Java 中的 FTP
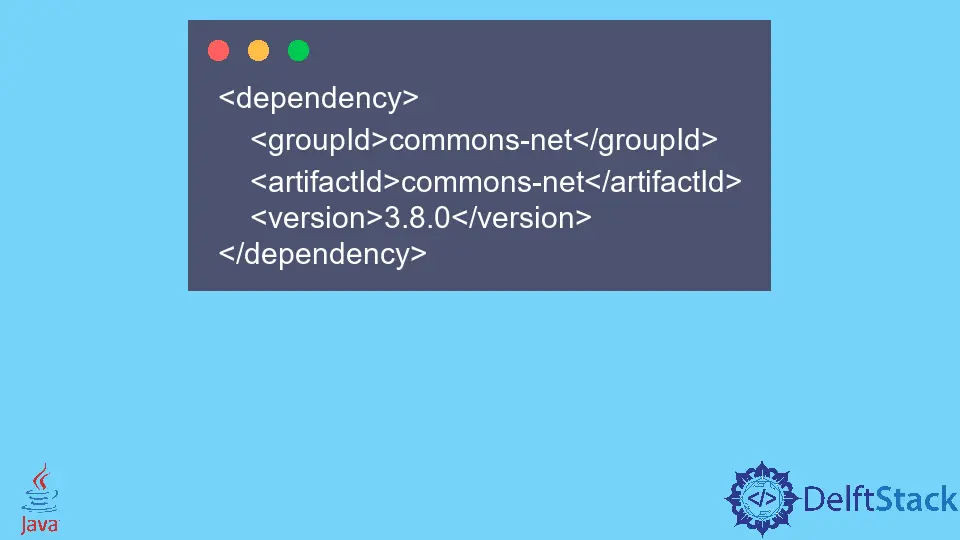
FTP 或文件传输协议是一种通信协议,它允许我们将数据从服务器传输到客户端。要从 FTP 服务器获取文件,我们需要配置一个客户端与服务器通信。
我们将 FTP 客户端连接到 FTP 服务器并在下一节中获取文件。
在 Java 中使用 Apache Commons Library 使用 FTP 客户端
我们需要在项目中使用以下 maven 依赖项。
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.8.0</version>
</dependency>
为了使程序正常工作,我们需要一个使用 www.drivehq.com
创建的服务器,它提供了一个免费的 FTP 服务器,我们可以在这个示例中使用它。
在代码中创建时,我们需要来自 FTP 服务器的凭据和主机名。我们将凭据保存到变量中并设置要从服务器下载的文件路径。
我们创建一个 FTPClient()
类的对象,并打印来自 FTP 的每个响应,我们使用 addProtocolCommandListener()
方法添加一个命令侦听器,其中我们传递一个 PrintCommandListener()
对象和 PrintWriter()
对象和 System.out
在控制台上打印消息。
我们创建一个文件来保存从服务器检索到的数据。我们使用 File
类执行此操作,为了获得 OutputStream
对象,我们使用 FileOutputStream
类。
我们使用带有主机名的 connect()
方法连接到服务器。我们的服务器需要访问身份验证凭据,因此我们使用 FTPClient
的 login()
方法将 userName
和 password
传递给它。
我们使用 getReplyCode()
函数从服务器获取响应代码来检查连接是否成功,如果不成功,我们断开连接。
最后,为了从 FTP 服务器获取文件,我们调用带有两个参数的 retrieveFile()
方法;第一个参数是 filePath
,第二个参数是复制检索到的文件的 outputStream
。
retrieveFile()
返回一个 boolean
,表示检索是否成功。如果文件被检索而没有任何错误,我们会显示一条消息。
最后,我们关闭输出流。
import java.io.*;
import org.apache.commons.net.PrintCommandListener;
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPReply;
public class ExampleClass3 {
public static void main(String[] args) {
String ftpHost = "ftp.drivehq.com";
String userName = "myFtpUsername";
String password = "123456";
String filePath = "\\testDoc.txt";
FTPClient ftpClient = new FTPClient();
ftpClient.addProtocolCommandListener(new PrintCommandListener(new PrintWriter(System.out)));
try {
File newFile = new File("testDoc.txt");
FileOutputStream fileOutputStream = new FileOutputStream(newFile);
OutputStream outputStream = new BufferedOutputStream(fileOutputStream);
ftpClient.connect(ftpHost);
ftpClient.login(userName, password);
int getFtpClientReply = ftpClient.getReplyCode();
if (!FTPReply.isPositiveCompletion(getFtpClientReply)) {
ftpClient.disconnect();
}
boolean success = ftpClient.retrieveFile(filePath, outputStream);
if (success)
System.out.println("File Retrieved Successfully.");
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
输出:
220 Welcome to the most popular FTP hosting service! Save on hardware, software, hosting and admin. Share files/folders with read-write permission. Visit http://www.drivehq.com/ftp/;
USER myFtpUsername
331 User name ok, need password.
PASS 123456
230 User myFtpUsername logged on. Free service has restrictions and is slower.
PORT 192,168,1,97,249,233
200 Port command successful.
RETR \testDoc.txt
150 Opening BINARY mode data connection for file transfer.
226 Transfer complete
File Retrieved Successfully.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn