How to Increase Heap Space in Java
- Increase Java Heap Size Using Command Line
- Increase Java Heap Size in an Integrated Development Environment (IDE)
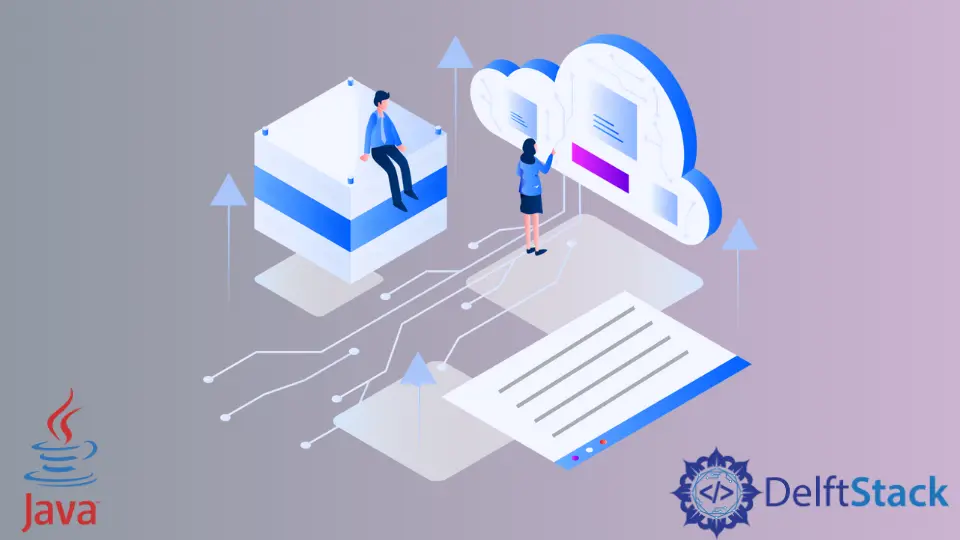
In Java, Heap Space is mainly used for garbage collection and allocating memory to objects.
A default Heap space is allocated when installing the JVM on our machine, but it can vary. The following points show how we can increase the heap size using two ways in Java.
Increase Java Heap Size Using Command Line
The first method to increase the heap size in Java is to use the command line to run the commands that override the default Java Heap Space. We follow the below two steps.
Step 1: Check the Default Maximum Heap Size in Java
Before we change the size of the heap in Java, we must know the default maximum heap size allocated to the JVM. To do that we use the code Runtime.getRuntime().maxMemory()
that returns the maximum heap size in bytes.
In the program, we get the maximum heap space in bytes and convert it to a more readable format like megabytes or gigabytes. The output shows that our current default size is around 1.48 GB.
We perform this step so that we do not allocate a smaller size than the heap’s default size.
public class JavaExample {
public static void main(String[] args) {
double maxHeapSize = Runtime.getRuntime().maxMemory();
String sizeInReadableForm;
double kbSize = maxHeapSize / 1024;
double mbSize = kbSize / 1024;
double gbSize = mbSize / 1024;
if (gbSize > 0) {
sizeInReadableForm = gbSize + " GB";
} else if (mbSize > 0) {
sizeInReadableForm = mbSize + " MB";
} else {
sizeInReadableForm = kbSize + " KB";
}
System.out.println("Maximum Heap Size: " + sizeInReadableForm);
}
}
Output:
Maximum Heap Size: 1.48046875 GB
Step 2: Use the Command Line to Set the Maximum Heap Size
Now that we know the maximum Java heap space, we increase it using the command line.
To get the commands that we can use to modify the heap size, we open the command line and use the command java -X
that returns a whole list of commands, but we are interested in only the following two commands.
The command -Xms
sets the initial and minimum heap size while the -Xms
sets the maximum size; we need to use the second command.
-Xms<size> set initial Java heap size
-Xmx<size> set maximum Java heap size
In the command line, we write the following command that runs the program that we see in the first step.
We use the -Xmx2g
to increase the heap size by 2GB. Notice that we write the storage unit as a single character; for example, if we want to set a 2GB heap size, we write 2g.
The output shows that the default size of around 1.4GB is now modified to 2.0GB.
java -Xmx2g JavaExample
Output:
Maximum Heap Size: 2.0 GB
Increase Java Heap Size in an Integrated Development Environment (IDE)
We can also increase Java heap space in an IDE if we run the program. Although some IDEs might have different steps to perform the task, if we follow these steps, we can modify the heap size in the majority of the IDEs.
-
We find the
Run
menu item in the menu bar. -
In the
Run
menu item, there will be an option namedRun Configurations
orEdit Configurations
that we have to open. -
A dialog box will open to show details about the application we want to run with the configurations.
There will be two input boxes: one for the program arguments and the second for VM arguments. If there is no input box for the VM arguments, we can add them using the
Modify Options
button. -
In the input box of the VM arguments, we write the following command to run the program with the increased heap size.
-Xmx2g
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn