Closure in Java
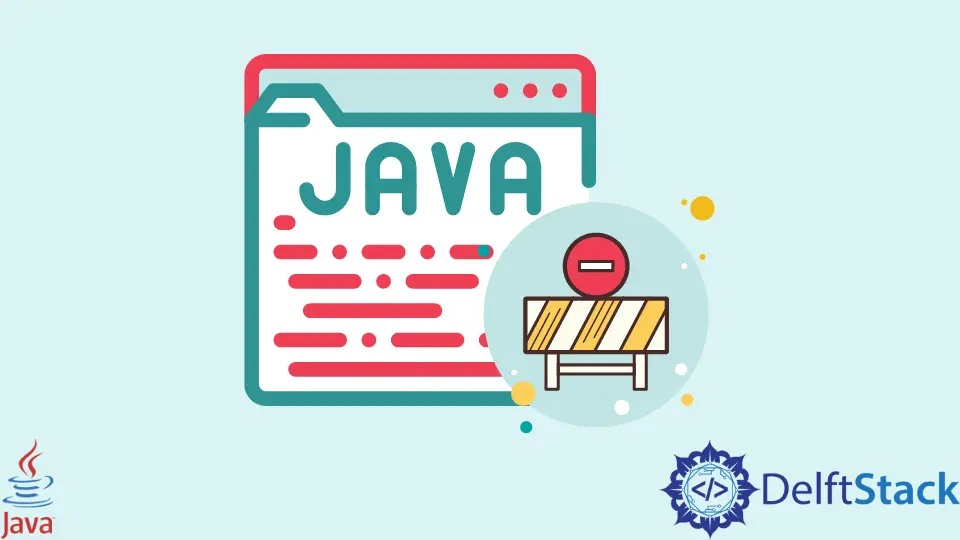
If we define a lambda expression through which we are willing to increment the value of a variable, it will throw an error.
However, there’s an easy solution to this problem. By using Closures
, we can prevent this error.
Closures
are the inline function and have valued expressions, meaning they are the functions of a class with bounded variables. Another exciting feature of Closures
is that they can be passed to another function as a parameter.
This article will discuss Closures
in detail and provide necessary examples and explanations to make the topic easier.
Before going into the discussion, let’s see the general syntax of closure which is something like the below.
(List_of_Arguments) -> {
Function_Body
}
Closure in Java
In our below example, we will illustrate the most basic of the Closures
where we used Closures
to combine two strings. Let’s take a look at our below code.
package javacodes;
// Importing necessary packages
import java.io.*;
interface CombineString { // Defining a interface
public String concat(String a, String b);
}
// The controlling class
public class JavaClosures {
public static void main(String[] args) {
// Using the "Lambda Expression"
CombineString cs = (str1, str2) -> str1 + str2;
System.out.println("The combined string is: " + cs.concat("Alen", " Walker"));
}
}
We already commented on the purpose of each line. In the example above, we first defined an interface named CombineString
with an abstract method named concat
.
Now in our controlling class, we just created a Closure
to define the abstract method.
After running the example above, you will get an output like the one below.
The combined string is: Alen Walker
Nested Use of Closures in Java
Let’s get a bit deeper into our topic. Look at our example below, where we created a nested call between two Closures
.
interface PrintData { // An interface to print data
public void Print(int data); // An abstract method to print data
}
interface NumberAdd { // An interface to add two numbers
public void add(int a, int b); // An abstract method to add two numbers
}
// Main controlling class
public class JavaClosures {
public static void main(String[] args) {
// Using the "Lambda Expression"
PrintData printdata = (str) -> {
System.out.println("The combined string is: " + str);
};
NumberAdd addnum = (x, y) -> {
printdata.Print(x + y); // The nested call
};
addnum.add(5, 5); // Calling the add method
}
}