How to Clear the Console in Java
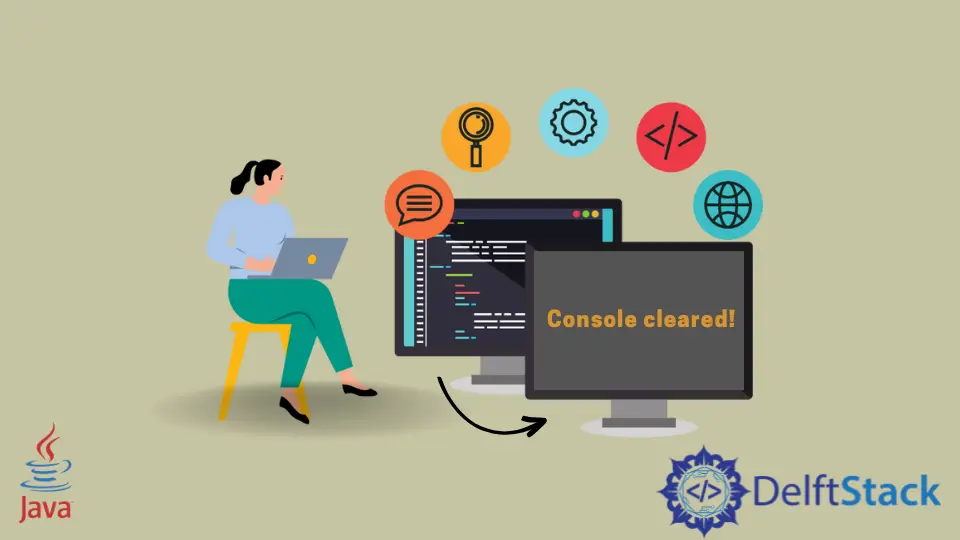
We have introduced how to get input from console in Java in another article.
In this tutorial, we will look at the two ways that can be used to clean the console screen in Java. We will be looking at examples to learn how to execute Java clear screen commands at runtime.
Use ANSI Escape Codes to Clear Console in Java
We can use special codes called ANSI escape code sequences to change cursor positions or display different colors. These sequences can be interpreted as commands that are a combination of bytes and characters.
To clear the console in Java, we will use the escape code \033[H\033[2J
. This weird set of characters represents the command to clean the console. To understand it better, we can break it down.
The first four characters \033
means ESC
or the escape character. Combining 033
with [H
, we can move the cursor to a specified position. The last characters, 033[2J
, cleans the whole screen.
We can look at the below example, which is using these escape codes. We are also using System.out.flush()
that is specially used for flushing out the remaining bytes when using System.out.print()
so that nothing gets left out on the console screen.
Example:
public class ClearConsoleScreen {
public static void main(String[] args) {
System.out.print("Everything on the console will cleared");
System.out.print("\033[H\033[2J");
System.out.flush();
}
}
Use ProcessBuilder
to Clear Console in Java
In this method, we will use a ProcessBuilder
that is a class mainly used to start a process. We can build a process with the commands that will clean the console.
ProcessBuilder()
takes in the commands to execute and its arguments. The issue with this approach is that different operating systems can have different commands to clean the console screen. It is why, in our example, we check the current operating system.
At last, we are using the Process
class to start a new process with inheritIO
to set the standard input and output channels to Java’s I/O channel.
public class ClearScreen {
public static void main(String[] args) {
System.out.println("Hello World");
ClearConsole();
}
public static void ClearConsole() {
try {
String operatingSystem = System.getProperty("os.name") // Check the current operating system
if (operatingSystem.contains("Windows")) {
ProcessBuilder pb = new ProcessBuilder("cmd", "/c", "cls");
Process startProcess = pb.inheritIO.start();
startProcess.waitFor();
}
else {
ProcessBuilder pb = new ProcessBuilder("clear");
Process startProcess = pb.inheritIO.start();
startProcess.waitFor();
}
} catch (Exception e) {
System.out.println(e);
}
}
}
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn