How to Get Input From the Console in Java
-
Get Input From the Console Using the
Scanner
Class in Java -
Read Int Input Using the
Scanner
Class in Java -
Read Boolean Input Using the
Scanner
Class in Java
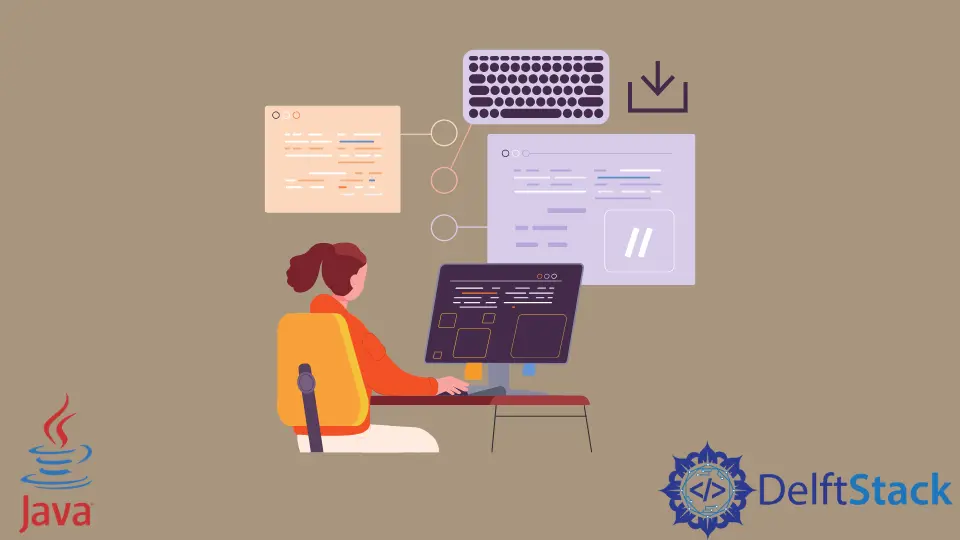
In this tutorial, we will look at the Scanner
class of Java and learn how we can read the input from the console using this class. The Scanner
class comes from the Java package java.util.Scanner
.
In the below examples, we will go through the methods of Scanner
that we can use to read inputs of different data types like String
, Int
, and boolean
.
Get Input From the Console Using the Scanner
Class in Java
The String
data type is commonly used when it comes to taking the input from the console. Scanner
has two functions next()
and nextLine()
that take the input as String
. The difference between these two functions is that next()
takes the input until it encounters whitespace, and nextLine()
reads the input till it sees a new line.
In our example, we use the nextLine()
method as we might want to have more than one word in a single line.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
System.out.println("Please enter your name: ");
Scanner sc = new Scanner(System.in);
String yourName = sc.nextLine();
System.out.println("Hello " + yourName);
}
}
Output:
Please enter your name:
Mike Stuart
Hello Mike Stuart
Read Int Input Using the Scanner
Class in Java
We can also get the int
values from the console using Scanner
. nextInt()
helps us to read int
values. In the below example, it asks for the age, which is commonly read as int
.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
System.out.println("Please enter your age: ");
Scanner sc = new Scanner(System.in);
int scanAge = sc.nextInt();
System.out.println("You are " + scanAge + " years old");
}
}
Output:
Please enter your age:
23
You are 23 years old
Read Boolean Input Using the Scanner
Class in Java
We can also take boolean
value as an input. Scanner.nextBoolean()
only reads either true
and false
or 0
and 1
.
In the below example, we take the input and then check if the input was true
or false
.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
System.out.println("Are you studying in college? Answer as True or False");
Scanner scanStudy = new Scanner(System.in);
boolean studyingInCollege = scanStudy.nextBoolean();
if (studyingInCollege) {
System.out.println("You are studying in college");
} else {
System.out.println("You are not studying in college");
}
}
}
Output:
Are you studying in college? Answer as True or False
false
You are not studying in college
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn