How to Disable SSL Validation in Java
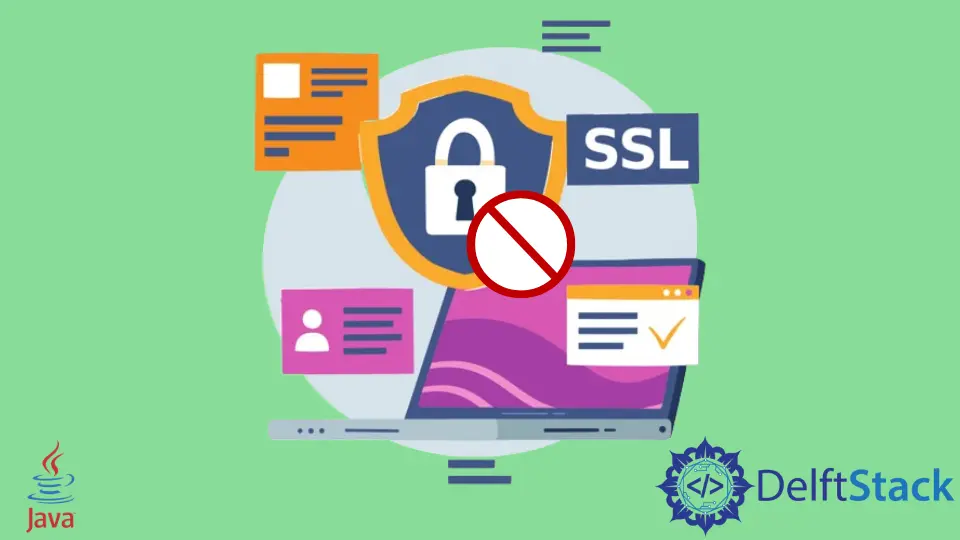
Sometimes when we try to connect to a URL, you may get an SSLHandshakeException
or IOException
error. The reason behind this error is the failure to establish an SSL-encrypted
connection.
In Java, when we try to open an SSL
connection, the JSSE
implementation of the SSL
protocol performs some validation process to check whether the requested host is real or fake. This checking involves the validation of the server’s X.509 certificate
.
This article will show how we can disable this certificate validation when creating an HTTP
connection. Also, we’ll write an example code with an explanation regarding the topic to make it easy to understand.
Java Disable SSL
Validation
Before moving towards the solution, let’s look at the error below that we get when we try to connect with an URL:
Caused by: javax.net.ssl.SSLHandshakeException: sun.security.validator.ValidatorException: PKIX path building failed: sun.security.provider.certpath.SunCertPathBuilderException: unable to find valid certification path to requested target
at sun.security.ssl.Alerts.getSSLException(Alerts.java:110)
at sun.security.ssl.SSLSocketImpl.fatal(SSLSocketImpl.java:149)
at sun.security.ssl.Handshaker.fatalSE(Handshaker.java:202)
The above exceptions can be avoided by turning off the Host Verification
and the Certificate Validation
for SSL
. To achieve that, you can follow the below example.
Solution: Turn Off the Host Verification
& Certificate Validation
In our below example, we illustrated how we could turn off the Host Verification
and the Certificate Validation
. See the following code.
Example Code:
// importing necessary packages
import java.io.InputStreamReader;
import java.io.Reader;
import java.net.URL;
import java.net.URLConnection;
import java.security.SecureRandom;
import java.security.cert.X509Certificate;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLSession;
import javax.net.ssl.TrustManager;
import javax.net.ssl.X509TrustManager;
// Our main class
public class DisableSSL {
public static void main(String[] args) throws Exception {
// Creating a Trust Manager.
TrustManager[] TrustMgr = new TrustManager[] {
new X509TrustManager(){public X509Certificate[] getAcceptedIssuers(){return null;
}
// No Checking required
public void checkClientTrusted(X509Certificate[] certs, String authType) {}
// No Checking required
public void checkServerTrusted(X509Certificate[] certs, String authType) {}
}
}
;
// Installing the Trast manager
SSLContext SSLCont = SSLContext.getInstance("SSL");
SSLCont.init(null, TrustMgr, new SecureRandom());
HttpsURLConnection.setDefaultSSLSocketFactory(SSLCont.getSocketFactory());
// Creating an all-trusting verifier for Host Name
HostnameVerifier ValidHost = new HostnameVerifier() {
public boolean verify(String HostName, SSLSession MySession) {
return true;
}
};
// Installing an all-trusting verifier for the HOST
HttpsURLConnection.setDefaultHostnameVerifier(ValidHost);
URL MyURL = new URL("https://www.example.com/"); // The location URL
URLConnection connect = MyURL.openConnection(); // Openning the URL connection
Reader MyReader = new InputStreamReader(connect.getInputStream()); // Creating a reader
while (true) { // Processing the reader data
int Chars = MyReader.read();
if (Chars == -1) {
break;
}
System.out.print((char) Chars);
}
}
}
We already described the purpose of each line via comments
. However, if you look at the code deeply, you will find that we didn’t define anything for the checkClientTrusted
and the checkServerTrusted
.
In this way, we bypass the validation. Therefore, executing the above example code will get an output like the one below on your console.
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8" />
<meta http-equiv="Content-type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif;
}
div {
width: 600px;
margin: 5em auto;
padding: 2em;
background-color: #fdfdff;
border-radius: 0.5em;
box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02);
}
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this
domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn