FTP in Java
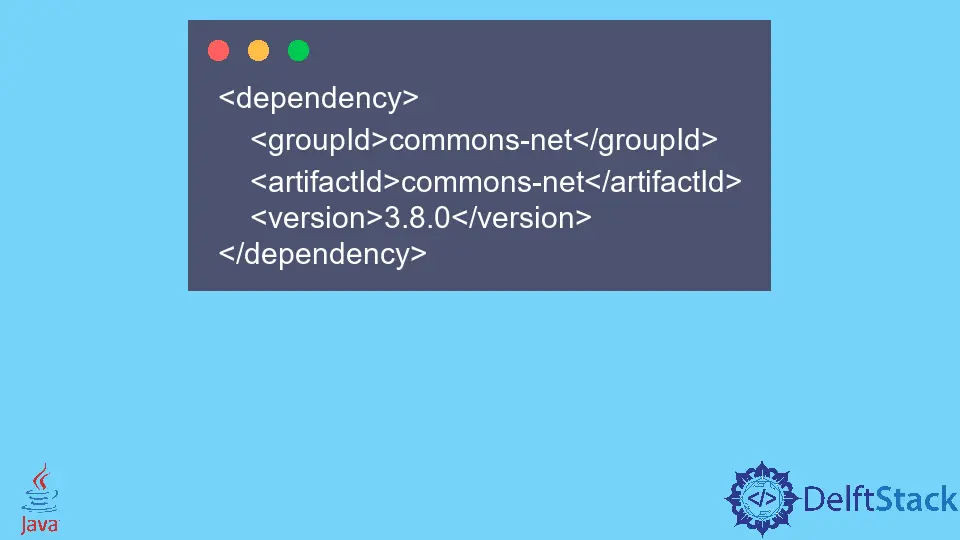
FTP or File Transfer Protocol is a communication protocol that allows us to transfer data from the server to a client. To get the files from the FTP server, we need to configure a client to communicate with the server.
We connect an FTP client to an FTP server and get a file in the following section.
Use Apache Commons Library to Use an FTP Client in Java
We need to use the following maven dependency in our project.
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.8.0</version>
</dependency>
For the program to work, we need a server that we create using www.drivehq.com
that provides a free FTP server that we can use for this example.
We need the credentials and hostname from the FTP server when created in the code. We save the credentials to variables and set the file path we want to download from the server.
We create an object of the FTPClient()
class, and to print every response from the FTP, we add a command listener using the addProtocolCommandListener()
method in which we pass an object of PrintCommandListener()
with the PrintWriter()
object and System.out
to print the messages on the console.
We create a file that will hold the data retrieved from the server. We do this using the File
class, and to get the OutputStream
object, we use the FileOutputStream
class.
We connect to the server using the connect()
method that takes the hostname. Our server needs authentication credentials to be accessed, so we use the login()
method of FTPClient
to pass the userName
and password
to it.
We get the response code from the server using the getReplyCode()
function to check if the connection was successful, and if it is not successful, we disconnect.
Finally, to get the file from the FTP server, we call the retrieveFile()
method that takes two arguments; the first argument is the filePath
, and the second argument is the outputStream
that the retrieved file is copied.
The retrieveFile()
returns a boolean
, which states whether the retrieval was successful or not. We show a message if the file is retrieved without any error.
At last, we close the output stream.
import java.io.*;
import org.apache.commons.net.PrintCommandListener;
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPReply;
public class ExampleClass3 {
public static void main(String[] args) {
String ftpHost = "ftp.drivehq.com";
String userName = "myFtpUsername";
String password = "123456";
String filePath = "\\testDoc.txt";
FTPClient ftpClient = new FTPClient();
ftpClient.addProtocolCommandListener(new PrintCommandListener(new PrintWriter(System.out)));
try {
File newFile = new File("testDoc.txt");
FileOutputStream fileOutputStream = new FileOutputStream(newFile);
OutputStream outputStream = new BufferedOutputStream(fileOutputStream);
ftpClient.connect(ftpHost);
ftpClient.login(userName, password);
int getFtpClientReply = ftpClient.getReplyCode();
if (!FTPReply.isPositiveCompletion(getFtpClientReply)) {
ftpClient.disconnect();
}
boolean success = ftpClient.retrieveFile(filePath, outputStream);
if (success)
System.out.println("File Retrieved Successfully.");
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
220 Welcome to the most popular FTP hosting service! Save on hardware, software, hosting and admin. Share files/folders with read-write permission. Visit http://www.drivehq.com/ftp/;
USER myFtpUsername
331 User name ok, need password.
PASS 123456
230 User myFtpUsername logged on. Free service has restrictions and is slower.
PORT 192,168,1,97,249,233
200 Port command successful.
RETR \testDoc.txt
150 Opening BINARY mode data connection for file transfer.
226 Transfer complete
File Retrieved Successfully.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn