Java 비활성화 SSL 유효성 검사
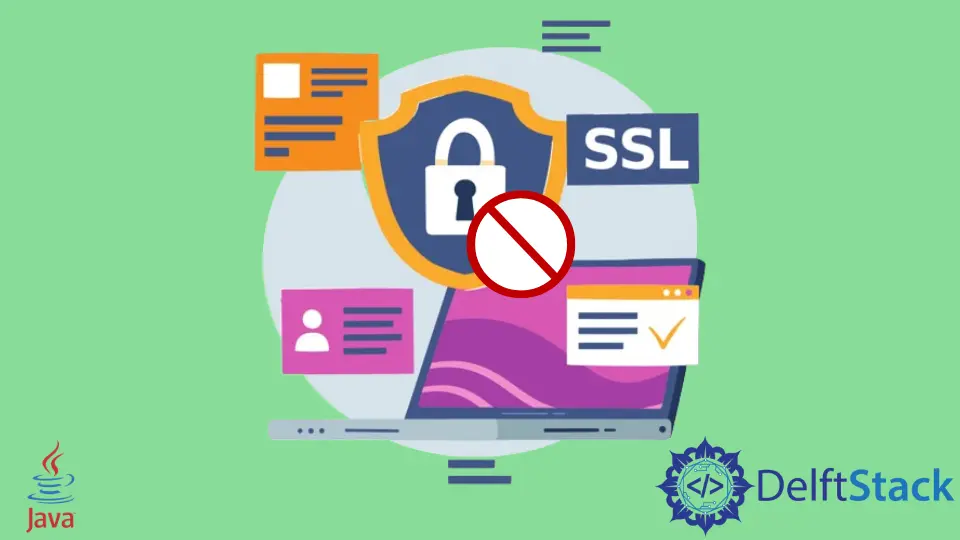
때때로 URL에 연결하려고 할 때 SSLHandshakeException
또는 IOException
오류가 발생할 수 있습니다. 이 오류의 원인은 SSL 암호화
연결을 설정하지 못했기 때문입니다.
Java에서 SSL
연결을 열려고 하면 SSL
프로토콜의 JSSE
구현이 일부 유효성 검사 프로세스를 수행하여 요청된 호스트가 진짜인지 가짜인지 확인합니다. 이 확인에는 서버의 X.509 인증서
유효성 검사가 포함됩니다.
이 문서에서는 HTTP
연결을 생성할 때 이 인증서 유효성 검사를 비활성화하는 방법을 보여줍니다. 또한 이해하기 쉽도록 주제에 대한 설명과 함께 예제 코드를 작성할 것입니다.
Java 비활성화 SSL
유효성 검사
솔루션으로 이동하기 전에 URL로 연결하려고 할 때 발생하는 아래 오류를 살펴보겠습니다.
Caused by: javax.net.ssl.SSLHandshakeException: sun.security.validator.ValidatorException: PKIX path building failed: sun.security.provider.certpath.SunCertPathBuilderException: unable to find valid certification path to requested target
at sun.security.ssl.Alerts.getSSLException(Alerts.java:110)
at sun.security.ssl.SSLSocketImpl.fatal(SSLSocketImpl.java:149)
at sun.security.ssl.Handshaker.fatalSE(Handshaker.java:202)
SSL
에 대한 호스트 확인
및 인증서 유효성 검사
를 끄면 위의 예외를 방지할 수 있습니다. 이를 달성하기 위해 아래 예를 따를 수 있습니다.
해결 방법: 호스트 확인
및 인증서 유효성 검사
를 끕니다.
아래 예에서는 호스트 확인
및 인증서 유효성 검사
를 해제하는 방법을 설명했습니다. 다음 코드를 참조하십시오.
예제 코드:
// importing necessary packages
import java.io.InputStreamReader;
import java.io.Reader;
import java.net.URL;
import java.net.URLConnection;
import java.security.SecureRandom;
import java.security.cert.X509Certificate;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLSession;
import javax.net.ssl.TrustManager;
import javax.net.ssl.X509TrustManager;
// Our main class
public class DisableSSL {
public static void main(String[] args) throws Exception {
// Creating a Trust Manager.
TrustManager[] TrustMgr = new TrustManager[] {
new X509TrustManager(){public X509Certificate[] getAcceptedIssuers(){return null;
}
// No Checking required
public void checkClientTrusted(X509Certificate[] certs, String authType) {}
// No Checking required
public void checkServerTrusted(X509Certificate[] certs, String authType) {}
}
}
;
// Installing the Trast manager
SSLContext SSLCont = SSLContext.getInstance("SSL");
SSLCont.init(null, TrustMgr, new SecureRandom());
HttpsURLConnection.setDefaultSSLSocketFactory(SSLCont.getSocketFactory());
// Creating an all-trusting verifier for Host Name
HostnameVerifier ValidHost = new HostnameVerifier() {
public boolean verify(String HostName, SSLSession MySession) {
return true;
}
};
// Installing an all-trusting verifier for the HOST
HttpsURLConnection.setDefaultHostnameVerifier(ValidHost);
URL MyURL = new URL("https://www.example.com/"); // The location URL
URLConnection connect = MyURL.openConnection(); // Openning the URL connection
Reader MyReader = new InputStreamReader(connect.getInputStream()); // Creating a reader
while (true) { // Processing the reader data
int Chars = MyReader.read();
if (Chars == -1) {
break;
}
System.out.print((char) Chars);
}
}
}
우리는 이미 주석
을 통해 각 줄의 목적을 설명했습니다. 그러나 코드를 자세히 살펴보면 checkClientTrusted
및 checkServerTrusted
에 대해 아무 것도 정의하지 않았음을 알 수 있습니다.
이런 식으로 유효성 검사를 우회합니다. 따라서 위의 예제 코드를 실행하면 콘솔에 아래와 같은 출력이 표시됩니다.
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8" />
<meta http-equiv="Content-type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif;
}
div {
width: 600px;
margin: 5em auto;
padding: 2em;
background-color: #fdfdff;
border-radius: 0.5em;
box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02);
}
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this
domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn