자바의 데코레이터
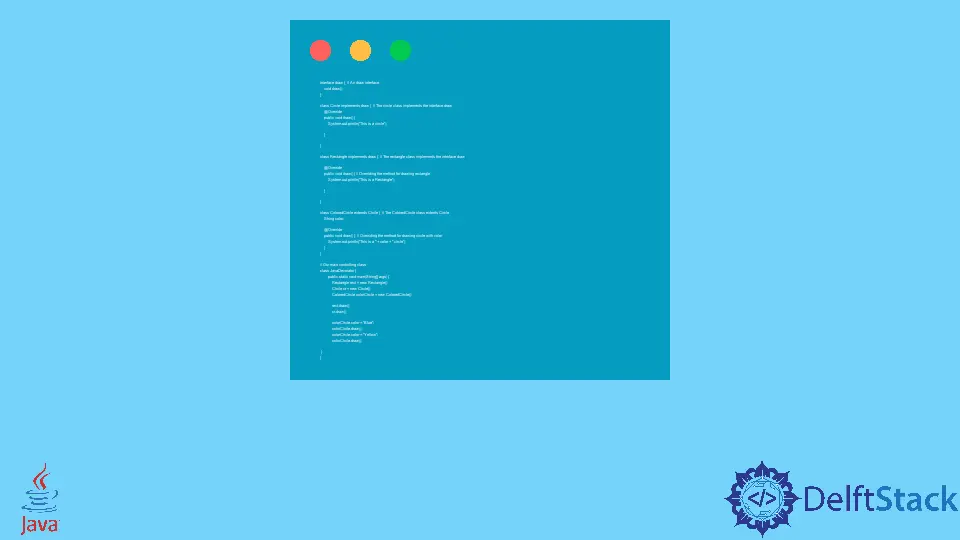
데코레이터 디자인 패턴을 사용하면 사용자가 기능을 동적으로 추가하고 개체의 동작에 영향을 주지 않고 개체의 동작을 설정할 수 있습니다. 클래스의 동작을 확장하기 위해 데코레이터는 주로 상속을 사용합니다.
이 기사에서는 Java의 데코레이터 디자인 패턴에 대해 설명합니다. 또한 주제를 쉽게 이해할 수 있도록 예제와 설명을 통해 주제를 다룰 것입니다.
Java의 데코레이터 디자인 패턴에 대한 특별 참고 사항
Decorator 디자인 패턴에 대한 몇 가지 중요한 참고 사항은 다음과 같습니다.
- 데코레이터 디자인 패턴을 통해 클래스의 실제 구조를 변경하지 않고 클래스의 개체에 기능을 추가할 수 있습니다.
- 데코레이터 디자인 패턴은 추상 클래스 또는 인터페이스를 사용합니다.
- Decorator 디자인 패턴의 단점 중 하나는 유사한 객체를 여러 개 사용한다는 것입니다.
Java에서 데코레이터 디자인 패턴의 예
아래에서 Decorator 디자인 패턴에 대한 예제를 공유했습니다. 먼저 구현할 구조를 확인하십시오.
Draw
|----- Circle
| |------ Blue Circle
| |------ Yellow Circle
|
|----- Rectangle
위의 구조를 구현하는 다음 코드를 살펴보십시오.
interface draw { // An draw interface
void draw();
}
class Circle implements draw { // The circle class implements the interface draw
@Override
public void draw() {
System.out.println("This is a circle");
}
}
class Rectangle implements draw { // The rectangle class implements the interface draw
@Override public void draw() { // Overriding the method for drawing rectangle
System.out.println("This is a Rectangle");
}
}
class ColoredCircle extends Circle { // The ColoredCircle class extents Circle
String color;
@Override public void draw() { // Overriding the method for drawing circle with color
System.out.println("This is a " + color + " circle");
}
}
// Our main controlling class
class JavaDecorator {
public static void main(String[] args) {
Rectangle rect = new Rectangle();
Circle cr = new Circle();
ColoredCircle colorCircle = new ColoredCircle();
rect.draw();
cr.draw();
colorCircle.color = "Blue";
colorCircle.draw();
colorCircle.color = "Yellow";
colorCircle.draw();
}
}
우리는 각 라인의 목적을 명령했습니다. 이제 예제 코드를 실행하면 아래 출력이 표시됩니다.
This is a Rectangle
This is a circle
This is a Blue circle
This is a Yellow circle
이 문서에서 공유하는 예제 코드는 Java로 되어 있으며 시스템에 Java가 없는 경우 환경에 Java를 설치해야 합니다.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn