Decorator in Java
- Special Notes on the Decorator Design Pattern in Java
- An Example of a Decorator Design Pattern in Java
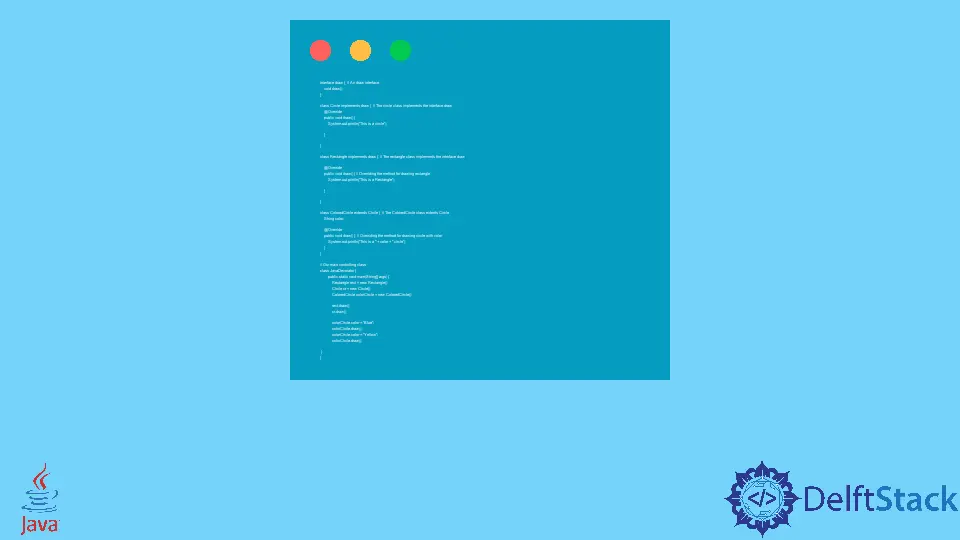
The Decorator design pattern enables users to add functionality dynamically and set objects’ behavior without affecting their objects’ behavior. To extend the behavior of the class, the decorator mostly uses inheritance.
In this article, we will discuss the Decorator design pattern in Java. Also, we are going to cover the topic with the help of examples and explanations to make the topic easier.
Special Notes on the Decorator Design Pattern in Java
Below some important notes on the Decorator design pattern are shared:
- Through the Decorator design pattern, you can add functionality to an object of a class without making any changes to the actual structure of the class.
- The Decorator design pattern uses the abstract classes or interface.
- One of the disadvantages of the Decorator design pattern is that it uses several similar objects.
An Example of a Decorator Design Pattern in Java
Below, we shared an example regarding the Decorator design pattern. First, see the structure that we are going to implement:
Draw
|----- Circle
| |------ Blue Circle
| |------ Yellow Circle
|
|----- Rectangle
Take a look at the following code where we implement the above structure:
interface draw { // An draw interface
void draw();
}
class Circle implements draw { // The circle class implements the interface draw
@Override
public void draw() {
System.out.println("This is a circle");
}
}
class Rectangle implements draw { // The rectangle class implements the interface draw
@Override public void draw() { // Overriding the method for drawing rectangle
System.out.println("This is a Rectangle");
}
}
class ColoredCircle extends Circle { // The ColoredCircle class extents Circle
String color;
@Override public void draw() { // Overriding the method for drawing circle with color
System.out.println("This is a " + color + " circle");
}
}
// Our main controlling class
class JavaDecorator {
public static void main(String[] args) {
Rectangle rect = new Rectangle();
Circle cr = new Circle();
ColoredCircle colorCircle = new ColoredCircle();
rect.draw();
cr.draw();
colorCircle.color = "Blue";
colorCircle.draw();
colorCircle.color = "Yellow";
colorCircle.draw();
}
}
We have commanded the purpose of each line. Now, after running the example code, you will see the below output:
This is a Rectangle
This is a circle
This is a Blue circle
This is a Yellow circle
Please note that the example codes shared in this article are in Java, and you must install Java on your environment if your system doesn’t have Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn