}
Output:
{
"args": {},
"data": "",
"files": {},
"form": {
"name": "Michelle",
"occupation": "Secretory"
},
"headers": {
"Accept": "text/html, image/gif, image/jpeg, *; q=.2, */*; q=.2",
"Content-Length": "34",
"Content-Type": "application/x-www-form-urlencoded",
"Host": "httpbin.org",
"User-Agent": "Java/17.0.2",
"X-Amzn-Trace-Id": "Root=1-62308f8e-7aa124b15d6d3cd1114d6463"
},
"json": null,
"origin": "182.182.126.98",
"url": "https://httpbin.org/post"
}
Use Apache HttpClient to Send HTTP Requests in Java
To use Apache HttpClient first, you must add the library to your project download Apache HttpClient and add the jar files to your project build path libraries.
Use Apache HttpClient to Send GET HTTP Requests in Java
We can also use the Apache HttpClient and get the content by sending HTTP requests through the GET method. Apache HttpClient is easier than HttpURLConnection.
Example:
package delftstack;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
public class Get_HttpClient {
public static void main(String[] args) throws IOException {
try (CloseableHttpClient Apache_Get_Client = HttpClientBuilder.create().build()) {
// Create a GET request
HttpGet Apache_Get_Request = new HttpGet("https://www.delftstack.com/");
// execute the request to get the response
HttpResponse Apache_Get_Response = Apache_Get_Client.execute(Apache_Get_Request);
// Get the content
BufferedReader Webpage =
new BufferedReader(new InputStreamReader(Apache_Get_Response.getEntity().getContent()));
StringBuilder Webpage_Content = new StringBuilder();
String Webpage_Content_Line;
while ((Webpage_Content_Line = Webpage.readLine()) != null) {
Webpage_Content.append(Webpage_Content_Line);
Webpage_Content.append(System.lineSeparator());
}
System.out.println(Webpage_Content);
}
}
}
Output:
Use Apache HttpClient to Send POST HTTP Requests in Java
We can use Apache HttpClient and get the content by sending an HTTP request through the POST method.
Example:
package delftstack;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
public class Post_HttpClient {
public static void main(String[] args) throws IOException {
try (CloseableHttpClient Apache_Post_Client = HttpClientBuilder.create().build()) {
// Create a post request
HttpPost Apache_Post_Request = new HttpPost("https://www.delftstack.com/");
// pass parameters or set entity
Apache_Post_Request.setEntity(new StringEntity("This is delftstack"));
// execute the request to get the response
HttpResponse Apache_Post_Response = Apache_Post_Client.execute(Apache_Post_Request);
// Get the content
BufferedReader Webpage =
new BufferedReader(new InputStreamReader(Apache_Post_Response.getEntity().getContent()));
StringBuilder Webpage_Content = new StringBuilder();
String Webpage_line;
while ((Webpage_line = Webpage.readLine()) != null) {
Webpage_Content.append(Webpage_line);
Webpage_Content.append(System.lineSeparator());
}
System.out.println(Webpage_Content);
}
}
}
Output:
Java 11 and the versions before had the built-in functionality of HttpClient, which is not supported in the newer versions. That HttpClient was different from Apache HTTPClient.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook