How to Use the Suffix F in Java
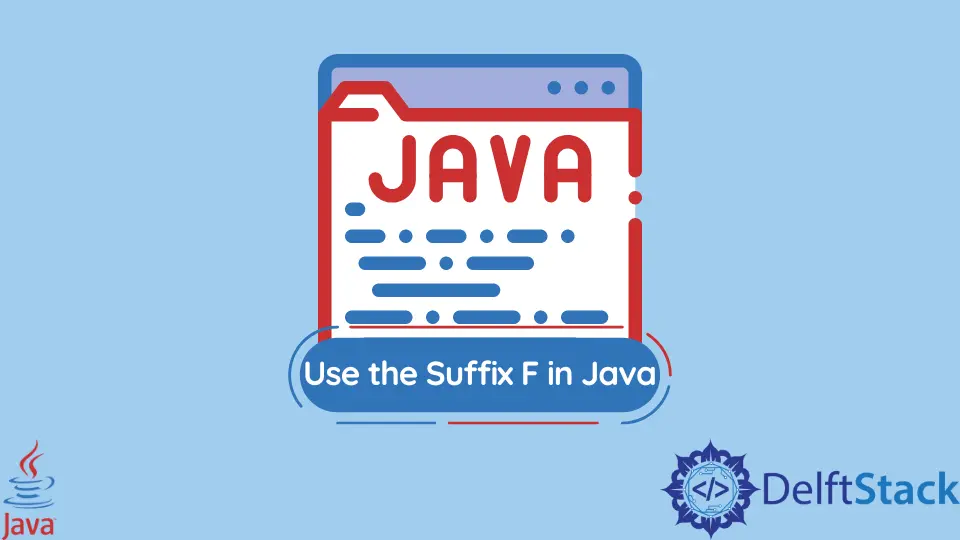
The decimal values in Java are by default double
values. When we only require float values, we must tell the compiler that it is a float value.
This tutorial demonstrates the use of the suffix f
in Java.
Use f
With a Value to Tell the Compiler It Is a Float Value in Java
We use the suffix f
after the value for that purpose. The value with the suffix f
will be identified as a float from the compiler.
Once we define the variable in Java, we have to specify the data type of that variable, but if a float is not predefined, we can use the suffix f
to tell the compiler it is a float value.
Example:
public class Java_F {
public static void main(String args[]) {
float num1 = 5.5f;
double num2 = 5f;
// Check the type of the variables above
System.out.println("The Data Types for the values are: ");
System.out.println(((Object) num1).getClass().getSimpleName() + " = " + num1);
System.out.println(((Object) num2).getClass().getSimpleName() + " = " + num2);
System.out.println(((Object) 5f).getClass().getSimpleName() + " = " + 5f);
}
}
Output:
The Data Types for the values are:
Float = 5.5
Double = 5.0
Float = 5.0
The code above uses f
with predefined variables and undefined values. This example checks for the data types of given values.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook