How to Check Type of a Variable in Java
-
Use
getClass().getSimpleName()
to Check the Type of a Variable in Java -
Use
getClass().getTypeName()
to Check the Type of a Variable in Java - Conclusion
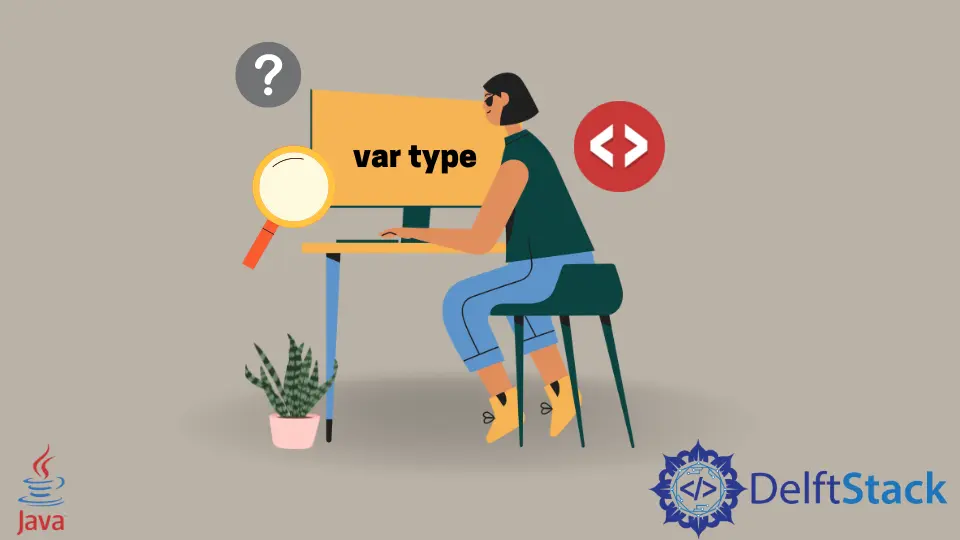
In Java, knowing the type of a variable or object is crucial for writing efficient and flexible code.
Two commonly used approaches to check the type of a variable involve the getClass().getSimpleName()
and getClass().getTypeName()
methods.
In this article, we will introduce how to utilize them to get the types of variables and objects in Java.
Use getClass().getSimpleName()
to Check the Type of a Variable in Java
We can check the type of a variable in Java by calling getClass().getSimpleName()
method via the variable.
These methods provide insights into the runtime class of an object and its fully qualified type name, respectively.
The getClass()
method is a fundamental feature available for all objects in Java. It provides information about the runtime class of an object, allowing you to understand the type of the object dynamically. Here’s the syntax for using the getClass()
method:
Class<?> objectClass = object.getClass();
Now, let’s break down how to use getClass()
in detail:
Step 1: Create an Object
To begin, you need an object whose type you want to ascertain. For this example, let’s create an instance of the String
class:
Object myObject = new String("Hello, Java!");
Step 2: Call the getClass()
Method
Next, you can invoke the getClass()
method on the object:
Class<?> objectClass = myObject.getClass();
The objectClass
variable now holds a Class
object representing the runtime class of myObject
.
Step 3: Explore Class Information
With the Class
object at your disposal, you can explore various aspects of the class, such as its name, modifiers, superclass, and implemented interfaces. For instance, to retrieve the simple name of the class (without package information), you can use getSimpleName()
:
String className = objectClass.getSimpleName();
This gives you the simple name of the class, which, in this case, is "String"
.
Complete Example Code
Here’s the complete code for using getClass()
:
public class GetTypeUsingGetClass {
public static void main(String[] args) {
Object myObject = new String("Hello, Java!");
Class<?> objectClass = myObject.getClass();
String className = objectClass.getSimpleName();
System.out.println("Object Type: " + className);
}
}
Output:
String[]
This method is callable by objects only; therefore, to check the type of primitive data types, we need to cast the primitive to Object
first. The below example illustrates how to use this function to check the type of non-primitive data types.
public class MyClass {
public static void main(String args[]) {
int x = 5;
System.out.println(((Object) x).getClass().getSimpleName());
}
}
Output:
Integer
Use getClass().getTypeName()
to Check the Type of a Variable in Java
Starting from Java 8, a new method called getTypeName()
is available to directly provide the fully qualified type name as a String
. It simplifies the process of obtaining the type name, as it does not require interacting with the Class
object. Here’s the syntax for using the getTypeName()
method:
String typeName = object.getClass().getTypeName();
Let’s delve into how to use getTypeName()
:
Step 1: Create an Object
Just like before, you need an object to determine its type:
Object myObject = new String("Hello, Java!");
Step 2: Call the getTypeName()
Method
Invoke the getTypeName()
method on the object:
String typeName = myObject.getClass().getTypeName();
The typeName
variable now holds a String
representing the fully qualified type name of the object.
Example Code
Here’s the complete code for using getTypeName()
:
public class GetTypeUsingGetTypeName {
public static void main(String[] args) {
Object myObject = new String("Hello, Java!");
String typeName = myObject.getClass().getTypeName();
System.out.println("Object Type: " + typeName);
}
}