How to Capture and Analyze Java Heap Dump
- Introduction to Heap Dump and Its Formats
-
Example Code Causing
OutOfMemoryError
in Java - Different Ways to Capture Heap Dump
- Analyze Java Heap Dump
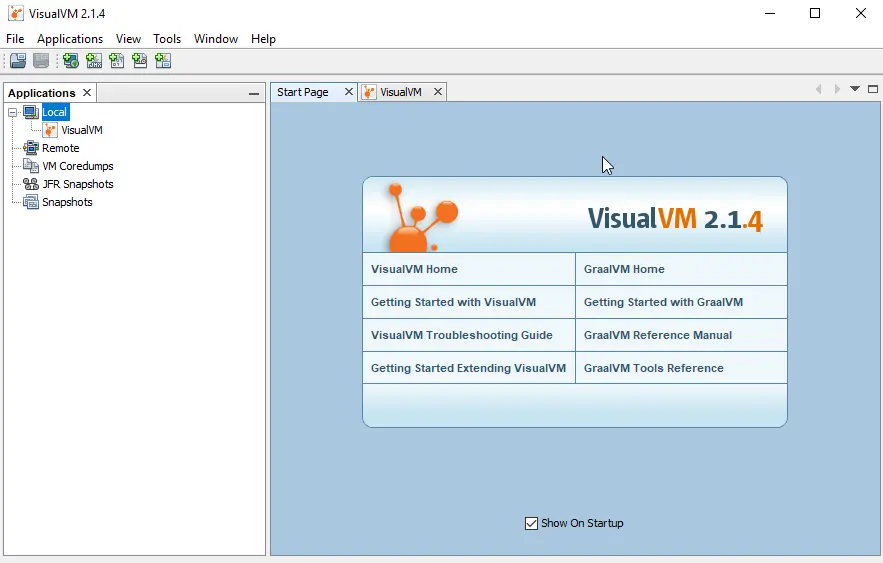
Heap dumps contain a snapshot of all live objects the running Java application uses on a Java heap.
This tutorial educates about heap dump, its various formats, and its importance.
Further, we will go through an example demonstrating OutOfMemoryError
, which will lead to various approaches to capture heap dump and a tool to analyze it.
Introduction to Heap Dump and Its Formats
A heap contains all the objects that we create by instantiating a class. Every class of Java runtime is also created in this heap.
This heap is created when JVM (Java Virtual Machine) starts and can expand/shrink during runtime to adjust objects destroyed or created in an application.
The garbage collection process runs whenever a heap gets full, this process collects all the objects that are no longer used, or we can say not referenced anymore (you can find more on memory manage here. Usually, heap dumps are stored in the binary format hprof
files.
We can retrieve detailed information about each object instance like type, class name, address, size and whether an instance contains references to another object(s) or not. The heap dumps can be in one of the following two formats:
- The Portable Heap Dump Format (also known as PHD format)
- The Classic Format
Remember that the portable heap dump is the default format and is in binary which must be processed for further analysis. On the other hand, the classic format is in ASCII text which is human-readable.
You may read about these two formats here.
Importance of Using Heap Dump
Usually, we get the advantage of heap dump when an application is crashed due to OutOfMemoryError
or a Java application consuming more memory than expected.
Heap dump helps us to identify the primary causes for the error and other details, for instance, the number of objects in every class, memory usage for every class, etc.
It also assists in capturing the memory size occupied by each Java object of an application. All this captured information can be useful for finding an actual code causing memory leak issues.
Let’s look at a code example causing OutOfMemoryError
, which will lead to various ways to capture Java heap dump.
Example Code Causing OutOfMemoryError
in Java
import java.util.ArrayList;
import java.util.List;
public class Test {
public static void main(String[] args) {
List<byte[]> myList = new ArrayList<>();
int index = 0;
while (true) {
// 1MB each iteration, 1 x 1024 x 1024 = 1048576
byte[] bytes = new byte[1048576];
myList.add(bytes);
Runtime runTime = Runtime.getRuntime();
System.out.printf("[%d] free memory is: %s%n", index++, runTime.freeMemory());
}
}
}