How to End a Java Program
- Importance of Program Termination in Java
- Common Techniques for Terminating a Java Program
- Best Practices in Terminating a Java Program
- Conclusion
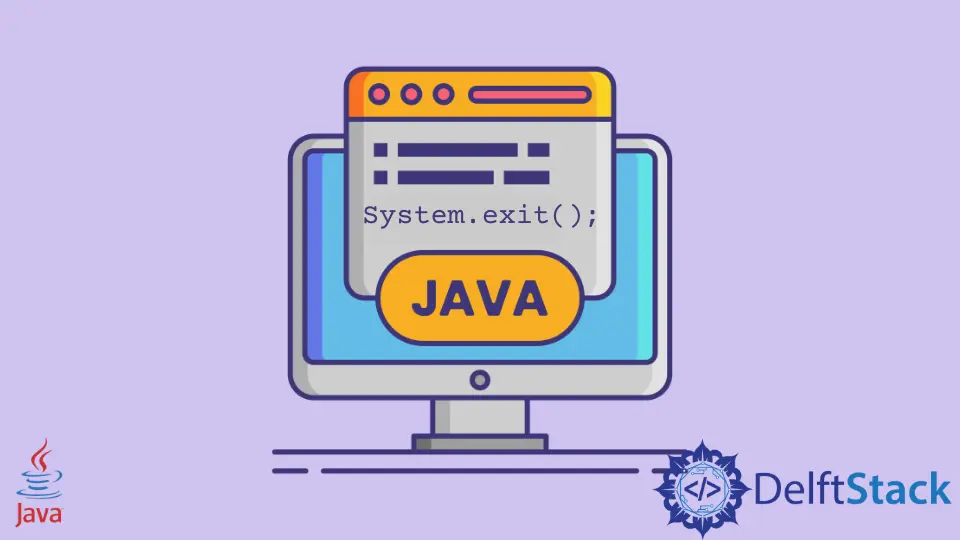
This article shows all the best ways that we can use to end a running program in Java. We will go through a few examples to understand the situation and the easiest way to handle it.
Importance of Program Termination in Java
Program termination in Java is crucial for maintaining system integrity and resource efficiency. Proper termination ensures that allocated resources, such as memory and open files, are released, preventing memory leaks and resource exhaustion.
It also allows for the execution of cleanup code, facilitating the orderly shutdown of processes. Additionally, gracefully terminating a program enhances user experience, preventing abrupt closures and potential data corruption.
Controlling program exit is essential for applications to operate reliably, preventing unintended consequences and contributing to the overall stability and performance of Java programs.
Common Techniques for Terminating a Java Program
Using the System.exit()
Method
To end a Java program, we can use the exit()
method of the System
class. It is the most popular way to end a program in Java.
The System.exit()
terminates the Java Virtual Machine (JVM) that exits the current program that we are running.
This method allows developers to explicitly terminate the Java Virtual Machine (JVM), ensuring a controlled and immediate shutdown of the program.
Code Example:
public class ProgramTerminator {
public static void main(String[] args) {
System.out.println("Program is starting...");
// Your program logic goes here
System.out.println("Program execution completed.");
// Terminate the program with exit code 0 (normal termination)
System.exit(0);
// The following code will not be executed
System.out.println("This line will not be printed.");
}
}
In this example, we begin by printing a message to indicate the start of the program. The placeholder comment (// Your program logic goes here
) represents the actual logic of your program, which can be any set of instructions.
Following the program logic, we print another message indicating the completion of the program.
Here, the critical aspect is the subsequent line that employs System.exit(0)
. This line serves as the termination point for the program, and any code below it will not be executed.
The argument 0
passed to System.exit()
signifies a normal, graceful termination. Developers can choose different integer values as exit codes, with non-zero values often indicating abnormal terminations or error conditions.
Output:
Upon execution, this program will display the Program is starting...
message, execute the program logic, print Program execution completed
, and then terminate gracefully. The last System.out.println()
statement and any subsequent code will be skipped due to the prior use of System.exit()
.
The System.exit()
method offers a straightforward means to conclude a Java program, ensuring proper cleanup and controlled termination. Its judicious use contributes to the development of robust and well-behaved Java applications.
Using the return
Statement
This approach of using the return
statement provides a clean and controlled way to conclude a Java program, allowing for proper finalization steps and ensuring a smooth exit. It is particularly useful in scenarios where explicit termination via System.exit()
might be too abrupt.
Code Example:
public class ProgramTerminator {
public static void main(String[] args) {
System.out.println("Program is starting...");
// Your program logic goes here
System.out.println("Program execution completed.");
// Terminate the program gracefully by returning from the main method
return;
}
}
In this example, we initiate the program by printing Program is starting...
.
The subsequent comment placeholder (// Your program logic goes here
) signifies the area where your specific program logic would reside. This could be any sequence of instructions, depending on the requirements of your application.
Continuing with the program, we print Program execution completed
. as an indicator of successful completion.
The key element here is the return
statement immediately following this message. When encountered, the return
statement causes the main
method to exit, leading to the natural termination of the program.
It is important to note that any code below the return
statement, such as the commented out System.out.println()
, will not be executed.
Output:
Upon execution, this program will display Program is starting...
, execute the program logic, print Program execution completed
, and then gracefully terminate as the return
statement is encountered. The subsequent code, including the commented out System.out.println()
, will be skipped.
Best Practices in Terminating a Java Program
Use the return
Statement
Conclude your Java program by allowing the main
method to naturally return. This ensures that any cleanup code following the return
statement is executed before termination.
Leverage System.exit()
Judiciously
If an immediate and unconditional termination is necessary, employ System.exit()
with caution. Be mindful of potential consequences, and use it sparingly to prevent premature program halts.
Handle Exceptions Gracefully
If an unexpected condition arises during program execution, handle exceptions gracefully. Implement appropriate exception handling mechanisms to ensure a controlled exit and provide meaningful error messages.
Close Resources in a finally
Block
If your program involves resource management (e.g., file handling, database connections), use a finally
block to guarantee that resources are properly closed, even in the presence of exceptions.
Consider Shutdown Hooks for Cleanup
For complex applications, utilize shutdown hooks to register cleanup tasks that should be executed before program termination. This mechanism allows for orderly shutdown procedures.
Communicate Program Termination
Provide clear and informative messages indicating the start and completion of program execution. This enhances user experience and aids in debugging when necessary.
Avoid Unconventional Termination Methods
Steer clear of unconventional termination methods, such as throwing uncaught exceptions solely for program termination. These practices can lead to unexpected consequences and hinder maintainability.
Document Termination Procedures
Document the termination procedures within your code, especially if employing specific methods like System.exit()
. This aids in understanding the intended behavior during program termination.
Test Termination Scenarios
Include termination scenarios in your testing procedures to ensure that the program exits gracefully and handles exceptional cases appropriately. This helps in identifying and addressing potential issues early in the development process.
Conclusion
In the realm of Java programming, understanding the significance of proper program termination is paramount for building robust and reliable applications. We explored various methods, from the conventional use of return
statements to the more forceful System.exit()
.
The choice of termination method depends on the specific needs of your application. Embracing best practices, such as resource cleanup, graceful exception handling, and clear communication during program execution, contributes to the overall stability and maintainability of Java programs.
By adhering to these principles, developers can ensure that their applications exit gracefully, providing a seamless user experience and mitigating potential issues. Termination in Java is not just about ending a program; it’s about doing so in a controlled, predictable, and responsible manner.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn