자바의 폐쇄
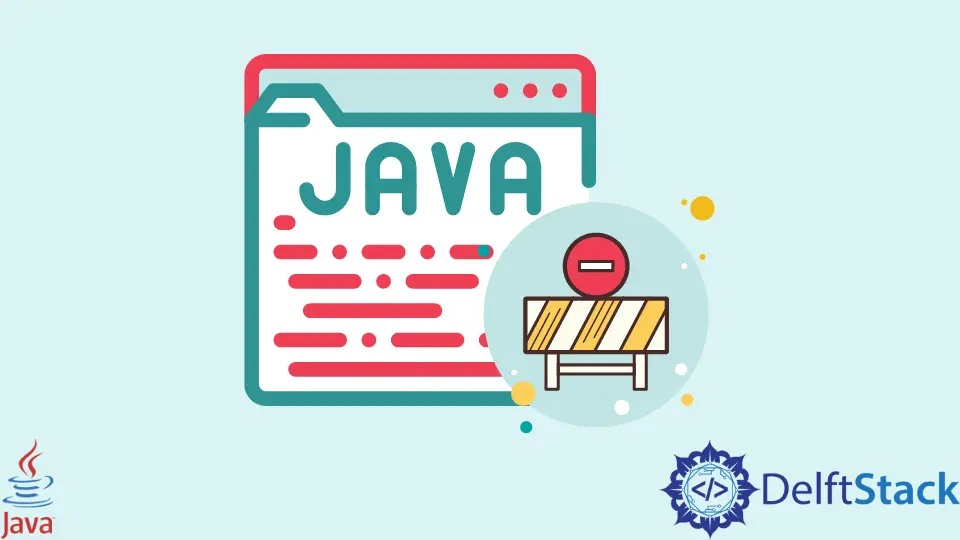
변수 값을 증가시키려는 람다 식을 정의하면 오류가 발생합니다.
그러나이 문제에 대한 쉬운 해결책이 있습니다. Closures
를 사용하면 이 오류를 방지할 수 있습니다.
Closures
는 인라인 함수이며 값이 있는 표현식을 가지고 있습니다. 즉, 제한된 변수가 있는 클래스의 함수입니다. Closures
의 또 다른 흥미로운 기능은 다른 함수에 매개변수로 전달할 수 있다는 것입니다.
이 기사에서는 폐쇄
에 대해 자세히 논의하고 주제를 더 쉽게 만들기 위해 필요한 예제와 설명을 제공합니다.
토론에 들어가기 전에 아래와 같은 일반적인 클로저 구문을 살펴보겠습니다.
(List_of_Arguments) -> {
Function_Body
}
자바의 폐쇄
아래 예제에서는 Closures
를 사용하여 두 문자열을 결합하는 가장 기본적인 Closures
를 설명합니다. 아래 코드를 살펴보겠습니다.
package javacodes;
// Importing necessary packages
import java.io.*;
interface CombineString { // Defining a interface
public String concat(String a, String b);
}
// The controlling class
public class JavaClosures {
public static void main(String[] args) {
// Using the "Lambda Expression"
CombineString cs = (str1, str2) -> str1 + str2;
System.out.println("The combined string is: " + cs.concat("Alen", " Walker"));
}
}
우리는 이미 각 행의 목적에 대해 설명했습니다. 위의 예에서 우리는 먼저 concat
이라는 추상 메서드를 사용하여 CombineString
이라는 인터페이스를 정의했습니다.
이제 제어 클래스에서 추상 메서드를 정의하기 위해 Closure
를 만들었습니다.
위의 예제를 실행하면 아래와 같은 결과를 얻을 수 있습니다.
The combined string is: Alen Walker
Java에서 클로저의 중첩 사용
우리 주제에 대해 좀 더 깊이 들어가 봅시다. 두 Closures
사이에 중첩된 호출을 생성한 아래 예제를 살펴보십시오.
interface PrintData { // An interface to print data
public void Print(int data); // An abstract method to print data
}
interface NumberAdd { // An interface to add two numbers
public void add(int a, int b); // An abstract method to add two numbers
}
// Main controlling class
public class JavaClosures {
public static void main(String[] args) {
// Using the "Lambda Expression"
PrintData printdata = (str) -> {
System.out.println("The combined string is: " + str);
};
NumberAdd addnum = (x, y) -> {
printdata.Print(x + y); // The nested call
};
addnum.add(5, 5); // Calling the add method
}
}
우리는 이미 각 행의 목적에 대해 설명했습니다. 위의 예에서는 먼저 PrintData
및 NumberAdd
인터페이스를 만들었습니다.
인터페이스 PrintData
에는 추상 메서드 Print
가 포함되고 인터페이스 NumberAdd
에는 추상 메서드 add
가 포함됩니다.
이제 제어 클래스에서 우리는 먼저 추상 메서드 Print
를 정의했습니다. 이 메서드를 거칠게 호출할 것입니다.
그런 다음 추상 메서드 add
를 정의하고 이 메서드의 정의를 보면 Print
메서드를 호출한 것을 볼 수 있습니다.
위의 예제를 실행하면 아래와 같은 결과를 얻을 수 있습니다.
The combined string is: 10
여기에서 공유되는 코드 예제는 Java로 되어 있으며 시스템에 Java가 포함되어 있지 않은 경우 환경에 Java를 설치해야 합니다.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn