How to Generate Barcodes in Java
- Types of Barcodes
-
Use the
zxing
Library to Generate Barcodes in Java -
Use the
itextpdf
Library to Generate Barcodes in Java - Conclusion
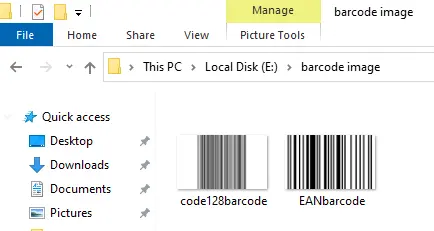
A barcode consists of bars and spaces. It is simply a machine-readable representation of characters and numbers in the form of a pattern of parallel lines of different widths.
These patterns are affixed to retail identification cards, store items, and postal mail to identify a particular product’s number, location, or name. A barcode symbol has five components, i.e., a quiet zone, a start character, a stop character, data characters, and another quiet zone.
Types of Barcodes
To identify barcodes, let’s have a look at the types of barcodes which are shown below:
- Linear (1D) barcodes
- Matrix (2D) barcodes
Some of the most used barcode formats are UPC, Code 39, Extended Code39, Code 128, EAN 128, and QR Code.
We can generate barcodes in Java with the help of the two most used libraries, which are itextpdf
and zxing
.
Use the zxing
Library to Generate Barcodes in Java
We can use the zxing
library to generate barcodes in Java. zxing
means Zebra Crossing.
To use this library, we must follow these steps in IntelliJ IDEA.
-
Create a new project with the language Java and build system maven.
-
Open
pom.xml
and write the following code.<dependencies> <dependency> <groupId>com.google.zxing</groupId> <artifactId>core</artifactId> <version>3.3.0</version> </dependency> <dependency> <groupId>com.google.zxing</groupId> <artifactId>javase</artifactId> <version>3.3.0</version> </dependency> </dependencies>
-
Press the
load maven changes
button or press the shortcut key ctrl+shift+o.
When we sync dependencies, we can import zxing
in our program.
Example:
This code will generate an image of the two mainly used barcodes, Code 128 and EAN 8. Remember, you must create the path of the images on your computer.
package org.example;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.client.j2se.MatrixToImageWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.oned.Code128Writer;
import com.google.zxing.oned.EAN8Writer;
import java.nio.file.Paths;
public class Main {
public static void main(String[] args) {
try {
// Content for encode function of code128writer
String text = "http://www.google.com";
// Path where we save our barcode
String path = "E:\\barcode image\\code128barcode.jpg";
// Barcode code128 writer object
Code128Writer c128writer = new Code128Writer();
// Represents a 2D matrix of bits. Create a bar code with a width of 500 and a height of 300
BitMatrix matrix = c128writer.encode(text, BarcodeFormat.CODE_128, 500, 300);
MatrixToImageWriter.writeToPath(matrix, "jpg", Paths.get(path));
System.out.println("Barcode image file is created...");
// Digits for encode function of EAN_8
String digits = "12345678";
// Path where we save our barcode
path = "E:\\barcode image\\EANbarcode.jpg";
// Barcode EAN8 writer object
EAN8Writer EANwriter = new EAN8Writer();
/*Represents a 2D matrix of bits.
Create an EAN bar code of width 500 and height 300*/
matrix = EANwriter.encode(digits, BarcodeFormat.EAN_8, 500, 300);
MatrixToImageWriter.writeToPath(matrix, "jpg", Paths.get(path));
System.out.println("Barcode image file is created...");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
Use the itextpdf
Library to Generate Barcodes in Java
With the help of the itextpdf
library, we can generate barcodes in Java and easily store them in PDF format. To use this library, we must follow these steps.
-
Download the
itextpdf
library from here. -
Open IntelliJ IDEA.
-
Create a new project with the language Java.
-
Right-click the project folder.
-
Open the module setting or press F4.
-
Click on the
dependencies
tab. -
Press the
add
button or press Alt+Insert. -
Select
itextpdf.jar
, which we downloaded from theitextpdf
website.
We can import itextpdf
in our Java code to generate barcodes.
Example:
With the help of this example, we can store Code 128 and EAN 13 barcodes in PDF format.
package org.example;
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.Image;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.pdf.Barcode128;
import com.itextpdf.text.pdf.BarcodeEAN;
import com.itextpdf.text.pdf.PdfContentByte;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.FileOutputStream;
public class Main {
public static void main(String[] args) {
Main.barcodePDF("mybarcodepdf.pdf", "Zeshan");
}
public static void barcodePDF(String pdfFilename, String myString) {
// declare object of document class of the itextpdf library
Document doc = new Document();
PdfWriter pdfWriter = null;
try {
// initialize docWriter object with the path of the pdf file
// mention the path of your computer
pdfWriter =
PdfWriter.getInstance(doc, new FileOutputStream("E:\\my barcode pdf\\" + pdfFilename));
// set pdf file properties
doc.addAuthor("zeshan");
doc.addCreationDate();
doc.addProducer();
doc.addCreator("google.com");
doc.addTitle("java barcode generation");
doc.setPageSize(PageSize.A4);
doc.open();
/*PdfContentByte is an object that contains the user-positioned text and
graphic contents of a page*/
PdfContentByte cb = pdfWriter.getDirectContent();
// type of barcode
Barcode128 code128 = new Barcode128();
// remove all leading and trail space and pass to set code
code128.setCode(myString.trim());
code128.setCodeType(Barcode128.CODE128);
/*create the image of the barcode with null bar color and null text color
set its position and scale percent*/
Image code128Image = code128.createImageWithBarcode(cb, null, null);
code128Image.setAbsolutePosition(10, 700);
code128Image.scalePercent(150);
// Add image to pdf file
doc.add(code128Image);
// Type of barcode
BarcodeEAN codeEAN = new BarcodeEAN();
// Remove all leading and trail space and pass to set code
codeEAN.setCode(myString.trim());
// Set barcode type
codeEAN.setCodeType(BarcodeEAN.EAN13);
/* Create an image of the barcode with a null bar color and
null text color its position and scale percent */
Image codeEANImage = code128.createImageWithBarcode(cb, null, null);
codeEANImage.setAbsolutePosition(10, 600);
codeEANImage.scalePercent(150);
// Add image to pdf file
doc.add(codeEANImage);
System.out.println("Barcode in PDF file is created...");
} catch (Exception ex) {
ex.printStackTrace();
} finally {
// close doc and docwriter if they are not null
if (doc != null) {
doc.close();
}
if (pdfWriter != null) {
pdfWriter.close();
}
}
}
}
Output:
Conclusion
Barcodes inscribe product data into bars and alphanumerical characters, making it much quicker and easier to enter things at a store or track inventory in an exceeding warehouse. Besides ease and speed, barcodes’ prominent business edges embrace accuracy, internal control, and price savings—their square measure many barcodes.
There are many formats of barcodes. We use different formats in different situations.
We can use all the barcode formats with the help of the zxing
and itextpdf
libraries. There are other libraries, but these two are easy and fast.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn